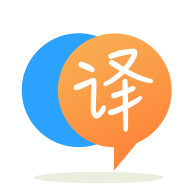
[英]Spring java config to invoke a public non static method of a singleton class with private constructor
[英]create a singleton class in java using public constructor
我是否必须使用私有构造函数来创建类单例? 除了使用私有构造函数之外还有其他方法吗? 我不能使用公共构造函数创建类单例吗?
如果你的类有一个公共构造函数,那么任何人都可以随时创建它的实例。 所以它不再是单身人士了。 对于单身人士,只能存在一个实例。
它必须是私有构造函数的原因是因为您希望阻止人们自由地使用它来创建多个实例。
只有当您能够检测到已存在的实例并禁止创建另一个实例时,才可能使用公共构造函数。
除了使用私有构造函数之外没有办法吗? 我们不能使用公共构造函数创建类单例吗?
是的,它实际上是可能的 ,例如:
class Singleton
{
private static Singleton instance = null;
public Singleton() throws Exception //Singleton with public constructor
{
if(instance != null)
throw new Exception("Instance already exist");
//Else, do whatever..such as creating an instance
}
}
如果你真的必须使用公共构造函数,那么你应该检查实例是否为null并抛出异常。
if(instance != null)
使用public constructor
创建Singleton对象的一种方法,如果object已存在,则从构造public constructor
throw exception
。
例如:
public class Singleton {
private static Singleton singleton;
private String hello;
public Singleton() throws Exception {
if (singleton != null) {
throw new Exception("Object already exist");
}
}
public static Singleton getInstance() throws Exception {
if (singleton == null) {
singleton = new Singleton();
}
return singleton;
}
public String getHello() {
return hello;
}
public void setHello(String hello) {
this.hello = hello;
}
public static void main(String[] args) {
try {
Singleton single1 = Singleton.getInstance();
single1.setHello("Hello");
System.out.println(single1.getHello());
Singleton single2 = Singleton.getInstance();
System.out.println(single2.getHello());
Singleton single3 = new Singleton();
System.out.println(single3.getHello());
} catch (Exception ex) {
}
}
}
通常,当你应该只有一个类的一个对象时使用单例模式(例如,工厂通常被实现为单例)。
public
modifier允许任何人访问您的方法(以及您的构造函数)。 在这种情况下,任何人都可以创建您的类的实例,因此它不再是单例。 通过将private
修饰符设置为构造函数,您确信它不能在类之外使用 - 没有人会再创建该类的对象。
public class SingletonExample {
private static final SingletonExample instance;
// Initialize instance object in static block - done while loading the class by class loader
static {
instance = new SingletonExample();
}
private SingletonExample(){}
public static SingletonExample getInstance(){
return instance;
}
// Method used to create java.test.DateFormat using Oracle SQL Date pattern
public DateFormat createDateFormat(String mask){
if("DD-MM-YYYY HH24:MI:SS".equals(mask)){
return new SimpleDateFormat("dd-MM-yyyy HH:mm:ss");
} else if("DD-MM-YYYY".equals(mask)){
return new SimpleDateFormat("dd-MM-yyyy");
} else {
throw new UnsupportedOperationException("Unsupported mask - " + mask);
}
}
}
和用法示例
public class SingletonExampleTest{
public static void main(String... args){
java.util.Date date = Calendar.getInstance().getTime();
SingletonExample singleton = SingletonExample.getInstance();
String mask = "DD-MM-YYYY";
DateFormat df = singleton.createDateFormat(mask);
System.out.println(df.format(date));
}
}
私有构造函数是一个原因,为什么你的类是Singleton。
这个例子很好地说明了如何使用getInstance()
来使用Singleton类
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.