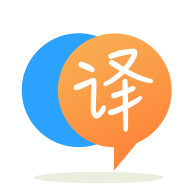
[英]AES encryption/decryption between C# (encryption) and Java (decryption)
[英]AES string encryption/decryption in C and java
我正在尝试加密C和java中的字符串以查看两端的结果是否相同,然后尝试在每一个中解密该结果,但是当我运行我的代码时,每个结果看起来非常不同
这是我的C代码:
#include <string.h>
#include <openssl/aes.h>
char key[] = "thisisasecretkey";
int main(){
unsigned char text[]="hello world";
unsigned char enc_out[80];
unsigned char dec_out[80];
AES_KEY enc_key, dec_key;
AES_set_encrypt_key(key, 128, &enc_key);
AES_encrypt(text, enc_out, &enc_key);
printf("original:%s\t",text);
printf("\nencrypted:%s\t",enc_out);
printf("\n");
return 0;
}
这是我的java代码:
package com.caja.utilidades;
import java.security.Key;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public class MainClass {
private static final String ALGORITHM = "AES";
private static final String keyValue = "thisisasecretkey";
public static void main(String[] args) throws Exception {
System.out.println(encrypt("hello world"));
}
public static String encrypt(String valueToEnc) throws Exception {
Key key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encValue = cipher.doFinal(valueToEnc.getBytes());
return new String(encValue);
}
private static Key generateKey() throws Exception {
Key key = new SecretKeySpec(keyValue.getBytes(), ALGORITHM);
return key;
}
}
在C我正在使用openssl库,对于C和java我正在使用eclipse,提前感谢。
我在代码中做了一些更改,以比较两个程序中的结果
新代码
C代码:
#include <string.h>
#include <openssl/aes.h>
char key[] = "thisisasecretkey";
int main(){
unsigned char text[]="hello world";
unsigned char enc_out[80];
unsigned char dec_out[80];
AES_KEY enc_key, dec_key;
AES_set_encrypt_key(key, 128, &enc_key);
AES_encrypt(text, enc_out, &enc_key);
int i;
printf("original:\t");
for(i=0;*(text+i)!=0x00;i++)
printf("%02X ",*(text+i));
printf("\nencrypted:\t");
for(i=0;*(enc_out+i)!=0x00;i++)
printf("%02X ",*(enc_out+i));
printf("\n");
printf("original:%s\t",text);
printf("\nencrypted:%s\t",enc_out);
printf("\ndecrypted:%s\t",dec_out);
printf("\n");
return 0;
}
java代码:
import java.security.Key;
import java.security.MessageDigest;
import java.util.Arrays;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public class MainClass {
private static final String ALGORITHM = "AES";
private static final String keyValue = "thisisasecretkey";
final protected static char[] hexArray = "0123456789ABCDEF".toCharArray();
public static void main(String[] args) throws Exception {
System.out.println(encrypt("hello world"));
}
public static String encrypt(String valueToEnc) throws Exception {
Key key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encValue = cipher.doFinal(valueToEnc.getBytes());
System.out.println(bytesToHex(encValue));
return new String(encValue);
}
private static Key generateKey() throws Exception {
byte[] key2 = keyValue.getBytes("UTF-8");
MessageDigest sha = MessageDigest.getInstance("SHA-1");
key2 = sha.digest(key2);
key2 = Arrays.copyOf(key2, 16);
Key key = new SecretKeySpec(key2, ALGORITHM);
return key;
}
public static String bytesToHex(byte[] bytes) {
char[] hexChars = new char[bytes.length * 2];
for ( int j = 0; j < bytes.length; j++ ) {
int v = bytes[j] & 0xFF;
hexChars[j * 2] = hexArray[v >>> 4];
hexChars[j * 2 + 1] = hexArray[v & 0x0F];
}
return new String(hexChars);
}
}
C结果:
original: 68 65 6C 6C 6F 20 77 6F 72 6C 64
encrypted: 17 EF AC E9 35 B1 81 67 EA 7D BB 99 E2 4F D1 E8 70 35 62 BD
original:hello world
encrypted:ï¬é5±?gê}»™âOÑèp5b½
decrypted:hello world
Java结果:
encrypted: 764AA3D074EE1399858ECD7076957D21
encrypted: vJ£Ðtî™…ŽÍpv•}!
我将与Java方面交谈; 我相信同样的评论也适用于C版本。
您不希望获取加密的字节数组并将其转换为String。 特别是,这里:
byte[] encValue = cipher.doFinal(valueToEnc.getBytes());
return new String(encValue);
问题是new String(byte[] b)
将把字节数组解释为用默认编码编码的字符串。 当然,字节数组不是编码字符串,因此这不是特别有用。
如果您想获得一个可用于比较加密字节数组的字符串(可视化),典型的方法是对字节数组进行十六进制编码。 请参见如何在Java中将字节数组转换为十六进制字符串? 如何在C中将缓冲区(字节数组)转换为十六进制字符串? 欲获得更多信息。 当然,有许多库支持此功能。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.