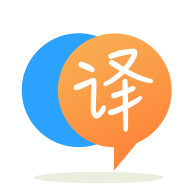
[英]How can I merge 2 javascript objects, populating the properties in one if they don't exist in the other?
[英]In Javascript how do I automatically set properties to null if they don't exist?
我有一个Javascript类(使用John Resig的方法 ),它创建的实例并传递args
对象,如下所示:
var myCucumber = new Cucumber({
size: 'small'
organic: true
})
在类本身内,它引用args
对象上的许多属性。 但是,这些属性都不是必需的,因此有时可能会缺少一些属性,从而导致“属性未定义”错误。
为了解决这个问题,我执行以下操作:
args.size = args.size || null;
args.organic = args.organic || false;
args.colour = args.colour || null;
args.origin = args.origin || null;
必须为整个类中可能使用的每个属性执行此操作似乎很烦人。
是否有一种干净的方法可以假设,如果在创建类的实例时未传递args的任何属性,则该属性为null
?
我建议添加一个函数以预期的方式处理值。
例:
Cucumber.prototype._args = function(attr) {
return this.args[attr] || null;
}
// Then you may use it to access values as follows:
this._args('size');
尝试这样的事情:
for (var key in args.keys()) {
args[key] = args[key] || null;
}
这是因为每个对象都有一个keys()
函数,该函数返回该对象的键数组。
参考: https : //developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Global_Objects/Object
您有几种方法可以执行此操作,但是我不会使用Resig方法,因为它在ES5中存在问题。 是否已弃用John Resig的Javascript继承代码段?
1)(Resig)创建一个构造函数并将值分配给所有不存在的属性:
var Cucumber = Class.extend({
{
init: function(args){
this.size = null;
this.organic = false;
//etc
for (var key in args.keys()) {
this[key] = args[key];
}
},
}
2)第二个选项使用带描述符的Object.create 。 这使您能够创建具有默认值的对象属性。
// Example where we create an object with a couple of sample properties.
// (Note that the second parameter maps keys to *property descriptors*.)
o = Object.create(Object.prototype, {
// foo is a regular 'value property'
size: { writable: true, configurable: true, value: null },
});
3)相似地使用Object.defineProperty
我更喜欢后两种方式,因为我相信使用Object.create / Object.defineProperty显然更好,这是一些其他信息:
http://jaxenter.com/a-modern-approach-to-object-creation-in-javascript-107304.html
您可以在引用对象之前检查是否已设置任何对象属性,如@adeneo建议。
如果您的对象具有很长的属性列表,则可以使用@ aliasm2k的解决方案。
或者,您可以编写一个对象构造函数并使用它。 例如
function ToothPaste(color = null, flavor = null, amount = null){
this.color = color;
this.flavor = flavor;
this.amount = amount;
}
var myTp = new ToothPaste('white');
alert(myTp.color);
alert(myTp.flavor);
alert(myTp.amount);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.