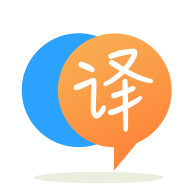
[英]MySQL + VB.NET - Populate multiple Datagridviews from multiple tables
[英]Formating excel spread sheet export from multiple datagridviews vb.net
我目前正在使用带有SQL后端的Microsoft Visual Studio 2013。 我正在加载多个DGV,然后尝试将其导出到Excel电子表格。 但是,我不确定如何格式化此数据。 当我尝试导出多个DGV时,它们会彼此覆盖在excel文件的左上角。 有谁知道如何格式化和选择要在excel电子表格上加载DGV的位置。 我也不希望它们都放在不同的纸上。 这是我的代码:
Private Sub BtnExcelExport_Click(sender As Object, e As EventArgs) Handles BtnExcelExport.Click
Try
Dim xlapp As Microsoft.Office.Interop.Excel.Application
Dim xlworkbook As Microsoft.Office.Interop.Excel.Workbook
Dim xlworksheet As Microsoft.Office.Interop.Excel.Worksheet
Dim misvalue As Object = System.Reflection.Missing.Value
Dim I As Integer
Dim J As Integer
xlapp = New Microsoft.Office.Interop.Excel.Application
xlworkbook = xlapp.Workbooks.Add(misvalue)
xlworksheet = xlworkbook.Sheets("sheet1")
For I = 0 To DGVJobs.RowCount - 1
For J = 0 To DGVJobs.ColumnCount - 1
For K As Integer = 1 To DGVJobs.Columns.Count
xlworksheet.Cells(1, K) = DGVJobs.Columns(K - 1).HeaderText
xlworksheet.Cells(I + 2, J + 1) = DGVJobs(J, I).Value.ToString()
Next
Next
Next
'Here is my second DGV upload out of a few, this one will write over top of the other
For I = 0 To DGVAssociates.RowCount - 1
For J = 0 To DGVAssociates.ColumnCount - 1
For K As Integer = 1 To DGVAssociates.Columns.Count
xlworksheet.Cells(1, K) = DGVAssociates.Columns(K - 1).HeaderText
xlworksheet.Cells(I + 2, J + 1) = DGVAssociates(J, I).Value.ToString()
Next
Next
Next
xlworksheet.SaveAs("C:\example.xlsx")
xlworkbook.Close()
xlapp.Quit()
releaseobject(xlapp)
releaseobject(xlworkbook)
releaseobject(xlworksheet)
MsgBox("You can find the file C:\example.xlsx")
Dim res As MsgBoxResult
res = MsgBox("Saving completed, would you like to open the file?", MsgBoxStyle.YesNo)
If (res = MsgBoxResult.Yes) Then
Process.Start("C:\example.xlsx")
End If
Catch ex As Exception
MsgBox(ex.ToString)
End Try
End Sub
Private Sub releaseobject(ByVal obj As Object)
Try
System.Runtime.InteropServices.Marshal.ReleaseComObject(obj)
obj = Nothing
Catch ex As Exception
obj = Nothing
Finally
GC.Collect()
End Try
End Sub
第二个for循环是我遇到覆盖问题的地方。 如果任何人都有可以将我发送到良好网站的链接,请也将其发布。
为什么您要遍历两次列? 最内层的循环是不必要的。
现在,用于指定要在excel中写入的列的变量在For
的两组中都从0变为ColumnCount
。 这就是为什么他们互相覆盖的原因。
尝试这个:
For I = 0 To DGVJobs.RowCount - 1
For J = 0 To DGVJobs.ColumnCount - 1
xlworksheet.Cells(1, J + 1) = DGVJobs.Columns(J).HeaderText
xlworksheet.Cells(I + 2, J + 1) = DGVJobs(J, I).Value.ToString()
Next
Next
For I = 0 To DGVAssociates.RowCount - 1
For J = 0 To DGVAssociates.ColumnCount - 1
xlworksheet.Cells(1, J + 1 + DGVJobs.ColumnCount) = DGVAssociates.Columns(K - 1).HeaderText
xlworksheet.Cells(I + 2, J + 1 + DGVJobs.ColumnCount) = DGVAssociates(J, I).Value.ToString()
Next
Next
(顺便说一句,离题;将数据导出到excel的更快方法是先创建一个包含所有数据的数组,然后将其分配到工作表中的相应Range
)
尝试使用此代码,那么您肯定会给我发一条感谢消息:)
Imports Excel = Microsoft.Office.Interop.Excel
Imports System.IO
Private Sub ExportToExcel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ExportToExcel.Click
Try
Dim xlapp As Microsoft.Office.Interop.Excel.Application
Dim xlworkbook As Microsoft.Office.Interop.Excel.Workbook
Dim xlworksheet, xlworksheet1 As Microsoft.Office.Interop.Excel.Worksheet
Dim misvalue As Object = System.Reflection.Missing.Value
Dim I As Integer
Dim J As Integer
xlapp = New Microsoft.Office.Interop.Excel.Application
xlworkbook = xlapp.Workbooks.Add(misvalue)
xlworksheet = xlworkbook.Sheets("Sheet1")
For I = 0 To DataGridView_Report.RowCount - 1
For J = 0 To DataGridView_Report.ColumnCount - 1
For K As Integer = 1 To DataGridView_Report.Columns.Count
xlworksheet.Cells(1, K) = DataGridView_Report.Columns(K - 1).HeaderText
xlworksheet.Cells(I + 2, J + 1) = DataGridView_Report(J, I).Value.ToString()
Next
Next
Next
xlworksheet1 = CType(xlworkbook.Worksheets.Add(), Excel.Worksheet)
For I = 0 To DataGridView_Calculation.RowCount - 1
For J = 0 To DataGridView_Calculation.ColumnCount - 1
For K As Integer = 1 To DataGridView_Calculation.Columns.Count
xlworksheet1.Cells(1, K) = DataGridView_Calculation.Columns(K - 1).HeaderText
xlworksheet1.Cells(I + 2, J + 1) = DataGridView_Calculation(J, I).Value.ToString()
Next
Next
Next
xlworksheet.Columns.AutoFit()
xlworksheet1.Columns.AutoFit()
Dim xlRange, xlRange1 As Excel.Range
xlRange = xlworksheet.Range("A1", "N1")
xlRange1 = xlworksheet1.Range("A1", "L1")
xlRange.Columns.Font.Bold = True
xlRange1.Columns.Font.Bold = True
xlworksheet.Name = "My Sheet1"
xlworksheet1.Name = "My Sheet2"
Dim saveFileDialog1 As New SaveFileDialog()
saveFileDialog1.Filter = "Excel Workbook|*.xls|Excel Workbook 2011|*.xlsx"
saveFileDialog1.Title = "Save Excel File"
saveFileDialog1.FileName = "Export " & Now.ToShortDateString & ".xlsx"
saveFileDialog1.ShowDialog()
saveFileDialog1.InitialDirectory = "C:/"
If saveFileDialog1.FileName <> "" Then
Dim fs As System.IO.FileStream = CType(saveFileDialog1.OpenFile(), System.IO.FileStream)
fs.Close()
End If
Dim strFileName As String = saveFileDialog1.FileName
Dim blnFileOpen As Boolean = False
Try
Dim fileTemp As System.IO.FileStream = System.IO.File.OpenWrite(strFileName)
fileTemp.Close()
Catch ex As Exception
blnFileOpen = False
Exit Sub
End Try
If System.IO.File.Exists(strFileName) Then
System.IO.File.Delete(strFileName)
End If
xlworkbook.SaveAs(strFileName)
xlapp.Workbooks.Open(strFileName)
xlapp.Visible = True
Exit Sub
errorhandler:
MsgBox(Err.Description)
Catch ex As Exception
MsgBox(ex.ToString)
End Try
End Sub
Private Sub releaseobject(ByVal obj As Object)
Try
System.Runtime.InteropServices.Marshal.ReleaseComObject(obj)
obj = Nothing
Catch ex As Exception
obj = Nothing
Finally
GC.Collect()
End Try
End Sub
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.