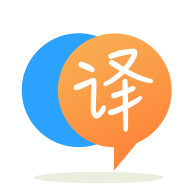
[英]Find out the Number of ways in which two queens intersects (won't be stable) on n*n chessboard?
[英]ArrayList won't hold objects correctly (N Queens example)
我已经解决了N Queens问题,返回了一个包含所有可能解决方案的ArrayList。
我知道算法本身是正确的,因为它可以打印出正确的解决方案。 但是,当我遍历返回的ArrayList时,看不到正确的解决方案。 我只看到空的网格(即4行0)。
谁能告诉我为什么我要在程序中打印正确的答案,但是没有将它们正确地添加到ArrayList中?
这是我提供上下文的代码,但是您应该特别注意queens()中的第一个if语句,其中printGrid()打印我(假定)添加到列表中的正确解决方案。 但是,当我遍历main方法中的列表时,得到0。
public class Question {
static int GRID_SIZE = 4;
public static ArrayList<int[][]> queens(int[][] grid) {
ArrayList<int[][]> list = new ArrayList<int[][]>();
queens(grid, 0, list);
return list;
}
public static void queens(int[][] grid, int row, ArrayList<int[][]> list) {
// if you reached the end of the grid successfully
if (row == GRID_SIZE) {
//print grid - shows correct solution
printGrid(grid);
//adding correct solution to list?
list.add(grid);
}
else {
//iterate through columns
for (int i = 0; i < GRID_SIZE; i++) {
// check if the spot is free
if (valid(grid, row, i)) {
// place queen
grid[row][i] = 1;
// move onto next row
queens(grid, row + 1, list);
//backtrack if placement of queen does not allow successful solution
grid[row][i] = 0;
}
}
}
}
// need to check previous rows to see if it's safe to place queen
public static boolean valid(int[][] grid, int row, int col) {
// check columns of each previous row
for (int i = 0; i < row; i++) {
if (grid[i][col] == 1) {
return false;
}
}
// check left diagonal
int rowNum = row;
int colNum = col;
while (rowNum >= 0 && colNum >= 0) {
if (grid[rowNum--][colNum--] == 1) {
return false;
}
}
// check right diagonals
rowNum = row;
colNum = col;
while (rowNum >= 0 && colNum < GRID_SIZE) {
if (grid[rowNum--][colNum++] == 1) {
return false;
}
}
return true;
}
public static void printGrid(int[][] grid) {
System.out.println("---------");
for (int i = 0; i < grid.length; i++) {
for (int j = 0; j < grid.length; j++) {
System.out.print(grid[i][j] + " ");
}
System.out.println();
}
System.out.println("---------");
}
public static void main(String[] args) {
int[][] grid = new int[GRID_SIZE][GRID_SIZE];
for (int i = 0; i < grid.length; i++) {
for (int j = 0; j < grid.length; j++) {
grid[i][j] = 0;
}
}
ArrayList<int[][]> list = queens(grid);
System.out.println(list.size());
printGrid(list.get(0));
}
}
这是输出:
---------
0 1 0 0
0 0 0 1
1 0 0 0
0 0 1 0
---------
---------
0 0 1 0
1 0 0 0
0 0 0 1
0 1 0 0
---------
ITERATING THROUGH ARRAYLIST:
---------
0 0 0 0
0 0 0 0
0 0 0 0
0 0 0 0
---------
---------
0 0 0 0
0 0 0 0
0 0 0 0
0 0 0 0
---------
您只有一个数组实例,可以不断进行变异并将其多次添加到列表中。 最后,列表包含对处于其最终状态的单个数组的引用。
而不是list.add(grid);
您应该添加grid
的副本(请注意,这里的Arrays.copyOf()
或System.arraycopy()
不够,因为它们执行浅表复制并且您的数组是2维的)。
伊兰是对的。 更新的queens()
:
// if you reached the end of the grid successfully
if (row == GRID_SIZE) {
//print grid - shows correct solution
printGrid(grid);
//creating copy of grid
int[][] copyGrid = new int[GRID_SIZE][GRID_SIZE];
for (int i =0; i < GRID_SIZE; i++) {
copyGrid[i] = grid[i].clone();
}
list.add(copyGrid);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.