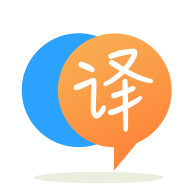
[英]How to define a custom key equivalence predicate for an std::unordered_set?
[英]Undefined behavior in std::unordered_set with custom predicate
我有一个std::unordered_set
,它应该存储指向存储在std::list
中的值的指针。 这些值首先添加到列表中,然后将其指针插入到集合中。 该集合使用谓词来比较指针指向的值而不是地址。 这会产生不确定的行为。
这是一个最小的工作示例:
#include <unordered_set>
#include <iostream>
#include <string>
#include <list>
using namespace std;
template<typename T> struct set_hash {
size_t operator()(const T* p) const noexcept {
return reinterpret_cast<uintptr_t>(p);
}
};
template<typename T> struct set_eq {
bool operator()(const T* a, const T* b) const noexcept {
std::cout << "*a["<<*a<<"] == *b["<<*b<<"] "
<< boolalpha << (*a == *b) << std::endl;
return *a == *b;
}
};
template<typename T> using set_t =
std::unordered_set<const T*, set_hash<T>, set_eq<T>>;
int main()
{
set_t<string> set;
list<string> list{"a", "b", "a", "c", "a", "d"};
for (auto& str : list) {
set.insert(&str);
cout << str << ' ';
}
cout << endl;
for (auto p : set) cout << *p << ' ';
cout << endl;
string c("c");
cout << **set.find(&c) << endl;
return 0;
}
多次运行该程序后,我得到三个可能的输出:
a b a c a d
d a c a b a
Segmentation fault
a b a c a d
d a c a b a
*a[c] == *b[a] false
Segmentation fault
a b a c a d
d a c a b a
*a[c] == *b[c] true
c
我期望的输出是
a b a c a d
a b c (not necessarily in this order)
c
带有*a[c] == *b[c] true
,具体取决于谓词被调用的次数。
我不明白是什么导致了不确定的行为。 我用gcc4.8.2,gcc4.9.1和gcc4.9.2得到了相同的结果。
问题在于散列函数对指针进行哈希处理,而比较函数对指针所指向的值进行比较。 因此,如果您有两个具有相同值的不同列表元素,则哈希函数将给出不同的哈希值(来自指针),而比较函数将比较相等。
哈希函数必须保持一致-它需要哈希值而不是指针。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.