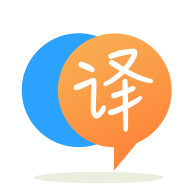
[英]How can I iterate through array of deeply nested objects in javascript?
[英]How can i iterate through nested arrays of objects and remove some attribute
我有一个JavaScript对象数组,其中每个对象都可以有子代,子代的类型与父代的类型相同,子代也可以有多个子代。 我想遍历所有节点并更改一些值。
[
{
"text": "Auto",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true,
"selected": true
}
},
{
"text": "BookMark1",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
},
"children": [
{
"text": "BookMark2",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
}
},
{
"text": "BookMark3",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
}
},
{
"text": "BookMark4",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
}
},
{
"text": "BookMark5",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
}
},
{
"text": "BookMark6",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
},
"children": [
{
"text": "BookMark2",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
}
},
{
"text": "BookMark3",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
}
}
]
},
{
"text": "BookMark7",
"icon": "/libs/jstree/folder.png",
"state": {
"opened": true
}
}
]
}
]
在以上对象中,我要遍历所有节点并删除“ state”属性。 我该如何实现。
尝试这个:
function removeKeys(arr, key) {
arr.forEach(function (element, index) {
delete element[key];
if (element.children !== undefined) removeKeys(element.children, key);
});
}
removeKeys(json, "state");
小提琴: http : //jsfiddle.net/9scd3qb3/
这是递归的方法,它检查内部和外部。
https://jsfiddle.net/8ku7wohd/2/
function removeState(a){
if (a.hasOwnProperty('state')) delete a['state'];
if (a instanceof Array) a.forEach(removeState);
else if (a instanceof Object) for (var k in a) removeState(a[k]);
}
您需要对对象使用递归函数进行迭代。 我在这里为您创建了一个示例和最常见的走槽嵌套对象的方式:
function walk(item){
for(index in item){
console.log(item[index]);
if(typeof item[index] == "object"){
walk(item[index]);
}
}
}
使用此功能作为基础,您可以扩展其功能,并执行您真正需要的一切。 如果使用的是JQuery,则它们将提供功能.each(function(index, item){})
。
如果我有其他帮助,请在此处更新您的帖子或评论。
您可以将JSON.stringify
与replacer
参数一起使用,以这种方式进行操作:
JSON.parse(JSON.stringify(object, ['text', 'icon', 'children']))
该数组给出了要列入结果的“列入白名单”的属性的列表。 所有其他属性(包括state
)都将被忽略/删除。
试试看:
jQuery.each(myJSONObject, function(i, val) {
delete val.state;
});
更新:
另一个需要临时变量的解决方案。
var tempJsonObject = [];
jQuery.each(myJSONObject, function(i, val) {
delete val.state;
tempJsonObject[i] = val;
});
myJSONObject = tempJsonObject;
更新:
包括删除子对象上的state
属性。
removeProperty(myJSONObject, "state");
function removeProperty(myJSONObject, prop) {
jQuery.each(myJSONObject, function(i, val) {
delete val.state;
if(val.hasOwnProperty("children")) {
removeProperty(val.children, "state");
}
});
}
var jsonObj = JSON.parse(text);
for (var i in jsonObj) {
console.log(jsonObj[i].text);
jsonObj[i].text = "some text"
var innerJson = jsonObj[i].children
for (var j in innerJson) {
console.log(innerJson[j].text);
innerJson[j].text = "some other text for all inner"
}
};
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.