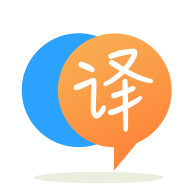
[英]MEF composition using ImportMany and existing instances of composable parts
[英]MEF composition with multiple interdependent parts
设计基于MEF的插件架构且插件之间具有依赖性的最佳方法是什么? 例如:
class MainForm
{
CompositionContainer container;
[ImportMany]
IEnumerable<Lazy<IWindow>> windows;
public MainForm()
{
this.container = new CompositionContainer(new AssemblyCatalog(Assembly.GetExecutingAssembly()));
this.container.ComposeParts(this);
}
public void DoSomething()
{
foreach (var winPart in windows)
{
Debug.WriteLine(winPart.Value.Name);
}
}
}
interface IWindow
{
string Name { get; }
}
delegate void AnEvent(object sender, EventArgs e);
interface IEventManager
{
event AnEvent AnEvent;
void OnAnEvent(object sender, EventArgs eventArgs);
}
[Export(typeof(IEventManager))]
class EventManager : IEventManager
{
public event AnEvent AnEvent = delegate { };
public void OnAnEvent(object sender, EventArgs eventArgs) { AnEvent(sender, eventArgs); }
}
[Export(typeof(IWindow))]
class Window1 : IWindow
{
[Import]
IEventManager eventMgr;
public Window1() { }
public string Name
{
get
{
eventMgr.OnAnEvent(this, new EventArgs());
return "Window1";
}
}
}
[Export(typeof(IWindow))]
class Window2 : IWindow
{
[Import]
IEventManager eventMgr;
public Window2() { this.eventMgr.AnEvent += eventMgr_AnEvent; }
void eventMgr_AnEvent(object sender, EventArgs e)
{
Debug.WriteLine("Event from Window 2");
}
public string Name { get { return "Window2"; } }
}
在Window2
构造函数中, this.eventMgr
为null。 我希望它由MainForm组成。 一种方法是拥有多个CompositionContainers
但如果这样做,则有两个EventManager
实例而不是一个共享实例。 最好的方法是什么?
在进一步探讨这个问题时,相互依赖是完全允许的。 关键是要使目录中可用的装配传递到用于构成零件的CompositionContainer。 然后,以下代码有助于允许一个插件访问另一个插件:
[Export(typeof(IWindow))]
class Window2 : IWindow
{
IEventManager eventMgr;
[ImportingConstructor]
public Window2([Import(typeof(IEventManager))]IEventManager mgr)
{
this.eventMgr.AnEvent += eventMgr_AnEvent;
}
void eventMgr_AnEvent(object sender, EventArgs e)
{
Debug.WriteLine("Event from Window 2");
}
public string Name { get { return "Window2"; } }
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.