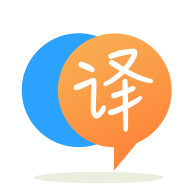
[英]Why are the name repeating in my array, and how would i get a txt file to sort
[英]How do I get my program to recognize and sort the different variable types from a .txt file?
对C ++(和一般编程)来说还算是新手。 我正在尝试写出一个读取以下input.txt的程序:
10
4
6
A 15 6
B 20 10
B 12 8
A 54 12
B 64 9
A 73 30
A 80 10
B 99 15
如图所示,有一个int变量和char(A和B)的组合。 程序使用ifstream识别前三个int(10、4、6)完全正确。 但是,我正在尝试找出一种方法,将剩余的变量分类为特定的数组。
例如,我希望它能够识别出每当有一个“ A”时,该“ A”之后的第一个int进入一个数组,随后的int进入另一个数组。 因此,在这种情况下,我们将拥有数组[15,54,73,80]和[6,12,30,10]。
请注意,未设置input.txt,我需要让它读取具有不同数量的A和B的文件。
有很多方法可以解决此问题,包括编写boost :: spirit语法,但是我不建议C ++新手使用。
相反,我建议使用直接方法,该方法涉及if语句:
- instead of streaming into an int, stream into a string.
- for each string (word), check if word is A or B, if so add to your char container. otherwise call stoi and add to your int container.
在这里,您已经非常快速地编写了示例,因此可以将某些部分做得更好,例如检查字符串是否为数字,或者使用的向量过多等。
#include <vector>
#include <memory>
#include <iostream>
#include <fstream>
#include <sstream>
#include <map>
#include <iterator>
#include <locale>
struct Data
{
std::string key;
std::vector< int > v;
};
bool isNumber( const std::string& s )
{
if( s.empty() )
{
return false;
}
std::locale loc;
std::string::const_iterator it = s.begin();
while( it != s.end() )
{
if( !std::isdigit( *it, loc) )
{
return false;
}
++it;
}
return true;
}
int main(int argc, char *argv[])
{
std::ifstream file;
file.open( "/home/rwadowski/file.txt" );
std::map< std::string, Data > map;
if( file.is_open() )
{
std::string line;
while( std::getline( file, line ) )
{
std::istringstream stream( line );
std::istream_iterator< std::string > it( stream );
std::istream_iterator< std::string > end;
std::vector< std::string > strings;
while( it != end )
{
strings.push_back( *it );
++it;
}
std::string key;
std::vector< int > v;
for( const std::string& s : strings )
{
if( isNumber( s ) )
{
v.push_back( std::stoi( s ) );
}
else
{
key = s;
}
}
Data data = map[ key ];
data.v.insert( data.v.end(), v.begin(), v.end() );
map[ key ] = data;
}
file.close();
}
std::map< std::string, Data >::iterator i = map.begin();
while( i != map.end() )
{
std::cout << "Key[" << i->first << "] = ";
for( int j = 0; j < i->second.v.size(); ++j )
{
std::cout << i->second.v[ j ] << " ";
}
std::cout << std::endl;
++i;
}
return 0;
}
使用此代码读取input.txt
( C++
)
std::ifstream file("input.txt");
if (!file.is_open()) {
cerr << "Error\n";
return 1;
}
int m, n, o, p, q;
char r;
std::vector<int> a, b;
std::vector<char> c;
file >> m >> n >> o;
while (file >> r >> p >> q) {
if (r == 'A') {
a.push_back(p);
b.push_back(q);
c.push_back(r);
}
}
file.close();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.