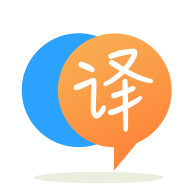
[英]I get Object Reference not set to an instance of an object while invoking SetExpressCheckout method
[英]Object reference not set to an instance of an object when Invoking generic reflective method
因此,这个问题非常令人困惑。 它相当复杂和抽象,所以我将尽力解释它。
我有3类LinkedIn
, Facebook
和Twitter
。 它们全部都继承自一个通用的基类SocialBase
,该基类仅具有一个属性: uuid
并且仅使用此属性,以便我们可以在数据库中找到实际的类型。
因此,我有一个函数可以接受一个Profile
类,该类包含指向所有LinkedIn
, Facebook
和Twitter
表的外键,以及一个Enum值,该值将告诉我们是否要查找LinkedIn
, Facebook
或Twitter
外键
public static async Task UnlinkSocialAccountFromProfile(Profile prof, SocialNetworks provider)
{
//TYPE variable of LinkedIn, Facebook, or Twitter
var handler = HandlerMapping[provider];
//client is MobileServiceClient
var method = client.GetType().GetMethod("GetTable", Type.EmptyTypes);
//MobileServiceClient.GetTable<handler>()
var generic = method.MakeGenericMethod(handler);
//IMobileServiceTable<handler>
var table = generic.Invoke(client, null);
//Profile has 3 foreign keys, LinkedinUUID, FacebookUUID, TwitterUUID, we want the <handler>UUID
string propertyValue = prof.GetType().GetProperty(handler.Name + "UUID").GetValue(prof) as string;
//Invoke Extension method with our generic types
var genMethod =
typeof (Extensions).GetMethod("FilterByNamedProperty")
.MakeGenericMethod(table.GetType().GetGenericArguments()[0], propertyValue.GetType());
//Get the List<handler> that results from our query
var result = await (Task<List<Linkedin>>)(genMethod.Invoke(null, new [] {table, "uuid", propertyValue}));
//var result = await (table as IMobileServiceTable<SocialBase>).FilterByNamedProperty("uuid", propertyValue);
await new SocialResources().DeleteIfExists((result as IList<SocialBase>)[0]);
}
因此,我在这里正在获取类的Type
,因此这将是SocialBase
子类之一。 对于这个特定的例子,我知道我要寻找LinkedIn
类型。 因此,在获得类型LinkedIn
之后,我需要从我的MobileServiceTable
调用通用方法,因此通常看起来像MobileServiceTable.GetTable<LinkedIn>()
但是由于反思,我们不得不采取更长的路径。
得到我返回的IMobileServiceTable<LinkedIn>
实例后,我得到了我要寻找的外键的值。 在这种情况下,它将称为LinkedInUUID
。 现在来了棘手的部分。 我有这个扩展方法,它将为我构造查询表达式,因为它必须是Expression<Func<LinkedIn, bool>>
public async static Task<List<TSource>> FilterByNamedProperty<TSource, TValue>(this IMobileServiceTable<TSource> source, string propertyName, TValue value)
{
// uuid
var property = typeof(TSource).GetProperty(propertyName);
// (TSource)p
var parExp = Expression.Parameter(typeof(TSource));
//p.uuid
var methodExp = Expression.Property(parExp, property);
// value
var constExp = Expression.Constant(value, typeof(TValue));
// p.uuid == value
var binExp = Expression.Equal(methodExp, constExp);
// p => p.uuid == value
var lambda = Expression.Lambda<Func<TSource, bool>>(binExp, parExp);
return await source.Where(lambda).ToListAsync();
}
我相信这些注释在解释每条语句出现时的构建过程方面做得很好。 但是,一旦我们到达return await ...
,应用程序将崩溃。 这是该行之前的输出以及紧随其后的错误。
IMobileServiceTable<TSource> source = {Microsoft.WindowsAzure.MobileServices.MobileServiceTable<SocialConnect.Linkedin>}
(正确)
propertyName = uuid
(正确)
TValue value = dscRJQSIxJaEfd
(正确)
我强烈感觉问题出在我的lambda
表达式上,但是如果我用这一行测试扩展,则var test = await (source as IMobileServiceTable<Linkedin>).Where(p => p.uuid == (value as string)).ToListAsync();
它工作得很好。 但是,一旦我将其更改为使用lambda
变量,我就会得到异常。 lambda表达式的实际值为{Param_0 => (Param_0.uuid == "dscRJQSIxJaEfd")}
,看起来正确
有任何想法吗?
编辑抱歉,这是堆栈跟踪的实际异常
`A first chance exception of type 'System.NullReferenceException' occurred in mscorlib.dll
System.NullReferenceException: Object reference not set to an instance of an object.
at Microsoft.WindowsAzure.MobileServices.Query.FilterBuildingExpressionVisitor.GetTableMemberName(Expression expression, MobileServiceContractResolver contractResolver)
at Microsoft.WindowsAzure.MobileServices.Query.FilterBuildingExpressionVisitor.VisitMemberAccess(MemberExpression expression)
at Microsoft.WindowsAzure.MobileServices.Query.FilterBuildingExpressionVisitor.Visit(Expression node)
at Microsoft.WindowsAzure.MobileServices.Query.FilterBuildingExpressionVisitor.VisitBinary(BinaryExpression expression)
at Microsoft.WindowsAzure.MobileServices.Query.FilterBuildingExpressionVisitor.Visit(Expression node)
at Microsoft.WindowsAzure.MobileServices.Query.FilterBuildingExpressionVisitor.Compile(Expression expression, MobileServiceContractResolver contractResolver)
at Microsoft.WindowsAzure.MobileServices.Query.MobileServiceTableQueryTranslator1.AddFilter(MethodCallExpression expression)
at Microsoft.WindowsAzure.MobileServices.Query.MobileServiceTableQueryTranslator1.VisitMethodCall(MethodCallExpression expression)
at Microsoft.WindowsAzure.MobileServices.Query.MobileServiceTableQueryTranslator1.Visit(Expression expression)
at Microsoft.WindowsAzure.MobileServices.Query.MobileServiceTableQueryTranslator1.Translate()
at Microsoft.WindowsAzure.MobileServices.Query.MobileServiceTableQueryProvider.Compile[T](IMobileServiceTableQuery1 query)
at Microsoft.WindowsAzure.MobileServices.Query.MobileServiceTableQueryProvider.<Execute>d__31.MoveNext()
--- End of stack trace from previous location where exception was thrown ---
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Runtime.CompilerServices.TaskAwaiter1.GetResult()
at Microsoft.WindowsAzure.MobileServices.Query.MobileServiceTableQuery1.<ToListAsync>d__0.MoveNext()
--- End of stack trace from previous location where exception was thrown ---
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Runtime.CompilerServices.TaskAwaiter1.GetResult()
at SocialConnect.Models.Extensions.<FilterByNamedProperty>d__22.MoveNext() in MobileServiceSample\MobileServiceSample\Extensions.cs:line 55`
好吧,我终于解决了这个问题。 显然问题不在于查询或我在做什么,而是与SocialBase
类有关。 我一直在使用它来制作方法,使我知道通用类型TEntity
具有uuid
属性,因此我可以在任何数据库中轻松找到它。 但是我想该字段没有正确序列化,这就是导致NullReferenceException
。 删除基类并反射性地找到属性和值之后,一切开始正常运行。
我不明白为什么它之前无法正常工作,因为在此之前,我已经在很多情况下使用了SocialBase
基类。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.