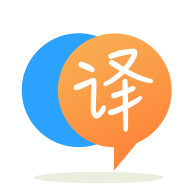
[英]How can I send the selected information in an html page(JSP) to a servlet?
[英]How can I make an HTML page (not JSP) communicate with a Servlet?
使用以下servlet代码,
package com.example.tutorial;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletExample extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
if(request.getParameter("firstname") == null || request.getParameter("lastname") == null){
this.getServletContext().getRequestDispatcher("/index.jsp").forward(request, response);
return;
}
this.getServletContext().getRequestDispatcher("/output.jsp").forward(request, response);
}
}
我首先使用index.jsp
接收请求参数,如下所示:
<?xml version="1.0" encoding="ISO-8859-1" ?>
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1" />
<title>Insert title here</title>
</head>
<body>
<form action="servletexample" method="post" >
<table border="0">
<tr>
<td>First Name:</td> <td><input type="text" name="firstname" /></td>
</tr>
<tr>
<td>Last Name:</td> <td><input type="text" name="lastname" /></td>
</tr>
<tr>
<td colspan="2"> <input type="submit" value="Submit" /></td>
</tr>
</table>
</form>
</body>
</html>
然后servlet使用requestdispatcher
与output.jsp
通信,如下所示:
<?xml version="1.0" encoding="ISO-8859-1" ?>
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1" />
<title>Insert title here</title>
</head>
<body>
<h1>Your first and last name is: </h1>
<%
String firstName = request.getParameter("firstname");
String lastName = request.getParameter("lastname");
out.print(firstName + " " + lastName);
%>
</body>
</html>
在上面的output.jsp
代码中, request.getParameter
方法用于从servlet检索转发的参数。
现在,我不想使用output.jsp
,而只想使用html文件[带javascript(如果需要)],
html正文的内容应该是什么?
注意:更进一步,意图是仅使用html / javascrip / css作为前端,并使用基于Java的Web框架(如Spring)作为后端。 我目前对servlets / jsp的学习是平稳过渡到可以与html / javascrip / css一起使用的Spring框架(后端)
根据您的评论:
我想要与服务器通信并更改显示的Javascript操作。 基本上我想将html / JavaSript / css视为前端,将servlet(仅)视为后端
然后最好改用ajax请求,并使用JSON编写响应而不是转发。 然后,您的前端可能是纯HTML或其他技术(例如移动应用程序)。
请注意,如果使用Servlet的唯一目的是创建RESTful服务,那么最好使用REST方法,而不是自己编写Servlet。 例如,您有两个选择:
如果您完全不熟悉Java,我建议您使用JAX-RS。 如果您已经对Java和Spring有一定的经验,那么我建议您使用Spring,可以选择使用Spring Boot,这取决于您的具体情况。
首先Java是基于编译器的语言,而html是基于解释器的语言。所以您不能在html文件中编写Java代码。它可以用jsp编写。 如果要使用html而不用编写Java代码,则可以。
使用此代码创建html文件output.html。
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
<h1>Your first and last name is: </h1>
</body>
</html>
在此之后,只需将RequestDispatcher forward语句替换为此
this.getServletContext().getRequestDispatcher("/output.html").forward(request, response);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.