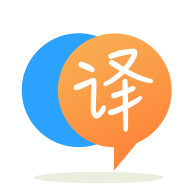
[英]Disable RecyclerView scrolling, so scrollview CHILD can scroll
[英]Disable Scrolling in child Recyclerview android
我的布局包含一个带有子Recyclerview的Parent RecyclerView
我知道将列表放在另一个列表中是不好的但我必须这样我才能使用子列表功能,如滑动和拖放
我的问题是,孩子Recyclerview获得焦点并停止父母滚动如果触摸点在它上面只是我想如果触摸垂直在孩子上Recyclerview父母上下滚动,如果触摸是水平或点击然后子Recyclerview列表项左右滑动。 有没有帮助实现这一目标?
我终于找到了解决方案。
创建自定义LinearLayoutManager
public class CustomLinearLayoutManager extends LinearLayoutManager {
public CustomLinearLayoutManager(Context context, int orientation, boolean reverseLayout) {
super(context, orientation, reverseLayout);
}
// it will always pass false to RecyclerView when calling "canScrollVertically()" method.
@Override
public boolean canScrollVertically() {
return false;
}
}
然后像这样实例化它以进行垂直滚动
CustomLinearLayoutManager customLayoutManager = new CustomLinearLayoutManager(getActivity(),LinearLayoutManager.VERTICAL,false);
最后将自定义布局设置为回收器视图的布局管理器
recyclerView.setLayoutManager(customLayoutManager);
虽然嵌入回收站视图可能不是一个好习惯,但有时您无法避免。 像这样的东西可能会起作用:
public class NoScrollRecycler extends RecyclerView {
public NoScrollRecycler(Context context){
super(context);
}
public NoScrollRecycler(Context context, AttributeSet attrs){
super(context, attrs);
}
public NoScrollRecycler(Context context, AttributeSet attrs, int style){
super(context, attrs, style);
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev){
//Ignore scroll events.
if(ev.getAction() == MotionEvent.ACTION_MOVE)
return true;
//Dispatch event for non-scroll actions, namely clicks!
return super.dispatchTouchEvent(ev);
}
}
这将禁用滚动事件,但不会禁用单击事件。 将此类用于“child”RecyclerView。 您希望PARENT recyclerview能够滚动,而不是孩子。 那么这应该这样做,因为父母将只是标准的RecyclerView,但孩子将是这个自定义的没有滚动,但处理点击。 可能需要禁用单击父级RecyclerView ..不确定,因为我没有测试过这个,所以请考虑它只是一个例子......
另外,要在XML中使用它(如果您不知道),请执行以下操作:
<com.yourpackage.location.NoScrollRecycler
...
... >
...
...
</com.yourpackage.location.NoScrollRecycler>
在您的ActivityName.java上,在onCreate()方法内写入:
RecyclerView v = (RecyclerView) findViewById(R.id.your_recycler_view_id);
v.setNestedScrollingEnabled(false);
无论如何,如果您正在使用协调器布局,如果您想简化操作,并且您想要禁用嵌套滚动。
<android.support.v4.widget.NestedScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<android.support.v7.widget.RecyclerView
android:id="@+id/activitiesListRV"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</android.support.v4.widget.NestedScrollView>
再次,您应用相同的原则:在您的ActivityName.java上,在onCreate()方法内写:
RecyclerView v = (RecyclerView) findViewById(R.id.your_recycler_view_id);
v.setNestedScrollingEnabled(false);
所以基本上在XML中,你必须指定app:layout_behavior
app:layout_behavior="@string/appbar_scrolling_view_behavior">
子级RecyclerView中的android:nestedScrollingEnabled="false"
你可以加
android:nestedScrollingEnabled="false"
用XML或XML格式的RecyclerView
childRecyclerView.setNestedScrollingEnabled(false);
到您的RecyclerView in Java。
编辑:-
childRecyclerView.setNestedScrollingEnabled(false);
将仅适用于android_version> 21台设备。 要在所有设备上工作,请使用以下命令
ViewCompat.setNestedScrollingEnabled(childRecyclerView, false);
你可以使用setNestedScrollingEnabled(false);
在子RecyclerView
上停止在子RecyclerView
内滚动。
在我的情况下代码是
mInnerRecyclerView.setNestedScrollingEnabled(false);
其中mInnerRecyclerView
是内部RecyclerView
。
我尝试了许多建议的解决方案,找不到一个适用于我的情况。 我使用GridLayoutManager在ScrollView中有超过1个RecyclerView。 上面建议的结果导致ScrollView在我抬起手指时停止滚动(当我的手指被提升到RecyclerView上时,它没有滑到视图的顶部或底部)
查看RecyclerView源代码,在onTouchEvent内部调用布局管理器:
final boolean canScrollHorizontally = mLayout.canScrollHorizontally();
final boolean canScrollVertically = mLayout.canScrollVertically();
如果在自定义布局管理器中覆盖这些,则返回false并停止滚动。 它还解决了ScrollView突然停止滚动的问题。
我想我已经太迟了但是在这里我找到了解决方案,如果它还在讨厌某人:
RecyclerView v = (RecyclerView);
findViewById(R.id.your_recycler_view_id);
v.setNestedScrollingEnabled(false);
sensorsRecyclerView.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
return true;
}
});
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.RecyclerView
android:id="@+id/rv"
android:layout_marginTop="2dp"
android:layout_marginLeft="2dp"
android:layout_marginBottom="10dp"
android:layout_marginRight="2dp"
android:nestedScrollingEnabled="false"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
</RelativeLayout>
把代码放在linner布局中......不需要务实地做任何事情
如果您不想创建自定义视图,另一个选项是在RecyclerView前面创建相同大小的布局,并使其可单击。
编辑:但不幸的是它也阻止了列表项的事件。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.