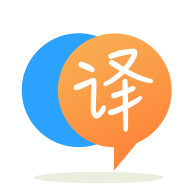
[英]What is wrong in my concatenation function mystrcat(char*, char*, char*)?
[英]What is Wrong with My Concatenation Operation?
我试图实现strcat()
,所以我想到了这段代码。 但是,我不知道这是怎么回事? 这给了我细分错误。
我以为这可能是内存分配混乱? 是吗 不使用malloc()
怎么解决?
#include <stdio.h>
char *strcat(char *s,char *d){
while(*s++ != '\0') ;
while(*s++ = *d++) ;
*s = '\0';
return s;
}
int main(){
char s[20]= "source";
char d[20]= "dest";
printf("%s\n",strcat(s,d));
return 0;
}
我必须Concat的d
提前s
。
s
不会足够长 修理:
...
#define S_STR "source"
char *d= "dest";
char *s= S_STR;
s = malloc(strlen(s) + strlen(d) + 1);
strcpy(s, S_STR);
printf("%s\n",strcat(s,d));
free(s);
return 0;
}
s,d是字符串常量! 你绝对不应该做这样的事情。 有一个像char s [100]这样的大数组,将源复制到它,然后使用串联。 请记住,s应该有空间容纳d的内容!
我修好了它
#include <stdio.h>
#define SIZE 20
char *strocat(char *s,char *d){
char *temp = s;
while(*s++ != '\0');
*--s;
while(*s++ = *d++);
*s = '\0';
return temp;
}
int main(){
char s[SIZE] = "source";
char d[SIZE] = "dest";
printf("%s\n",strocat(s,d));
return 0;
}
您声明的字符串s和d是常量字符串文字,您无法修改它们。 您应该声明两个char数组,并确保要复制到的一个足以容纳另一个char数组。
#include <stdio.h>
char *strcat(char *s,char *d)
{
//Get length of s string
size_t len = strlen(s);
//declare new pointer and assign it the value of s
char *ptr = s;
//move the pointer to the end of the string
ptr += len;
//copy contentes of d string to s string
while( *d != '\0' )
{
*ptr++ = *d++;
}
*ptr = '\0';
//return s
return s;
}
int main()
{
//make sure s array length is big enough to accomodate d string
char s[50] = "source";
char d[] = "dest";
printf("%s\n",strcat(s,d));
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.