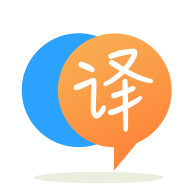
[英]How do I get TypeScript Array.map() to return the same as VanillaJS?
[英]How do I get the right “this” in an Array.map?
我认为有一些应用程序call
或apply
在这里,但我不知道如何实现它。
http://codepen.io/anon/pen/oXmmzo
a = {
foo: 'bar',
things: [1, 2, 3],
showFooForEach: function() {
this.things.map(function(thing) {
console.log(this.foo, thing);
});
}
}
a.showFooForEach();
假设我想map
一个数组,但在函数中,我需要访问foo
所属的this
。 map
的function
创建了一个新的this
上下文,所以我显然需要以某种方式反驳那个上下文,但是如何在仍然可以访问thing
时这样做呢?
刚刚意识到我应该更仔细地阅读Array.map()
的文档。 一个人只需要传递this
值作为map()
的第二个参数
http://codepen.io/anon/pen/VLggpX
a = {
foo: 'bar',
things: [1, 2, 3],
showFooForEach: function() {
this.things.map(function(thing) {
console.log(this.foo, thing);
}, this);
}
}
a.showFooForEach();
此外,理解bind()
, call()
和apply()
工作对于认真的JavaScript开发人员来说是必须的。 这些允许我们跳过愚蠢的任务,如
var self = this;
myItems.map(function(item) {
self.itemArray.push(item);
});
同
myItems.map(function(item) {
this.itemArray.push(item);
}.bind(this));
自2018年起,您可以使用箭头功能:
a = {
foo: 'bar',
things: [1, 2, 3],
showFooForEach: function() {
this.things.map((thing) => {
console.log(this.foo, thing);
});
}
}
a.showFooForEach();
您可以将bind()
用于您的上下文。
a = {
foo: 'bar',
things: [1, 2, 3],
showFooForEach: function() {
this.things.map(function(thing) {
console.log(this.foo, thing);
}.bind(this));
}
}
a.showFooForEach();
那是因为JS词汇范围
来自MDN:
bind()方法创建一个新函数,在调用时,将其this关键字设置为提供的值,并在调用新函数时提供任何前面提供的给定参数序列。
在这里阅读更多内容: https : //developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function/bind
在这里: http : //javascriptissexy.com/javascript-apply-call-and-bind-methods-are-essential-for-javascript-professionals/
另外, map()
确实接受第二个参数为“this”
a = {
foo: 'bar',
things: [1, 2, 3],
showFooForEach: function() {
this.things.map(function(thing) {
console.log(this.foo, thing);
}, this);
}
}
a.showFooForEach();
来自MDN map()
文档:
参数
callback生成新数组元素的函数
thisArg 可选 。 执行回调时要使用的值。
进一步阅读: https : //developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map
简短的建议
PS。:当您想对数组执行某些操作时,通常会调用Array.map,例如为每个项添加10,或者类似的东西......因为Array.map返回一个新数组。 如果您只使用console.log或不会影响阵列本身的东西,您可以使用Array.forEach调用
没有什么复杂的需要! map
需要thisArg
的第二个参数,所以你只需要在每个项目上调用你想要调用的函数:
a = {
foo: 'bar',
things: [1, 2, 3],
showFooForEach: function() {
this.things.map(function(thing) {
console.log(this.foo, thing);
}, this);
}
}
a.showFooForEach();
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map
有三种方式:
一个普通的变量
一个不具有的特殊奇怪this
:
var self = this;
this.things.map(function(thing) {
console.log(self.foo, thing);
});
Function.prototype.bind
this.things.map(function(thing) {
console.log(this.foo, thing);
}.bind(this));
使用Array.prototype.map的第二个参数
(可选)第二个参数是调用内部函数的上下文。
this.things.map(function(thing) {
console.log(this.foo, thing);
}, this);
前两种方式是处理this
情况的通用方法; 第三个特定于map
, filter
, forEach
。
map允许第二个名为“thisArg”的参数,所以你只需使用它:
showFooForEach: function() {
this.things.map(function(thing) {
console.log(this.foo, thing);
},this);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.