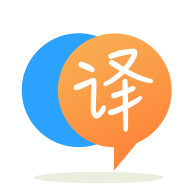
[英]How is immutability actually implemented in String class and mutability in StringBuffer and StringBuilder in code?
[英]Java - String immutability and Array mutability
我知道数组a
和b
指向与字符串s1
, s2
不相同的地方。 为什么?
码:
String[] a = {"a","b","c"};
String[] b = a;
a[0] = "Z";
String s1 = "hello";
String s2 = s1;
s1 = "world";
System.out.println(Arrays.toString(a) + " - a"); //a and b are same
System.out.println(Arrays.toString(b) + " - b");
System.out.println(s1 + " "+ s2); // s1 and s2 are not same.
输出:
[Z, b, c] - a
[Z, b, c] - b
world hello
在点(2)处, s1
和s2
指向池中的相同文字。 但是当你改变s1
,会创建另一个文字并且字符串不再指向同一个地方。
String s1 = "hello";
String s2 = s1; (2)
s1 = "world";
您可以通过打印结果来验证这一点
s1 == s2
请注意,我没有使用equals
因为我对比较引用感兴趣。 现在,只要为s1
指定不同的值,事情就会如下所示:
+-------+
| world | <- s1
+-------+
+-------+
| hello | <- s2
+-------+
数组是对象,因此如果更改数组元素,则更改a
和b
指向的对象。
字符串是不可变对象,因此您只能更改引用,在调用s1 = "world"
时您正在执行的操作。
更深入的解释:
字符串:
String s1 = "hello"; //creates a new String object with value "hello" and assigns a reference to that object to s1
String s2 = s1; //s2 now points to the same object as s1
s1 = "world"; //creates a new String object with value "world" and assigns a reference to that object to s1
所以最后s1将指向一个值为“world”的不同字符串对象。
阵列:
String[] a = {"a","b","c"}; //creates the array and assigns a reference to it to variable a
String[] b = a; //copies the reference of a to b, so both point to the same object
a[0] = "Z"; //changes the 1st element in the array, a and b still point to it
在这里,你永远不会改变的实际值a
是引用数组,但你而改变数组的第一个元素的值,它是本身的字符串“Z”的引用。
a
和b
是对同一个Array的引用(内存中有一个Array对象。)
a ---> ["a", "b", "c"] <---- b
您正在使用以下行更改此数组值:
a[0] = "Z"
所以你知道在内存中有这个:
a ---> ["Z", "b", "c"] <---- b
对于字符串,它是不同的。
首先,您有两个指向相同值的变量:
String s1 = "hello";
String s2 = s1;
你在内存中有这个:
s1 ---> "hello" <---- s2
但是,您可以使用以下代码将s1分配给新值:
s1 = "world";
变量s2仍然指向字符串“hello”。 现在内存中有2个字符串对象。
s1 ---> "world"
s2 ---> "hello"
在Java中,字符串是不可变的,但数组是可变的。 另见这个问题 。
请注意,如果您定义了一个类,则行为将更接近Array。
public class Foo() {
private int _bar = 0;
public void setBar(int bar) {
this._bar = bar
}
public void getBar() {
return this._bar;
}
}
Foo f1 = new Foo();
Foo f1 = f2;
你有这个:
f1 ----> Foo [ _bar = 0 ] <---- f2
您可以处理该对象:
f1.setBar(1)
f2.setBar(2) // This is the same object
这使得某些东西有点像“数组”:
f1 ----> Foo [ _bar = 2 ] <---- f2
但是如果你将f2分配给另一个值,你得到这个:
f2 = new Foo();
这会在内存中创建一个新值,但仍保留指向第一个对象的第一个引用。
f1 ----> Foo [ _bar = 2 ]
f2 ----> Foo [ _bar = 0 ]
关键的区别在于重新分配与修改。
重新分配 ( x = ...
)使变量指向新对象但不更改基础对象,因此不会更改指向原始对象的任何其他变量。
修改 ( x.something = ...
或x[...] = ...
)仅更改基础对象,因此指向同一对象的任何其他变量都将反映更改。
请注意,字符串是不可变的 - 它们没有修改它们的方法,也没有(非最终的)公共成员变量,所以它们不能被修改(没有反射)。
你可以想到人们指着书籍是你的变量而书本身就是底层的对象。
很多人都可以指出同一本书。 您可以让某人指向不同的图书,而无需更改图书本身或其他任何人指向的图书。 如果你在书中写一些东西,有人指着指向那本书的每个人都会看到这些变化。
int[] a = {0,1,2,3,4}; // Assign a to, let's say, "Object 1"
int[] b = {5,6,7,8}; // Assign b to, let's say, "Object 2"
int[] c = a; // Assign c to Object 1
a[0] = 2; // Change Object 1's 0th element
assert c[0] == 2; // c points to Object 1, whose 0th element is now 2
a = b; // Assign a to Object 2
assert c[0] == 2; // c still points to Object 1
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.