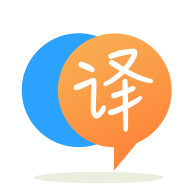
[英]IOS How to retrieve one of the element in xml after calling success and put it into an array to display on tableview
[英]IOS How to parse the xml retrieve the element to put in an array and display in UITableView
- (void)viewDidLoad {
[super viewDidLoad];
NSLog(@"Response recieved");
output= [[NSMutableArray alloc] init];
// NSString *severity = @"Informational";
NSString *soapMessage = @"<?xml version=\"1.0\" encoding=\"utf-8\"?>"
"<soap:Envelope xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\" xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\">"
"<soap:Body>"
" <IncidentGetList xmlns=\"https://www.monitoredsecurity.com/\">"
"<Severity></Severity>"
"<SourceOrganization></SourceOrganization>"
"<DestinationOrganization></DestinationOrganization>"
"<MaxIncidents></MaxIncidents>"
"<SourceIP></SourceIP>"
"<Category></Category>"
"<ExcludeCategory></ExcludeCategory>"
"<StartTimeStampGMT></StartTimeStampGMT>"
"<EndTimeStampGMT></EndTimeStampGMT>"
"<CustomerSeverity></CustomerSeverity>"
"</IncidentGetList>"
"</soap:Body>"
"</soap:Envelope>";
NSURL *url = [NSURL URLWithString:@"https://api.monitoredsecurity.com/SWS/incidents.asmx"];
NSMutableURLRequest *theRequest = [NSMutableURLRequest requestWithURL:url];
NSString *msgLength = [NSString stringWithFormat:@"%d", [soapMessage length]];
[theRequest addValue: @"text/xml; charset=utf-8" forHTTPHeaderField:@"Content-Type"];
[theRequest addValue: @"https://www.monitoredsecurity.com/IncidentGetList" forHTTPHeaderField:@"SOAPAction"];
[theRequest addValue: msgLength forHTTPHeaderField:@"Content-Length"];
[theRequest setHTTPMethod:@"POST"];
[theRequest setHTTPBody: [soapMessage dataUsingEncoding:NSUTF8StringEncoding]];
NSURLConnection *connection = [[NSURLConnection alloc] initWithRequest:theRequest delegate:self];
[connection start];
if(connection)
{
webResponseData = [NSMutableData data] ;
}
else
{
NSLog(@"Connection is NULL");
}
// Menu View
// Uncomment the following line to preserve selection between presentations.
// self.clearsSelectionOnViewWillAppear = NO;
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem;
// Insert Navigation Bar
// [self insertNavBarWithScreenName:SCREEN_INCIDENT];
//
// CGRect frm = btnDownArrow.frame;
// frm.origin.x = 185;
// frm.origin.y = 42;
// [btnDownArrow setFrame:frm];
// [self.navBarView addSubview:btnDownArrow];
//
//
//
// [self addGestureRecognizersToPiece:self.view];
}
- (void)connection:(NSURLConnection *) connection didReceiveResponse:(NSURLResponse *)response
{
NSLog(@"Response recieved");
[self.webResponseData setLength:0];
}
- (void)connection:(NSURLConnection*) connection didReceiveData:(NSData *)data
{
NSLog(@"Data recieved");
// NSString *responseString = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];
[self.webResponseData appendData:data];
// NSLog(responseString);
// [response setText:responseString];
//[status setText:@"Response retrieved async"];
}
-(void)connectionDidFinishLoading:(NSURLConnection *)connection {
NSLog(@"Received %lu Bytes", (unsigned long)[webResponseData length]);
NSString *theXML = [[NSString alloc] initWithBytes:
[webResponseData mutableBytes] length:[webResponseData length] encoding:NSUTF8StringEncoding];
NSLog(@"%@",theXML);
//now parsing the xml
NSData *myData = [theXML dataUsingEncoding:NSUTF8StringEncoding];
NSXMLParser *xmlParser = [[NSXMLParser alloc] initWithData:myData];
//setting delegate of XML parser to self
xmlParser.delegate = self;
[xmlParser parse];
// Run the parser
@try{
BOOL parsingResult = [xmlParser parse];
NSLog(@"parsing result = %hhd",parsingResult);
}
@catch (NSException* exception)
{
UIAlertView* alert = [[UIAlertView alloc]initWithTitle:@"Server Error" message:[exception reason] delegate:self cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
return;
}
}
//Implement the NSXmlParserDelegate methods
-(void) parser:(NSXMLParser *)parser didStartElement:(NSString *)elementName
namespaceURI:(NSString *)namespaceURI qualifiedName:
(NSString *)qName attributes:(NSDictionary *)attributeDict
{
//currentElement = elementName;
currentElement = [elementName copy];
strCaptured = [[NSMutableString alloc] init];
if ([currentElement isEqualToString:@"Severity"]) {
item = [[NSMutableDictionary alloc] init];
NSLog(@"Log Output%@",[item objectForKey:@"Severity"]);
}
currentElement = elementName;
//NSLog(@"current element: ", elementName);
}
- (void)parserDidStartDocument:(NSXMLParser *)parser{
NSLog(@"File found and parsing started");
}
- (void)parser:(NSXMLParser *)parser foundCharacters:(NSString *)string
{
[strCaptured appendString:string];
}
- (void)parser:(NSXMLParser *)parser didEndElement:(NSString *)elementName
namespaceURI:(NSString *)namespaceURI qualifiedName:(NSString *)qName
{
if ([currentElement isEqualToString:@"Severity "]) {
[output addObject:[item copy]];
} else {
[item setObject:strCaptured forKey:elementName];
}
}
- (void)parserDidEndDocument:(NSXMLParser *)parser {
if (errorParsing == NO)
{
NSLog(@"XML processing done!");
} else {
NSLog(@"Error occurred during XML processing");
}
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Row display. Implementers should *always* try to reuse cells by setting each cell's reuseIdentifier and querying for available reusable cells with dequeueReusableCellWithIdentifier:
// Cell gets various attributes set automatically based on table (separators) and data source (accessory views, editing controls)
return [output count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *cellId = @"cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"UITableViewCell"];
if(cell == nil){
cell= [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:cellId];
}
cell.textLabel.text= [output objectAtIndex:indexPath.row];
return cell;
}
在 NSObject 中创建一个文件,然后在解析中使用方法
- (void)parserDidStartDocument:(NSXMLParser *)parser
{
}
- (void) parser: (NSXMLParser *) parser parseErrorOccurred: (NSError *) parseError {
}
- (void) parser: (NSXMLParser *) parser didStartElement: (NSString *) elementName
namespaceURI: (NSString *) namespaceURI qualifiedName: (NSString *) qName
attributes: (NSDictionary *) attributeDict{
}
- (void) parser: (NSXMLParser *) parser didEndElement: (NSString *) elementName namespaceURI: (NSString *) namespaceURI
qualifiedName: (NSString *) qName{
if ([elementName isEqualToString:@"webResponseData"])
{
[communication addObject:[currentElement stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]]];
}
currentElement = nil;
}
- (void) parser: (NSXMLParser *) parser foundCharacters: (NSString *) string {
if(!currentElement)
currentElement = [[NSMutableString alloc] initWithString:string];
else
[currentElement appendString:string];
}
然后在 viewcontroller 中的 connectiondidfinishloading 导入解析器文件并制作文件示例的对象:-
NSString *responseStringCommunication=[[NSString alloc]initWithData:MutableData encoding:NSUTF8StringEncoding];
Parser *parser=[[Parser alloc]init];
NSMutableArray *array=[parser parse:responseStringCommunication];
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.