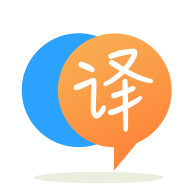
[英]Using an ItemsControl inside of a ListView ItemTemplate with XAML
[英]Items added to ItemsControl not using ItemTemplate
我对 wpf 比较陌生,所以我提前为任何不良的编码实践道歉。
我正在尝试创建一个仪表板应用程序,用户可以通过添加不同的控件(表格、图表等)来自定义它并移动它们/调整它们的大小。
我最初使用 Canvas 来绘制我的控件,并且可以进行移动和调整大小。 由于需要动态的内容,我切换到使用 ItemsControl,如下所示:
<ItemsControl Name="dashboardCanvas" ItemsSource="{Binding Path=CanvasContents}" Grid.Row="1">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<Canvas/>
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
<ItemsControl.Resources>
<ControlTemplate x:Key="MoveThumbTemplate" TargetType="{x:Type local:MoveThumb}">
<Rectangle Fill="Transparent"/>
</ControlTemplate>
<ControlTemplate x:Key="ResizeDecoratorTemplate" TargetType="Control">
<Grid>
<local:ResizeThumb Height="2" Cursor="SizeNS" Margin="0 -4 0 0" VerticalAlignment="Top" HorizontalAlignment="Stretch"/>
<local:ResizeThumb Width="2" Cursor="SizeWE" Margin="-4 0 0 0" VerticalAlignment="Stretch" HorizontalAlignment="Left"/>
<local:ResizeThumb Width="2" Cursor="SizeWE" Margin="0 0 -4 0" VerticalAlignment="Stretch" HorizontalAlignment="Right"/>
<local:ResizeThumb Height="2" Cursor="SizeNS" Margin="0 0 0 -4" VerticalAlignment="Bottom" HorizontalAlignment="Stretch"/>
<local:ResizeThumb Width="7" Height="7" Cursor="SizeNWSE" Margin="-6 -6 0 0" VerticalAlignment="Top" HorizontalAlignment="Left"/>
<local:ResizeThumb Width="7" Height="7" Cursor="SizeNESW" Margin="0 -6 -6 0" VerticalAlignment="Top" HorizontalAlignment="Right"/>
<local:ResizeThumb Width="7" Height="7" Cursor="SizeNESW" Margin="-6 0 0 -6" VerticalAlignment="Bottom" HorizontalAlignment="Left"/>
<local:ResizeThumb Width="7" Height="7" Cursor="SizeNWSE" Margin="0 0 -6 -6" VerticalAlignment="Bottom" HorizontalAlignment="Right"/>
</Grid>
</ControlTemplate>
<ControlTemplate x:Key="DesignerItemTemplate">
<Grid DataContext="{Binding RelativeSource={RelativeSource TemplatedParent}}">
<local:MoveThumb Template="{StaticResource MoveThumbTemplate}" DataContext="{Binding RelativeSource={RelativeSource TemplatedParent}}" Cursor="SizeAll"/>
<Control Template="{StaticResource ResizeDecoratorTemplate}"/>
<ContentPresenter Content="{TemplateBinding ContentControl.Content}"/>
<Button Content="x" VerticalAlignment="Top" HorizontalAlignment="Right" Width="10" Height="10" Click="Button_Click"/>
</Grid>
</ControlTemplate>
</ItemsControl.Resources>
<ItemsControl.ItemTemplate>
<DataTemplate>
<Grid DataContext="{Binding RelativeSource={RelativeSource TemplatedParent}}">
<local:MoveThumb Template="{StaticResource MoveThumbTemplate}" DataContext="{Binding RelativeSource={RelativeSource TemplatedParent}}" Cursor="SizeAll"/>
<Control Template="{StaticResource ResizeDecoratorTemplate}"/>
<ContentPresenter Content="{TemplateBinding ContentControl.Content}"/>
<Button Content="x" VerticalAlignment="Top" HorizontalAlignment="Right" Width="10" Height="10" Click="Button_Click"/>
</Grid>
</DataTemplate>
</ItemsControl.ItemTemplate>
<ItemsControl.ItemContainerStyle>
<Style>
<Setter Property="Canvas.Top" Value="{Binding Path=Y}"/>
<Setter Property="Canvas.Left" Value="{Binding Path=X}"/>
</Style>
</ItemsControl.ItemContainerStyle>
</ItemsControl>
我现在有一个问题,我添加到 ItemsControl 的任何控件都没有应用模板。
我看过这个问题: 为什么 ItemsControl 不使用我的 ItemTemplate? 但是我不能将 ItemsControl 继承到我的控件中,因为它们已经从 ContentControls 继承。
这是我的主要控制对象:
class TableControl: DashboardItem
{
public TableControl()
{
Width = 100;
Height = 100;
Content = new Ellipse
{
Fill = new SolidColorBrush(Colors.Green),
IsHitTestVisible = false
};
}
public int X
{
get
{
return 10;
}
}
public int Y
{
get
{
return 200;
}
}
}
此刻的 DashboardItem 很简单:
class DashboardItem : ContentControl
{
}
在后面的代码中,我有一个 DashboardItems 的 ObservableCollection,ItemsControl 绑定到它。
我将如何强制将 ItemsControl 模板应用于控件中的所有项目?
您的代码中有奇怪的混合。 您不能有ContentControl
的ObservableCollection
。 使您的对象DashboardItems
,真正的业务对象(或视图模型对象),可能持有 X 和 Y 属性,但没有ContentControl
的继承。
如果您提供 GUI 对象,WPF 似乎并不关心您的模板。
如果您需要为 Items Control 的不同项目设置不同的外观,那么您将能够使用
-a TemplateSelector(用于为给定行选择模板)
- 或 DataItemTemplate 的 DataType 属性
只需添加另一个解决方案。 正如公认的答案指出的那样,如果您直接使用ItemsControl
,则您传入的任何 GUI 对象都将按原样排列在ItemsPanel
上,从而完全绕过您的DataTemplate
。 (如果不是 GUI 对象,则首先将其包装在ContentPresenter
中。)
为了解决这个问题,您可以创建一个ItemsControl
的子类,您可以强制它始终为您的项目创建一个容器。 您可以通过为IsItemItsOwnContainerOverride
返回false
来执行此操作。 这告诉控件必须首先将项目包装在容器中——这在ItemsControl
的默认实现中是一个简单的ContentPresenter
——因此它将始终应用任何给定的ItemTemplate
。
这是代码...
public class EnsureContainerItemsControl : ItemsControl {
protected override bool IsItemItsOwnContainerOverride(object item) {
return false; // Force the control to always generate a container regardless of what was passed in
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.