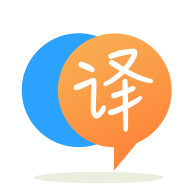
[英]Display Custom Pricing labels on WooCommerce Cart based on user role
[英]Creating Multiple Tiers of Pricing By User Role for WooCommerce/Wordpress
<?php
/** This will be the beginning of the Variation Pricing Functions-------------------------------------------------------------------------------
*/
/** this checks if the current user is capable to have the reseller pricing
*/
function sfprice_administrator_applicable(){
return (bool) ( current_user_can('Administrator') && ( !is_admin() || is_ajax() ) );
}
function sfprice_resellerplus_applicable(){
return (bool) ( current_user_can('resellerplus') && ( !is_admin() || is_ajax() ) );
}
function sfprice_reseller_applicable(){
return (bool) ( current_user_can('reseller') && ( !is_admin() || is_ajax() ) );
}
function sfprice_corporateplus_applicable(){
return (bool) ( current_user_can('corporateplus') && ( !is_admin() || is_ajax() ) );
}
function sfprice_smallbusiness_applicable(){
return (bool) ( current_user_can('smallbusiness') && ( !is_admin() || is_ajax() ) );
}
/** this get the reseller price when available for both Simple & Variable product type
*/
function sfprice_get_administrator_price( $product )
{
if(
$product->is_type( array('simple', 'variable') )
&& get_post_meta( $product->id, '_administrator_price', true ) > 0
){
return get_post_meta( $product->id, '_administrator_price', true );
}
elseif(
$product->is_type('variation')
&& get_post_meta( $product->variation_id, '_administrator_price', true ) > 0
){
return get_post_meta( $product->variation_id, '_administrator_price', true );
}
return 0;
}
function sfprice_get_resellerplus_price( $product )
{
if(
$product->is_type( array('simple', 'variable') )
&& get_post_meta( $product->id, '_resellerplus_price', true ) > 0
){
return get_post_meta( $product->id, '_resellerplus_price', true );
}
elseif(
$product->is_type('variation')
&& get_post_meta( $product->variation_id, '_resellerplus_price', true ) > 0
){
return get_post_meta( $product->variation_id, '_resellerplus_price', true );
}
return 0;
}
function sfprice_get_reseller_price( $product )
{
if(
$product->is_type( array('simple', 'variable') )
&& get_post_meta( $product->id, '_reseller_price', true ) > 0
){
return get_post_meta( $product->id, '_reseller_price', true );
}
elseif(
$product->is_type('variation')
&& get_post_meta( $product->variation_id, '_reseller_price', true ) > 0
){
return get_post_meta( $product->variation_id, '_reseller_price', true );
}
return 0;
}
function sfprice_get_corporateplus_price( $product )
{
if(
$product->is_type( array('simple', 'variable') )
&& get_post_meta( $product->id, '_corporateplus_price', true ) > 0
){
return get_post_meta( $product->id, '_corporateplus_price', true );
}
elseif(
$product->is_type('variation')
&& get_post_meta( $product->variation_id, '_corporateplus_price', true ) > 0
){
return get_post_meta( $product->variation_id, '_corporateplus_price', true );
}
return 0;
}
function sfprice_get_corporate_price( $product )
{
if(
$product->is_type( array('simple', 'variable') )
&& get_post_meta( $product->id, '_corporate_price', true ) > 0
){
return get_post_meta( $product->id, '_corporate_price', true );
}
elseif(
$product->is_type('variation')
&& get_post_meta( $product->variation_id, '_corporate_price', true ) > 0
){
return get_post_meta( $product->variation_id, '_corporate_price', true );
}
return 0;
}
function sfprice_get_smallbusiness_price( $product )
{
if(
$product->is_type( array('simple', 'variable') )
&& get_post_meta( $product->id, '_smallbusiness_price', true ) > 0
){
return get_post_meta( $product->id, '_smallbusiness_price', true );
}
elseif(
$product->is_type('variation')
&& get_post_meta( $product->variation_id, '_smallbusiness_price', true ) > 0
){
return get_post_meta( $product->variation_id, '_smallbusiness_price', true );
}
return 0;
}
/** First, we need to add reseller Pricing input to the product editing page. Here’s the code –
*/
add_action( 'woocommerce_product_options_pricing', 'sfprice_woocommerce_product_options_pricing' );
function sfprice_woocommerce_product_options_pricing()
{
woocommerce_wp_text_input( array(
'id' => '_administrator_price',
'class' => 'wc_input_administrator_price short',
'label' => __( 'Administrator Price', 'woocommerce' ) . ' ('.get_woocommerce_currency_symbol().')',
'type' => 'text'
));
woocommerce_wp_text_input( array(
'id' => '_resellerplus_price',
'class' => 'wc_input_resellerplus_price short',
'label' => __( 'Reseller Plus Price', 'woocommerce' ) . ' ('.get_woocommerce_currency_symbol().')',
'type' => 'text'
));
woocommerce_wp_text_input( array(
'id' => '_reseller_price',
'class' => 'wc_input_reseller_price short',
'label' => __( 'Reseller Price', 'woocommerce' ) . ' ('.get_woocommerce_currency_symbol().')',
'type' => 'text'
));
woocommerce_wp_text_input( array(
'id' => '_corporateplus_price',
'class' => 'wc_input_corporateplus_price short',
'label' => __( 'Corporate Plus Price', 'woocommerce' ) . ' ('.get_woocommerce_currency_symbol().')',
'type' => 'text'
));
woocommerce_wp_text_input( array(
'id' => '_corporate_price',
'class' => 'wc_input_corporate_price short',
'label' => __( 'Corporate Price', 'woocommerce' ) . ' ('.get_woocommerce_currency_symbol().')',
'type' => 'text'
));
woocommerce_wp_text_input( array(
'id' => '_smallbusiness_price',
'class' => 'wc_input_smallbusiness_price short',
'label' => __( 'Small Business Price', 'woocommerce' ) . ' ('.get_woocommerce_currency_symbol().')',
'type' => 'text'
));
}
/** This code will save the entered value for reseller price with the product with a custom meta ‘_reseller_price’.
*/
add_action( 'woocommerce_process_product_meta_simple', 'sfprice_woocommerce_process_product_meta_simple', 10, 1 );
function sfprice_woocommerce_process_product_meta_simple( $product_id )
{
if( isset($_POST['_administrator_price']) && $_POST['_administrator_price'] > 0 )
update_post_meta( $product_id, '_administrator_price', $_POST['_administrator_price'] );
if( isset($_POST['_resellerplus_price']) && $_POST['_resellerplus_price'] > 0 )
update_post_meta( $product_id, '_resellerplus_price', $_POST['_resellerplus_price'] );
if( isset($_POST['_reseller_price']) && $_POST['_reseller_price'] > 0 )
update_post_meta( $product_id, '_reseller_price', $_POST['_reseller_price'] );
if( isset($_POST['_corporateplus_price']) && $_POST['_corporateplus_price'] > 0 )
update_post_meta( $product_id, '_corporateplus_price', $_POST['_corporateplus_price'] );
if( isset($_POST['_corporate_price']) && $_POST['_corporate_price'] > 0 )
update_post_meta( $product_id, '_corporate_price', $_POST['_corporate_price'] );
if( isset($_POST['_smallbusiness_price']) && $_POST['_smallbusiness_price'] > 0 )
update_post_meta( $product_id, '_smallbusiness_price', $_POST['_smallbusiness_price'] );
}
/** Now, to Assign the price for each user type we will need to hook into woocommerce_get_price filter.
*/
add_filter( 'woocommerce_get_price', 'sfprice_woocommerce_get_price', 10, 2);
function sfprice_woocommerce_get_price( $price, $product )
{
if (sfprice_administrator_applicable() && sfprice_get_administrator_price($product) > 0 ) {
$price = sfprice_get_administrator_price($product);
return $price;
} elseif (sfprice_resellerplus_applicable() && sfprice_get_resellerplus_price($product) > 0 ) {
$price = sfprice_get_resellerplus_price($product);
return $price;
} elseif (sfprice_reseller_applicable() && sfprice_get_reseller_price($product) > 0 ) {
$price = sfprice_get_reseller_price($product);
return $price;
} elseif (sfprice_corporateplus_applicable() && sfprice_get_corporateplus_price($product) > 0 ) {
$price = sfprice_get_corporateplus_price($product);
return $price;
} elseif (sfprice_corporate_applicable() && sfprice_get_corporate_price($product) > 0 ) {
$price = sfprice_get_corporate_price($product);
return $price;
} elseif (sfprice_smallbusiness_applicable() && sfprice_get_smallbusiness_price($product) > 0 ) {
$price = sfprice_get_smallbusiness_price($product);
return $price;
}
}
/** The above described method is only for Simple Product. The same feature can be added for Variable Product also, read further below.
*/
add_action( 'woocommerce_product_after_variable_attributes', 'sfprice_woocommerce_product_after_variable_attributes', 10, 3 );
function sfprice_woocommerce_product_after_variable_attributes( $loop, $variation_data, $variation )
{ ?>
<tr class="administrator_price_row">
<td>
<div>
<label><?php _e( 'Administrator Price:', 'woocommerce' ); ?></label>
<input type="text" size="5" name="variable_administrator_price[<?php echo $loop; ?>]" value="<?php if ( isset( $variation_data['_administrator_price'][0] ) ) echo esc_attr( $variation_data['_administrator_price'][0] ); ?>" step="1" min="0" />
</div>
</td>
</tr>
<tr class="resellerplus_price_row">
<td>
<div>
<label><?php _e( 'Reseller Plus Price:', 'woocommerce' ); ?></label>
<input type="text" size="5" name="variable_resellerplus_price[<?php echo $loop; ?>]" value="<?php if ( isset( $variation_data['_resellerplus_price'][0] ) ) echo esc_attr( $variation_data['_resellerplus_price'][0] ); ?>" step="1" min="0" />
</div>
</td>
</tr>
<tr class="reseller_price_row">
<td>
<div>
<label><?php _e( 'Reseller Price:', 'woocommerce' ); ?></label>
<input type="text" size="5" name="variable_reseller_price[<?php echo $loop; ?>]" value="<?php if ( isset( $variation_data['_reseller_price'][0] ) ) echo esc_attr( $variation_data['_reseller_price'][0] ); ?>" step="1" min="0" />
</div>
</td>
</tr>
<tr class="corporateplus_price_row">
<td>
<div>
<label><?php _e( 'Corporate Plus Price:', 'woocommerce' ); ?></label>
<input type="text" size="5" name="variable_corporateplus_price[<?php echo $loop; ?>]" value="<?php if ( isset( $variation_data['_corporateplus_price'][0] ) ) echo esc_attr( $variation_data['_corporateplus_price'][0] ); ?>" step="1" min="0" />
</div>
</td>
</tr>
<tr class="corporate_price_row">
<td>
<div>
<label><?php _e( 'Corporate Price:', 'woocommerce' ); ?></label>
<input type="text" size="5" name="variable_corporate_price[<?php echo $loop; ?>]" value="<?php if ( isset( $variation_data['_corporate_price'][0] ) ) echo esc_attr( $variation_data['_corporate_price'][0] ); ?>" step="1" min="0" />
</div>
</td>
</tr>
<tr class="smallbusiness_price_row">
<td>
<div>
<label><?php _e( 'Small Business Price:', 'woocommerce' ); ?></label>
<input type="text" size="5" name="variable_smallbusiness_price[<?php echo $loop; ?>]" value="<?php if ( isset( $variation_data['_smallbusiness_price'][0] ) ) echo esc_attr( $variation_data['_smallbusiness_price'][0] ); ?>" step="1" min="0" />
</div>
</td>
</tr>
<?php
}
/** Saving the price
*/
add_action( 'woocommerce_save_product_variation', 'sfprice_woocommerce_save_product_variation', 10, 2 );
function sfprice_woocommerce_save_product_variation($variation_id, $i)
{
if( isset($_POST['variable_administrator_price'][$i]) )
update_post_meta( $variation_id, '_administrator_price', $_POST['variable_administrator_price'][$i]);
if( isset($_POST['variable_resellerplus_price'][$i]) )
update_post_meta( $variation_id, '_resellerplus_price', $_POST['variable_resellerplus_price'][$i]);
if( isset($_POST['variable_reseller_price'][$i]) )
update_post_meta( $variation_id, '_reseller_price', $_POST['variable_reseller_price'][$i]);
if( isset($_POST['variable_corporateplus_price'][$i]) )
update_post_meta( $variation_id, '_corporateplus_price', $_POST['variable_corporateplus_price'][$i]);
if( isset($_POST['variable_corporate_price'][$i]) )
update_post_meta( $variation_id, '_corporate_price', $_POST['variable_corporate_price'][$i]);
if( isset($_POST['variable_smallbusiness_price'][$i]) )
update_post_meta( $variation_id, '_smallbusiness_price', $_POST['variable_smallbusiness_price'][$i]);
}
上面是为WooCommerce / Wordpress的6层定价系统创建的代码。 当仅创建了一个新的带有角色关联的定价层时,简单产品就可以正常工作,并且在添加全部6个产品之前,该代码也适用于变体产品。
我很难找到代码中的问题,但它仍然适用于简单的产品; 但是同样无法加载版本产品的产品页面,也无法在后端显示每个版本所节省的价格。
任何帮助将不胜感激!
此外,日志文件中没有任何内容作为错误生成。
谢谢,
约书亚记
好像您缺少一个函数sfprice_corporate_applicable()
在您的脚本中,您尝试访问该功能,但是您的项目中不存在该功能。
所以像这样创建新功能
function sfprice_corporate_applicable() {
return (bool) ( current_user_can('corporate') && ( !is_admin() || is_ajax() ) );
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.