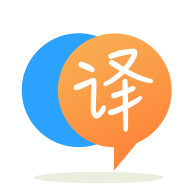
[英]How to embed a link in email message for file attached in email using JavaMail API?
[英]how to send a html email with attached file using JavaMail
以下 Java 代码用于将文件附加到html电子邮件并发送。 我想用这个html电子邮件发送附件。 任何建议,将不胜感激。
public void sendEmail(final String userName, final String password, final String host, final String html, final List<String> emails, String subject, String file) throws MessagingException
{
System.out.println("User Name: " + userName);
System.out.println("Password: " + password);
System.out.println("Host: " + host);
//Get the session object
Properties props = new Properties();
props.put("mail.smtp.host", host);
props.put("mail.smtp.auth", "true");
Session session = Session.getDefaultInstance(props,
new javax.mail.Authenticator()
{
@Override
protected PasswordAuthentication getPasswordAuthentication()
{
return new PasswordAuthentication(userName, password);
}
});
if (!emails.isEmpty())
{
//Compose the message
InternetAddress[] address = new InternetAddress[emails.size()];
for (int i = 0; i < emails.size(); i++)
{
address[i] = new InternetAddress(emails.get(i));
}
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(userName));
message.setRecipients(Message.RecipientType.TO, address);
message.setSubject(subject);
MimeBodyPart messageBodyPart = new MimeBodyPart();
Multipart multipart = new MimeMultipart();
messageBodyPart = new MimeBodyPart();
String fileName = "attachmentName";
DataSource source = new FileDataSource(file);
messageBodyPart.setDataHandler(new DataHandler(source));
messageBodyPart.setFileName(fileName);
multipart.addBodyPart(messageBodyPart);
message.setContent(html, "text/html; charset=utf-8");
message.setContent(multipart);
//send the message
Transport.send(message);
System.out.println("message sent successfully...");
} else
{
System.out.println("No Recieptions");
}
}
这给我带来的只是附件。 但我想用这个附件发送 html 电子邮件。
创建带有 HTML 正文和附件的邮件,实际上意味着创建内容为“多部分实体”的邮件,其中包含两部分,其中一部分是 HTML 内容,第二部分是附件。
这与您当前的代码不符:
Multipart multipart = new MimeMultipart(); // creating a multipart is OK
// Creating the first body part of the multipart, it's OK
messageBodyPart = new MimeBodyPart();
// ... bla bla
// ok, so this body part is the "attachment file"
messageBodyPart.setDataHandler(new DataHandler(source));
// ... bla bla
multipart.addBodyPart(messageBodyPart); // at this point, the multipart contains your file attachment, but only that!
// at this point, you set your mail's body to be the HTML message
message.setContent(html, "text/html; charset=utf-8");
// and then right after that, you **reset** your mail's content to be your multipart, which does not contain the HTML
message.setContent(multipart);
此时,您的电子邮件内容是一个多部分,只有 1 个部分,即您的附件。
因此,要达到您的预期结果,您应该采取不同的方式:
MimeBodyPart
这大致翻译为:
Multipart multipart = new MimeMultipart(); //1
// Create the attachment part
BodyPart attachmentBodyPart = new MimeBodyPart(); //2
attachmentBodyPart.setDataHandler(new DataHandler(fileDataSource)); //2
attachmentBodyPart.setFileName(file.getName()); // 2
multipart.addBodyPart(attachmentBodyPart); //3
// Create the HTML Part
BodyPart htmlBodyPart = new MimeBodyPart(); //4
htmlBodyPart.setContent(htmlMessageAsString , "text/html"); //5
multipart.addBodyPart(htmlBodyPart); // 6
// Set the Multipart's to be the email's content
message.setContent(multipart); //7
**Send Email using html body and file attachement**
> try {
host = localhost;//use your host or pwd if any
Properties props = System.getProperties();
props.put("mail.smtp.host", host);
props.setProperty("mail.smtp.auth", "false");
Session session = Session.getInstance(props, null);
MimeMessage mailer = new MimeMessage(session);
// recipient
mailer.setRecipient(javax.mail.Message.RecipientType.TO, new InternetAddress(toEmail));
// sender
mailer.setFrom(new InternetAddress(fromEmail));
// cc
if (ccEmail != null) {
for (int i = 0; i < ccEmail.size(); i++) {
String cc = ccEmail.get(i);
mailer.addRecipient(javax.mail.Message.RecipientType.CC, new InternetAddress(cc));
}
}
Transport t = session.getTransport("smtp");
t.connect();
mailer.setSubject(subject);
mailer.setFrom(new InternetAddress(fromEmail));
// attachement
Multipart multipart = new MimeMultipart();
BodyPart messageBodyPart = new MimeBodyPart();
BodyPart attachmentBodyPart = new MimeBodyPart();
messageBodyPart.setContent(htmlbody, "text/html"); // 5
multipart.addBodyPart(messageBodyPart);
// file path
File filename = new File(xmlurl);
DataSource source = new FileDataSource(filename);
attachmentBodyPart.setDataHandler(new DataHandler(source));
attachmentBodyPart.setFileName(filename.getName());
multipart.addBodyPart(attachmentBodyPart);
mailer.setContent(multipart);
Transport.send(mailer);
System.out.println("Mail completed");
} catch (SendFailedException e) {//errr
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.