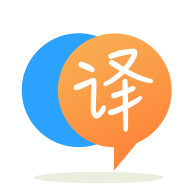
[英]Get Index of a Dictionary from an array of Dictionaries for a given Key and Value
[英]How to reverse the index in array of dictionaries?
我的数组字典键值对与此相似
[0]
key : x
value : 1
[1]
key : y
value : 2
[2]
key : z
value : 3
但是我需要反转字典的索引。 我需要将上面的字典转换为此:
[0]
key : z
value : 3
[1]
key : y
value : 2
[2]
key : x
value : 1
我尝试了Reverse()函数。 但这没有用。 我不知道该如何实现。 谁能帮我这个 ?
我该如何实现?
您不应该假设字典是有序的。 他们不是。
如果您想要一个有序的数组,则应使用SortedDictionary
。 如果需要,您也可以在那儿颠倒顺序。 您应该为此使用一个自定义比较器(从此处更改):
class DescendedStringComparer : IComparer<string>
{
public int Compare(string x, string y)
{
int ascendingResult = Comparer<string>.Default.Compare(x, y);
// turn the result around
return 0 - ascendingResult;
}
}
//
SortedDictionary<string, string> test
= new SortedDictionary<string, string>(new DescendedDateComparer());
例如,您可以使用foreach
进行迭代。 结果将按降序排列。
函数Enumerable.Reverse()返回IEnumerable而不是数组。 您是否忘了将反向序列设置为数组? 以下为我工作:
class Program
{
static void Main(string[] args)
{
var dict = new Dictionary<string, int>();
dict.Add("x", 1);
dict.Add("y", 2);
dict.Add("z", 3);
var reversed = dict.ToArray().Reverse();
var reversedArray = reversed.ToArray();
Console.ReadKey();
}
}
您应该使用SortedDictionary并在构造上传递密钥
public sealed class CompareReverse<T> : IComparer<T>
{
private readonly IComparer<T> original;
public CompareReverse(IComparer<T> original)
{
this.original = original;
}
public int Compare(T left, T right)
{
return original.Compare(right, left);
}
}
在您的主要通话中
var mydictionary = new SortedDictionary<int, string>(
new CompareReverse<int>(Comparer<int>.Default));
例如:
var mydictionary = new SortedDictionary<int, string>(
new CompareReverse<int>(Comparer<int>.Default));
dictionary.add(1, "1");
dictionary.add(2, "2");
dictionary.add(3, "3");
dictionary.add(4, "4");
SortedDictionary按键而不是按值排序。
这是一种反转字典中值顺序的干净方法:
List<KeyValuePair<string, string>> srcList = aDictionary.ToList();
srcList.Sort(
delegate(KeyValuePair<string, string> firstPair,
KeyValuePair<string, string> nextPair)
{
return firstPair.Value.CompareTo(nextPair.Value);
}
);
Dictionary<string, string> outputDictionary = new Dictionary<string, string>();
int listCount = srcList.Count;
for(int i = 0; i < listCount; i++) {
int valueIndex = i + 1;
outputDictionary.Add(srcList[i].Key, srcList[valueIndex]);
}
如果要输出的是KeyValuePairs列表而不是Dictionary,则可以用以下示例替换我示例中的最后6行代码:
List<KeyValuePair<string, string>> destList = new List<KeyValuePair<string, string>>();
int listCount = srcList.Count;
for(int i = 0; i < listCount; i++) {
int valueIndex = i + 1;
destList.Add(new KeyValuePair<string, string>(srcList[i].Key, srcList[valueIndex]));
}
你可以这样做 :
for (var i = dictionary.Count - 1; i >= 0; i--)
dictionary.Values.ElementAt(i), dictionary.Keys.ElementAt(i);
编辑:转到此处-> 如何在C#中以相反的顺序(从最后到第一)迭代字典<string,string>?
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.