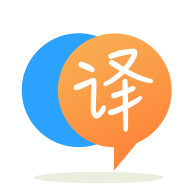
[英]C# code to add soap header wsse:Security, wsse:BinarySecurityToken,ds:Signature, wsse:UsernameToken,wsu:Timestamp
[英]How to use wsse soap service in c#?
我试过使用wsse enable SAOP请求的此代码,但是它不起作用。 我可以帮忙吗?
wsResponseWrapper res = new wsResponseWrapper();
NetworkCredential myCredentials = new NetworkCredential(username, password);
this.srv.Credentials = myCredentials;
this.srv.Timeout = 100000;
res = this.srv.getPlacesList(req);
这是我的app.config
<PullAPSRTC.Properties.Settings>
<setting name="PullAPSRTC_APSRTCService_BookingWSService" serializeAs="String">
<value>http://182.72.148.98:9091/apsrtc-oprs/ws/api/booking</value>
</setting>
</PullAPSRTC.Properties.Settings>
我曾经使用过SOAP,但这是我第一次提高安全性。
将标头添加到请求中,如下所示:
string tokennamespace = "o";
DateTime created = DateTime.UtcNow;
string createdStr = created.ToString("yyyy-MM-ddTHH:mm:ss.fffZ");
string phrase = Guid.NewGuid().ToString();
var nonce = Convert.ToBase64String(Encoding.UTF8.GetBytes(GetSHA1String(phrase)));
this.srv.Headers.Add(string.Format(
"<{0}:UsernameToken u:Id=\"" + Guid.NewGuid() +
"\" xmlns:u=\"http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd\">" +
"<{0}:Username>" + myCredentials.UserName + "</{0}:Username>" +
"<{0}:Password Type=\"http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-username-token-profile-1.0#PasswordText\">" +
myCredentials.Password + "</{0}:Password>" +
"<{0}:Nonce EncodingType=\"http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-soap-message-security-1.0#Base64Binary\">" +
nonce + "</{0}:Nonce>" +
"<u:Created>" + createdStr + "</u:Created></{0}:UsernameToken>", tokennamespace));
这是GetSHA1String方法代码:
public string GetSHA1String(string phrase)
{
SHA1CryptoServiceProvider sha1Hasher = new SHA1CryptoServiceProvider();
byte[] hashedDataBytes = sha1Hasher.ComputeHash(Encoding.UTF8.GetBytes(phrase));
return Convert.ToBase64String(hashedDataBytes);
}
我们将此用于WCF,以下是一些参考,以防您需要自定义请求:
http://www.codeproject.com/Articles/19339/WSSE-Authentication-for-WebRequest-Response
http://weblog.west-wind.com/posts/2012/Nov/24/WCF-WSSecurity-and-WSE-Nonce-Authentication
这是this.srv的声明和定义。
[System.Diagnostics.DebuggerStepThroughAttribute()] [System.CodeDom.Compiler.GeneratedCodeAttribute("System.ServiceModel", "3.0.0.0")] public partial class BookingWSClient : System.ServiceModel.ClientBase<PullAPSRTC.APSRTCService.BookingWS>, PullAPSRTC.APSRTCService.BookingWS { public BookingWSClient() { } public BookingWSClient(string endpointConfigurationName) : base(endpointConfigurationName) { } public BookingWSClient(string endpointConfigurationName, string remoteAddress) : base(endpointConfigurationName, remoteAddress) { } public BookingWSClient(string endpointConfigurationName, System.ServiceModel.EndpointAddress remoteAddress) : base(endpointConfigurationName, remoteAddress) { } public BookingWSClient(System.ServiceModel.Channels.Binding binding, System.ServiceModel.EndpointAddress remoteAddress) : base(binding, remoteAddress) { } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getConcessionListResponse PullAPSRTC.APSRTCService.BookingWS.getConcessionList(PullAPSRTC.APSRTCService.getConcessionList request) { return base.Channel.getConcessionList(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getConcessionList(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getConcessionList inValue = new PullAPSRTC.APSRTCService.getConcessionList(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getConcessionListResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getConcessionList(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getBookingDetailsResponse PullAPSRTC.APSRTCService.BookingWS.getBookingDetails(PullAPSRTC.APSRTCService.getBookingDetails request) { return base.Channel.getBookingDetails(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getBookingDetails(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getBookingDetails inValue = new PullAPSRTC.APSRTCService.getBookingDetails(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getBookingDetailsResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getBookingDetails(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getServiceTypesListResponse PullAPSRTC.APSRTCService.BookingWS.getServiceTypesList(PullAPSRTC.APSRTCService.getServiceTypesList request) { return base.Channel.getServiceTypesList(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getServiceTypesList(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getServiceTypesList inValue = new PullAPSRTC.APSRTCService.getServiceTypesList(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getServiceTypesListResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getServiceTypesList(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getCancellationInfoResponse PullAPSRTC.APSRTCService.BookingWS.getCancellationInfo(PullAPSRTC.APSRTCService.getCancellationInfo request) { return base.Channel.getCancellationInfo(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getCancellationInfo(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getCancellationInfo inValue = new PullAPSRTC.APSRTCService.getCancellationInfo(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getCancellationInfoResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getCancellationInfo(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getAvailableBalanceResponse PullAPSRTC.APSRTCService.BookingWS.getAvailableBalance(PullAPSRTC.APSRTCService.getAvailableBalance request) { return base.Channel.getAvailableBalance(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getAvailableBalance(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getAvailableBalance inValue = new PullAPSRTC.APSRTCService.getAvailableBalance(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getAvailableBalanceResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getAvailableBalance(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getAvailableListByServiceIdResponse PullAPSRTC.APSRTCService.BookingWS.getAvailableListByServiceId(PullAPSRTC.APSRTCService.getAvailableListByServiceId request) { return base.Channel.getAvailableListByServiceId(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getAvailableListByServiceId(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getAvailableListByServiceId inValue = new PullAPSRTC.APSRTCService.getAvailableListByServiceId(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getAvailableListByServiceIdResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getAvailableListByServiceId(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.registerB2BAgentsDetailsResponse PullAPSRTC.APSRTCService.BookingWS.registerB2BAgentsDetails(PullAPSRTC.APSRTCService.registerB2BAgentsDetails request) { return base.Channel.registerB2BAgentsDetails(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper registerB2BAgentsDetails(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.registerB2BAgentsDetails inValue = new PullAPSRTC.APSRTCService.registerB2BAgentsDetails(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.registerB2BAgentsDetailsResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).registerB2BAgentsDetails(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getAvailableServiceListResponse PullAPSRTC.APSRTCService.BookingWS.getAvailableServiceList(PullAPSRTC.APSRTCService.getAvailableServiceList request) { return base.Channel.getAvailableServiceList(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getAvailableServiceList(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getAvailableServiceList inValue = new PullAPSRTC.APSRTCService.getAvailableServiceList(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getAvailableServiceListResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getAvailableServiceList(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.saveBookingDetailsResponse PullAPSRTC.APSRTCService.BookingWS.saveBookingDetails(PullAPSRTC.APSRTCService.saveBookingDetails request) { return base.Channel.saveBookingDetails(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper saveBookingDetails(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.saveBookingDetails inValue = new PullAPSRTC.APSRTCService.saveBookingDetails(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.saveBookingDetailsResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).saveBookingDetails(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getServiceLayoutNewResponse PullAPSRTC.APSRTCService.BookingWS.getServiceLayoutNew(PullAPSRTC.APSRTCService.getServiceLayoutNew request) { return base.Channel.getServiceLayoutNew(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getServiceLayoutNew(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getServiceLayoutNew inValue = new PullAPSRTC.APSRTCService.getServiceLayoutNew(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getServiceLayoutNewResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getServiceLayoutNew(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getServiceLayoutResponse PullAPSRTC.APSRTCService.BookingWS.getServiceLayout(PullAPSRTC.APSRTCService.getServiceLayout request) { return base.Channel.getServiceLayout(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getServiceLayout(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getServiceLayout inValue = new PullAPSRTC.APSRTCService.getServiceLayout(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getServiceLayoutResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getServiceLayout(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getPlacesListResponse PullAPSRTC.APSRTCService.BookingWS.getPlacesList(PullAPSRTC.APSRTCService.getPlacesList request) { return base.Channel.getPlacesList(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getPlacesList(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getPlacesList inValue = new PullAPSRTC.APSRTCService.getPlacesList(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getPlacesListResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getPlacesList(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getMealDetailsResponse PullAPSRTC.APSRTCService.BookingWS.getMealDetails(PullAPSRTC.APSRTCService.getMealDetails request) { return base.Channel.getMealDetails(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getMealDetails(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getMealDetails inValue = new PullAPSRTC.APSRTCService.getMealDetails(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getMealDetailsResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getMealDetails(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.getAccommodationDetailsResponse PullAPSRTC.APSRTCService.BookingWS.getAccommodationDetails(PullAPSRTC.APSRTCService.getAccommodationDetails request) { return base.Channel.getAccommodationDetails(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper getAccommodationDetails(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.getAccommodationDetails inValue = new PullAPSRTC.APSRTCService.getAccommodationDetails(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.getAccommodationDetailsResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).getAccommodationDetails(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.confirmBookingDetailsResponse PullAPSRTC.APSRTCService.BookingWS.confirmBookingDetails(PullAPSRTC.APSRTCService.confirmBookingDetails request) { return base.Channel.confirmBookingDetails(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper confirmBookingDetails(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.confirmBookingDetails inValue = new PullAPSRTC.APSRTCService.confirmBookingDetails(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.confirmBookingDetailsResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).confirmBookingDetails(inValue); return retVal.@return; } [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Advanced)] PullAPSRTC.APSRTCService.confirmTicketCancellationResponse PullAPSRTC.APSRTCService.BookingWS.confirmTicketCancellation(PullAPSRTC.APSRTCService.confirmTicketCancellation request) { return base.Channel.confirmTicketCancellation(request); } public PullAPSRTC.APSRTCService.wsResponseWrapper confirmTicketCancellation(PullAPSRTC.APSRTCService.wsRequestWrapper arg0) { PullAPSRTC.APSRTCService.confirmTicketCancellation inValue = new PullAPSRTC.APSRTCService.confirmTicketCancellation(); inValue.arg0 = arg0; PullAPSRTC.APSRTCService.confirmTicketCancellationResponse retVal = ((PullAPSRTC.APSRTCService.BookingWS)(this)).confirmTicketCancellation(inValue); return retVal.@return; } }
和
BookingWSClient srv = new BookingWSClient();
您可以从http://182.72.148.98:9091/apsrtc-oprs/ws/api/booking?wsdl获取完整的参考
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.