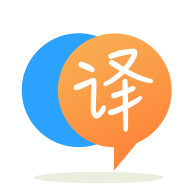
[英]Do multiple awaits to the same Task from a single thread resume in FIFO order?
[英]Is the order of resume multiple await expressions to the same task is guaranteed?
我正在学习使用C#4.5进行异步/等待,并想知道当多个“等待者”正在等待同一任务时会发生什么。
我正在做一些实验,我想我了解发生了什么。 但是,我有一些问题要问:
看一下这段代码:
AsyncAwaitExperiment.fxml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Threading;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
namespace View
{
/// <summary>
/// Interaction logic for AsyncAwaitExperiment.xaml
/// </summary>
public partial class AsyncAwaitExperiment : Window
{
private CancellationTokenSource token;
private Task longRunningTask;
public AsyncAwaitExperiment()
{
InitializeComponent();
}
private void startAsyncOperation(object sender, RoutedEventArgs e)
{
if (longRunningTask != null && !longRunningTask.IsCompleted)
{
return;
}
else
{
longRunningTask = null;
token = new CancellationTokenSource();
}
Action action = () =>
{
while (!token.Token.IsCancellationRequested)
{
Thread.Sleep(100);
}
};
longRunningTask = Task.Factory.StartNew(
action,
token.Token,
TaskCreationOptions.LongRunning,
TaskScheduler.Current);
}
private void stopOperation(object sender, RoutedEventArgs e)
{
if (longRunningTask == null
|| longRunningTask.IsCompleted
|| token == null
|| token.Token.IsCancellationRequested)
{
return;
}
token.Cancel();
}
private async void firstAwait(object sender, RoutedEventArgs e)
{
if (longRunningTask == null || longRunningTask.IsCompleted)
return;
await longRunningTask;
Console.WriteLine("First Method");
}
private async void secondAwait(object sender, RoutedEventArgs e)
{
if (longRunningTask == null || longRunningTask.IsCompleted)
return;
await longRunningTask;
Console.WriteLine("Second Method");
}
}
}
AsyncAwaitExperiment.fxml
<Window x:Class="View.AsyncAwaitExperiment"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="AsyncAwaitExperiment" Height="300" Width="300">
<Grid>
<StackPanel HorizontalAlignment="Center" VerticalAlignment="Center">
<Button Content="Start Async" Margin="3" Click="startAsyncOperation" />
<Button Content="Stop Async" Margin="3" Click="stopOperation" />
<Button Content="Await First" Margin="3" Click="firstAwait" />
<Button Content="Await Second" Margin="3" Click="secondAwait" />
</StackPanel>
</Grid>
</Window>
此代码的行为是:
通过这段代码和一些断点,我注意到了一些行为。
问题是:
PS:对不起,我的英语不好:/
您不应对活动的恢复顺序承担任何责任。 假设将来有人设法编写一个完全是多线程的新UI系统,那么您甚至期望UI线程可以并行运行(注意:没有人认为编写多线程UI系统是理智的。当前;即使“同时”恢复执行两个任务,也可能会无限期地暂停其中一项)
如果有活动之间的依赖关系在你的代码,使他们明确。 否则,假设它们可能以任何顺序发生并且可能被交错。 那是编程的唯一明智的方法。
最后,没有。 使用CancellationToken
,就完成了。 如果需要另一个,则需要创建一个新的。 实际上,这使考虑多个任务/活动变得更容易考虑-从取消令牌到取消令牌都不可能。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.