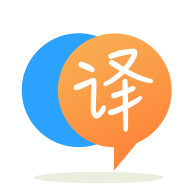
[英]How do I emplement a loop to get the program to ask the user for input again instead of ending the program
[英]How do I use set and get methods to ask and retrieve user input for my program?
我是编码的新手,并参加了Java编码的大学课程。 我正在学习如何编码可一起使用的不同类,并且需要帮助编写使用set和get方法请求用户输入的代码。 我的作业要求制作2个类,即矩形和房屋,它们要计算房屋中4个房间的面积和宽度,然后计算所有房间的总面积。 我创建并运行了两个类,其中House是主要类。 下面是矩形类的代码:
import java.text.NumberFormat;
import java.util.Scanner;
public class Rectangle
{
// the instance variables
private double length, width;
//gets and processes the user input
public void getUserInput()
{
Scanner input = new Scanner(System.in);
System.out.print("Length: ");
length = input.nextInt();
System.out.print("Width: ");
width = input.nextInt();
}
// methods of the rectangle class
public void setLength(double len)
{
length = len;
}
public void setWidth(double w)
{
width = w;
}
public double getLength()
{
return length;
}
public double getWidth()
{
return width;
}
public double getArea()
{
return length * width;
}
}
这是矩形类的代码:
import java.util.Scanner;
import java.text.NumberFormat;
public class House
{
public static void main(String args[])
{
// display a welcome message
System.out.println();
System.out.println("Welcome to the House Total Area Calculator!");
System.out.println();
Scanner user_input = new Scanner(System.in);
String choice = "y";
while (choice.equalsIgnoreCase("y"))
{
/**
creates 4 instances(objects) of the rectangle class.
each instance represents a room in a 4 bedroom house
*/
Rectangle rect1 = new Rectangle();
Rectangle rect2 = new Rectangle();
Rectangle rect3 = new Rectangle();
Rectangle rect4 = new Rectangle();
// get input for length / width of each room from the user
//room 1
System.out.println("Enter length and Width of room 1: ");
rect1.getUserInput();
System.out.println();
//room 2
System.out.println("Enter length and width of room 2: ");
rect2.getUserInput();
System.out.println();
//room 3
System.out.println("Enter length and width of room 3: ");
rect3.getUserInput();
System.out.println();
//room 4
System.out.println("Enter length and width of room 4: ");
rect4.getUserInput();
System.out.println();
double totalArea = rect1.getArea() * rect2.getArea() * rect3.getArea() * rect4.getArea();
System.out.println("The total area of all rooms in the house is: " + totalArea);
// ask if user wants to continue or exit the program
System.out.print("Continue? (y/n): ");
choice = user_input.next();
System.out.println();
}
}
}
程序运行良好,但是我的硬件作业要求使用set方法“接受要存储在长度和宽度字段中的参数”,并使用get方法来“返回存储在长度和宽度字段以及每个区域中的方法房间”。 但是我看到,如果我从矩形类中删除长度和宽度的set和get方法(仅将getArea方法保持原样),程序仍将以相同的方式运行而没有任何问题。 因此,我的问题是如何为set / get方法编写代码以在不使用getUserInput方法的情况下从用户检索数据输入(如果可能的话)?
好吧,您的Rectangle类可以像这样编码
private double length;
private double width;
public double getLength() {
return length;
}
public void setLength(Scanner scan) {
System.out.print("Enter length");
this.length = scan.nextDouble();
}
public double getWidth() {
return width;
}
public void setWidth(Scanner scan) {
System.out.print("Enter width");
this.width = scan.nextDouble();
}
public Double getArea(){
return length * width;
}
而且House类中的main()方法可以这样编码:
System.out.println("Welcome to the House Total Area Calculator!");
System.out.println();
Scanner user_input = new Scanner(System.in);
String choice = "y";
while (choice.equalsIgnoreCase("y"))
{
/**
creates 4 instances(objects) of the rectangle class.
each instance represents a room in a 4 bedroom house
*/
Rectangle r1 = new Rectangle();
Rectangle r2 = new Rectangle();
Rectangle r3 = new Rectangle();
Rectangle r4 = new Rectangle();
// get input for length / width of each room from the user
//room 1
System.out.println("Enter length and Width of room 1: ");
r1.setLength(user_input);
r1.setWidth(user_input);
System.out.println();
//room 2
System.out.println("Enter length and width of room 2: ");
r2.setLength(user_input);
r2.setWidth(user_input);
System.out.println();
//room 3
System.out.println("Enter length and width of room 3: ");
r3.setLength(user_input);
r3.setWidth(user_input);
System.out.println();
//room 4
System.out.println("Enter length and width of room 4: ");
r4.setLength(user_input);
r4.setWidth(user_input);
System.out.println();
double totalArea = r1.getArea() * r2.getArea() * r3.getArea() * r4.getArea();
System.out.println("The total area of all rooms in the house is: " + totalArea);
// ask if user wants to continue or exit the program
System.out.print("Continue? (y/n): ");
choice = user_input.next();
System.out.println();
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.