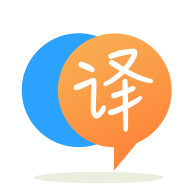
[英]AJAX - Sending knockout observables as JSON object to server using AJAX
[英]Sending a JSON object to a server using AJAX
我正在开发一个需要将表单数据序列化为JSON对象并异步使用AJAX将它们发送到服务器的应用程序(因为该服务器仅接受JSON对象)。 有两种形式可供考虑:
frontend.html
<div class="login">
<h>Login</h>
<form id="login_form_id" onsubmit="sign_in_client()">
<label>Email: </label><input id="email0" type="email" name="l_email" required>
<br>
<label>Password: </label><input id="password0" type="password" name="l_password" required>
<br><br>
<input type="submit" value="Submit">
</form>
</div>
<div class="signup">
<h>Signup</h>
<form id="signup_form_id" onsubmit="sign_up_client()">
<label>First Name: </label><input id="fname1" type="text" name="s_fname" required>
<br>
<label> Last Name: </label><input id="lname1" type="text" name="s_lname" required>
<br>
<label> City: </label><input id="city1" type="text" name="s_city" required>
<br>
<label> Country: </label><input id="country1" type="text" name="s_country" required>
<br>
<label> Male: </label><input id="gender1" type="radio" name="sex" value="male" required>
<br>
<label> Female: </label><input type="radio" name="sex" value="female" required>
<br>
<label> Email: </label><input id="email1" type="email" name="s_email" required>
<br>
<label> Password: </label><input id="password1" type="password" name="s_password" required>
<br>
<label> Repeat Pas: </label><input id="password2" type="password" name="s_rpassword" required>
<br>
<label> </label><input type="submit" value="Submit">
</form>
</div>
下面是处理表单输入解析的代码:
frontend.js
function sign_up_client()
{
var xmlhttp;
var fields = {};
if (window.XMLHttpRequest)
{// code for IE7+, Firefox, Chrome, Opera, Safari
xmlhttp=new XMLHttpRequest();
}
else
{// code for IE6, IE5
xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttp.onreadystatechange=function()
{
if (xmlhttp.readyState==4 && xmlhttp.status==200)
{
document.getElementById("frontEnd").innerHTML=xmlhttp.responseText;
}
}
// Open connection to server asynchronously to the sign_up route function
xmlhttp.open("POST", "sign_up", true);
// Set the content type to JSON objects
xmlhttp.setRequestHeader("Content-type","application/json");
// Send the form parameters needed for a sign-up operation
// Serialize them into a JSON object first
$("signup_form_id").find("input, textarea, select").each(function() {
var inputType = this.tagName.toUpperCase() === "INPUT" && this.type.toUpperCase();
if (inputType !== "BUTTON" && inputType !== "SUBMIT") {
}
xmlhttp.send(inputType);
});
}
从该问题复制了用于解析表单数据的代码。 我不清楚如何构造JSON对象。 上面的示例中是否包含按钮和提交类型? 是否正确选择了需要解析其输入的表单(按ID)?
函数的末尾是inputType,它准备好按原样发送适当的JSON对象吗?
编辑#1:
frontend.html
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="client.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script type="text/javascript" src="client.js"></script>
<script type="text/javascript" src="serverstub.js"></script>
</head>
<body>
<div class="welcome">
<img src="wimage.png" alt="Twidder Icon;" >
<div class="login">
<h>Login</h>
<form id="signin_form_id" onsubmit="sign_in_client()">
<label>Email: </label><input type="email" name="l_email" required>
<br>
<label>Password: </label><input id="password0" type="password" name="l_password" required>
<br><br>
<input type="submit" value="Submit">
</form>
</div>
<div class="signup">
<h>Signup</h>
<form onsubmit="sign_up_client()">
<label>First Name: </label><input id="fname1" type="text" name="s_fname" required>
<br>
<label> Last Name: </label><input id="lname1" type="text" name="s_lname" required>
<br>
<label> City: </label><input id="city1" type="text" name="s_city" required>
<br>
<label> Country: </label><input id="country1" type="text" name="s_country" required>
<br>
<label> Male: </label><input id="gender1" type="radio" name="sex" value="male" required>
<br>
<label> Female: </label><input type="radio" name="sex" value="female" required>
<br>
<label> Email: </label><input id="email1" type="email" name="s_email" required>
<br>
<label> Password: </label><input id="password1" type="password" name="s_password" required>
<br>
<label> Repeat Pas: </label><input id="password2" type="password" name="s_rpassword" required>
<br>
<label> </label><input type="submit" value="Submit">
</form>
</div>
</div>
</body>
</html>
frontend.js
function sign_up_client()
{
var xmlhttp;
var jsonObject = {};
if (window.XMLHttpRequest)
{// code for IE7+, Firefox, Chrome, Opera, Safari
xmlhttp=new XMLHttpRequest();
}
else
{// code for IE6, IE5
xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttp.onreadystatechange=function()
{
if (xmlhttp.readyState==4 && xmlhttp.status==200)
{
document.getElementById("myDiv").innerHTML=xmlhttp.responseText;
}
}
// Open connection to server asynchronously towards the sign_up route function
xmlhttp.open("POST", "sign_in", true);
// Set the content type to JSON objects
xmlhttp.setRequestHeader("Content-type","application/json");
// Send the form parameters needed for a sign-up operation
// Serialize them into a JSON object first
$("form").on("submit", function() {
var jsonObject = {};
$(".signup").find("input, textarea, select").map(function(index, elem) {
//Ingore types such as button, submit and radio
elem.type.match(/button|submit|radio/i) === null &&
(jsonObject[elem["name"]] = elem.value || "")
//If type = radio, grab the selected radio's value
elem.type.match(/radio/i) !== null &&
elem.checked && (jsonObject[elem["name"]] = elem.value || "")
});
alert (JSON.stringify(jsonObject, null, 4));
return false;
});
alert (JSON.stringify(jsonObject, null, 4));
// Send the JSON object
xmlhttp.send(jsonObject);
}
function sign_in_client()
{
var xmlhttp;
var jsonObject = {};
if (window.XMLHttpRequest)
{// code for IE7+, Firefox, Chrome, Opera, Safari
xmlhttp=new XMLHttpRequest();
}
else
{// code for IE6, IE5
xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttp.onreadystatechange=function()
{
if (xmlhttp.readyState==4 && xmlhttp.status==200)
{
document.getElementById("myDiv").innerHTML=xmlhttp.responseText;
}
}
// Open connection to server asynchronously towards the sign_up route function
xmlhttp.open("POST", "sign_in", true);
// Set the content type to JSON objects
xmlhttp.setRequestHeader("Content-type","application/json");
// Send the form parameters needed for a sign-up operation
// Serialize them into a JSON object first
$("form").on("submit", function() {
var jsonObject = {};
$(".login").find("input, textarea, select").map(function(index, elem) {
//Ingore types such as button, submit and radio
elem.type.match(/button|submit|radio/i) === null &&
(jsonObject[elem["name"]] = elem.value || "")
//If type = radio, grab the selected radio's value
elem.type.match(/radio/i) !== null &&
elem.checked && (jsonObject[elem["name"]] = elem.value || "")
});
alert (JSON.stringify(jsonObject, null, 4));
return false;
});
alert (JSON.stringify(jsonObject, null, 4));
// Send the JSON object
xmlhttp.send(jsonObject);
}
试试这个例子:
在下面的代码中,使用jQuery ajax语法,因为它对我来说似乎更加简化。 要从表单字段获取值,请使用
serialize
方法。$('form').on('submit', sign_up_client); function sign_up_client(e) { e.preventDefault(); var formJson = []; $(this).find(':input').each(function (index, elem) { var inputType = this.tagName.toUpperCase() === "INPUT" && var formObj = {}; if (inputType === "RADIO") { if ($(elem).is(":checked")) { formObj[$(elem).attr('name')] = $(elem).val(); formJson.push(formObj); } } else if (inputType !== "BUTTON" && inputType !== "SUBMIT") formObj[$(elem).attr('name')] = $(elem).val(); formJson.push(formObj); } }); $.ajax({ type: "POST", url: "test.php", data: formJson, dataType: "json", success: function (data) { }, error: function () { alert('error handing here'); } }); }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <div class="login"> <h>Login</h> <form id="login_form_id" method="post"> <label>Email:</label> <input id="email0" type="email" name="l_email" required> <br> <label>Password:</label> <input id="password0" type="password" name="l_password" required> <br> <br> <input type="submit" value="Submit"> </form> </div> <div class="signup"> <h>Signup</h> <form id="signup_form_id" method="post"> <label>First Name:</label> <input id="fname1" type="text" name="s_fname" required> <br> <label>Last Name:</label> <input id="lname1" type="text" name="s_lname" required> <br> <label>City:</label> <input id="city1" type="text" name="s_city" required> <br> <label>Country:</label> <input id="country1" type="text" name="s_country" required> <br> <label>Male:</label> <input id="gender1" type="radio" name="sex" value="male" required> <br> <label>Female:</label> <input type="radio" name="sex" value="female" required> <br> <label>Email:</label> <input id="email1" type="email" name="s_email" required> <br> <label>Password:</label> <input id="password1" type="password" name="s_password" required> <br> <label>Repeat Pas:</label> <input id="password2" type="password" name="s_rpassword" required> <br> <label></label> <input type="submit" value="Submit"> </form> </div>
这是针对特定情况从表单字段构造JSON对象的快速方法。
var o = {};
$(".signup").find("input, textarea, select").map(function(index, elem) {
//Ingore types such as button, submit and radio
elem.type.match(/button|submit|radio/i) === null &&
(o[elem["name"]] = elem.value || "")
//If type = radio, grab the selected radio's value
elem.type.match(/radio/i) !== null &&
elem.checked && (o[elem["name"]] = elem.value || "")
});
现在,您可以将o
作为JSON对象发送。
这是相同的演示 。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.