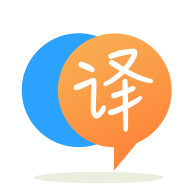
[英]Perl pass hash reference to Subroutine from Foreach loop (Array of Hash)
[英]Hash key value changing to array reference after subroutine in perl
我在同一哈希表的子例程的内部和外部创建密钥。 但是,在子例程之后,我在调用子例程之前创建的键中的值现在被解释为数组引用。
#!/usr/bin/perl
use module;
use strict;
use warnings;
my %hash;
my $count = 0;
my @array = ("a", "b", "c", "d");
for my $letter (@array) {
$hash{$letter} = $count;
$count++;
}
# need "\" to pass in hash otherwise changes
# will get lost outside of subroutine
foreach my $x (sort keys %hash) {
print "first $hash{$x}\n";
}
module::add_ten(\%hash);
foreach my $p (sort keys %hash) {
# $hash{$p} is printing array references, but before it was
# printing the value I desired. What did the subroutine do?
print "second $hash{$p} $hash{$p}->{ten}\n";
}
这是带有子例程的模块
package module;
sub add_ten {
my $count = 10;
# this passes the full array as reference
my ($hash_ref) = @_; # $hash_ref is actually %hash (yes, the % is not a typo)
my @keys = keys $hash_ref;
foreach my $ltr (sort keys $hash_ref) {
$hash_ref->{$ltr} = { ten => $count };
$count++;
}
}
1;
这是输出:
first 0
first 1
first 2
first 3
second HASH(0x7ff0c3049c50) 10
second HASH(0x7ff0c3049bc0) 11
second HASH(0x7ff0c3049b90) 12
second HASH(0x7ff0c3049b60) 13
我期望输出为:
first 0
first 1
first 2
first 3
second 0 10
second 1 11
second 2 12
second 3 13
我修改了我的模块:
package module;
sub add_ten {
my $count = 10;
# this passes the full array as reference
my ($hash_ref) = @_; # $hash_ref is actually %hash (yes, the % is not a typo)
my @keys = keys $hash_ref;
foreach my $ltr (sort keys $hash_ref) {
$hash_ref->{$ltr}{ten}=$count;
$count++;
}
}
1;
和主脚本(需要注释掉使用strict才能使其正常工作):
#!/usr/bin/perl
use module;
#use strict;
use warnings;
my %hash;
my $count = 0;
my @array = ("a", "b", "c", "d");
for my $letter (@array) {
$hash{$letter} = $count;
$count++;
}
# need "\" to pass in hash otherwise changes
# will get lost outside of subroutine
foreach my $x (sort keys %hash) {
print "first $hash{$x}\n";
}
module::add_ten(\%hash);
foreach my $p (sort keys %hash) {
print "second $hash{$p} $hash{$p}{ten}\n";
}
但这就是我想要达到的目标。
$hash_ref
是一个参考%hash
,所以当你通过改变引用的散列的元素的值$hash_ref
,你改变了哈希值%hash
。
那意味着当你做
$hash_ref->{$ltr} = { ten => $count };
你在做
$hash{a} = { ten => 10 };
$hash{a}
不再包含零也就不足为奇了。 您必须更改数据结构。 您可以使用以下内容:
$hash{a}{value} = 0;
$hash{a}{subhash}{ten} = 10;
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.