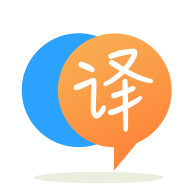
[英]Vec<&str>` cannot be built from an iterator over elements of type ()
[英]Iterator over elements around specific index in Vec<Vec<Object>>
我有一个网格: Vec<Vec<Object>>
和一对x / y索引。 我想找到围绕着一个索引的所有元素。
不幸的是,我不能简单地遍历元素,因为这最终两次借用了Vec
,借阅检查器对我大叫:
let mut cells = Vec::with_capacity(8);
for cx in xstart..xend {
for cy in ystart..yend {
if cx != x || cy != y {
cells.push(&mut squares[cy as usize][cx as usize]);
}
}
}
cells.into_iter()
我将其更改为迭代器链的最佳尝试也失败了:
let xstart = if x == 0 { x } else { x - 1 };
let xlen = if x + 2 > squares[0].len() { x + 1 } else { 3 };
let ystart = if y == 0 { y } else { y - 1 };
let ylen = if y + 2 > squares.len() { y + 1 } else { 3 };
let xrel = x - xstart;
let yrel = y - ystart;
squares.iter().enumerate()
.skip(ystart).take(ylen).flat_map(|(i, ref row)|
row.iter().enumerate()
.skip(xstart).take(xlen).filter(|&(j, &c)| i != yrel || j != xrel))
有人知道我该怎么做吗?
就个人而言,当元素的相对位置很重要时,我不确定是否会喜欢使用迭代器。 相反,我将寻求为这些元素创建一个“视图”。
要点可以在这里找到 ,但是想法很简单,所以这里是核心结构。
#[derive(Debug)]
struct NeighbourhoodRow<'a, T>
where T: 'a
{
pub left : Option<&'a mut T>,
pub center : Option<&'a mut T>,
pub right : Option<&'a mut T>,
}
#[derive(Debug)]
struct Neighbourhood<'a, T>
where T: 'a
{
pub top : NeighbourhoodRow<'a, T>,
pub center : NeighbourhoodRow<'a, T>,
pub bottom : NeighbourhoodRow<'a, T>,
}
为了构建它们,我使用了健康的split_at_mut
剂量:
fn take_centered_trio<'a, T>(row: &'a mut [T], x: usize) ->
(Option<&'a mut T>, Option<&'a mut T>, Option<&'a mut T>)
{
fn extract<'a, T>(row: &'a mut [T], x: usize) -> (Option<&'a mut T>, &'a mut [T]) {
if x+1 > row.len() {
(None, row)
} else {
let (h, t) = row.split_at_mut(x+1);
(Some(&mut h[x]), t)
}
}
let (prev, row) = if x > 0 { extract(row, x-1) } else { (None, row) };
let (elem, row) = extract(row, 0);
let (next, _ ) = extract(row, 0);
(prev, elem, next)
}
其余只是一些有趣的构造函数。
当然,您可以在这些基础上构建某种迭代器。
您想获得对所有周围元素的可变引用,对吗? 我认为这不可能直接做到。 问题是,Rust无法静态证明您想要可变引用不同的单元格。 例如,如果它忽略了这一点,那么您可能会在建立索引时犯一个小错误,并获得对同一数据的两个可变引用,这是Rust保证避免的事情。 因此,它不允许这样做。
在语言级别,这是由IndexMut
特性引起的。 您可以看到其唯一方法的self
参数寿命如何与结果寿命相关联:
fn index_mut(&'a mut self, index: Idx) -> &'a mut Self::Output;
这意味着,如果调用此方法(隐式地通过索引操作),则整个对象将被可变借用,直到结果引用超出范围。 这样可以防止多次调用&mut a[i]
。
解决此问题的最简单,最安全的方法是以“双缓冲”方式重构代码-您有两个字段实例,并且在每个步骤之间互相复制数据。 或者,您可以在每个步骤上创建一个临时字段,并在所有计算后用它替换主要字段,但它可能比交换两个字段效率低。
解决此问题的另一种方法自然是使用原始的*mut
指针。 这是unsafe
,只能直接用作最后的手段。 您可以使用不安全性来实现安全的抽象,例如
fn index_multiple_mut<'a, T>(input: &'a mut [Vec<T>], indices: &[(usize, usize)]) -> Vec<&'a mut T>
在这里,您首先要检查所有索引是否都不同,然后对某些指针强制转换使用unsafe
(可能使用transmute
)来创建结果向量。
第三种可能的方法是以某种巧妙的方式使用split_at_mut()
方法,但我不确定这种方法是否可行,如果可以的话,可能不太方便。
最后,在#rust
的帮助下,我制作了一个自定义迭代器
我已经type
d的结构以提供实际的代码。 正如#rust
的人指出的那样,您必须从迭代器中安全地返回&mut
,而不必使用任何使用unsafe
迭代器,并且鉴于此处的数学非常简单,可以确保它不会出错,因此不安全是解决问题的方法走。
type FieldSquare = u8;
use std::iter::Iterator;
pub struct SurroundingSquaresIter<'a> {
squares: &'a mut Vec<Vec<FieldSquare>>,
center_x: usize,
center_y: usize,
current_x: usize,
current_y: usize,
}
pub trait HasSurroundedSquares<'a> {
fn surrounding_squares(&'a mut self, x: usize, y:usize) -> SurroundingSquaresIter<'a>;
}
impl<'a> HasSurroundedSquares<'a> for Vec<Vec<FieldSquare>> {
fn surrounding_squares(&'a mut self, x: usize, y:usize) -> SurroundingSquaresIter<'a> {
SurroundingSquaresIter {
squares: self,
center_x: x,
center_y: y,
current_x: if x == 0 { x } else { x - 1 },
current_y: if y == 0 { y } else { y - 1 },
}
}
}
impl<'a> Iterator for SurroundingSquaresIter<'a> {
type Item = &'a mut FieldSquare;
fn next(&mut self) -> Option<&'a mut FieldSquare> {
if self.current_y + 1 > self.squares.len() || self.current_y > self.center_y + 1 {
return None;
}
let ret_x = self.current_x;
let ret_y = self.current_y;
if self.current_x < self.center_x + 1 && self.current_x + 1 < self.squares[self.current_y].len() {
self.current_x += 1;
}
else {
self.current_x = if self.center_x == 0 { self.center_x } else { self.center_x - 1 };
self.current_y += 1;
}
if ret_x == self.center_x && ret_y == self.center_y {
return self.next();
}
Some(unsafe { &mut *(&mut self.squares[ret_y][ret_x] as *mut _) })
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.