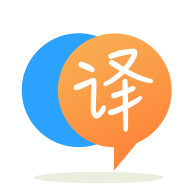
[英]Copy Constructor Issue C++: "0xC0000005: Access violation writing location 0x00000000."
[英]0xC0000005: Access violation reading location 0x00000000. c++ vs2015
我正在使用一个简单的文本解析器创建一个 RPG,并且我在文本解析器中继续遇到访问写入冲突的问题。 到目前为止,这是我的代码:
/*
Author: Michael Norris
Program: Generiquest
Filename: TextParser.cpp
Maintainence Log:
10/28/2015 Created textParser.cpp
Notes:
This text parser is fairly inefficient, but is easier to manage and understand for beginners.
*/
#include <conio.h>
#include <stdio.h>
#include <Windows.h>
#include <time.h>
#include "myheader.h"
#include <iostream>
#include <string.h>
using namespace std;
class Words
{
public:
Words()
{
word verbs[30];//This is an array of all the verbs
strcpy(verbs[0].text, "Items");
strcpy(verbs[1].text, "Stats");
strcpy(verbs[2].text, "Use");
strcpy(verbs[3].text, "Eat");
strcpy(verbs[4].text, "Throw");
strcpy(verbs[5].text, "Drop");
strcpy(verbs[6].text, "Look");
strcpy(verbs[7].text, "Move");
strcpy(verbs[8].text, "Put");
strcpy(verbs[9].text, "Speak");
strcpy(verbs[10].text, "Attack");
strcpy(verbs[11].text, "Go");
strcpy(verbs[12].text, "Climb");
strcpy(verbs[13].text, "Open");
strcpy(verbs[14].text, "Take");
strcpy(verbs[15].text, "Put");
strcpy(verbs[16].text, "Kill");
strcpy(verbs[17].text, "Get");
strcpy(verbs[18].text, "LOL");
//End of verb declarations
for (int ele = 0; ele < 19; ele++)
{
verbs[ele].type = verb;
}
}
};
Words mainWords;
void textParser()
{
char str[51] = "Test String";
char test[50] = "";
char word1[20] = "";
//char * word2;
char word3[20] = "";
char word4[20] = "";
system("cls");
scanf("%50[0-9a-zA-Z ]", &test);
flushall();
strcpy(word3, strtok(test, " ,.-"));
int cray;
for (bool correctI = false; correctI == false;)
{
if (word3 != NULL)
{
strcpy(word1, strtok(NULL, " ,.-"));
cray = strcmp(word1, NULL);//Error thrown here
if (cray != 0)
{
strcpy(word4, word1);
}
}
printf("%s", word3);
printf("%s", word4);
cray = stricmp(word1, "Items");
if (cray = 0)
{
printf("Success!!");
}
else
{
printf("Fail");
}
}
_getch();
}
//TODO: use stricmp()
我在文本解析器功能中遇到了麻烦。
您正在使用类编写 C,而不是 C++。
C++ 通过在它们之上构建薄抽象避免了许多指针问题。
例如,您可以使用std::string
而不是使用char*
C 样式数组。 所以不是不安全
const char* word1 = "Whatever";
cray = stricmp(word1, "Items");
if (cray == 0) {
你会得到
std::string word1 = "Whatever";
if("Items" == word1) {
没有类似的
strcmp(word1, NULL)
因为将字符串与空指针进行比较是没有意义的(并且在 C 中是不允许的)。
您可能想与空字符串进行比较:使用文字""
。
请注意,您在if (cray = 0)
也有错误(与我上面的代码相比)。
此外,无论何时遇到错误,都不应该立即在最近的论坛或 StackOverflow 上发布代码。 相反,您需要在调试器下启动您的应用程序并尝试自己找出问题。 这样你会更好地理解你的代码。
我认为在尝试编写更多 C++ 并使用 [C++] 标记更多问题之前,您可能应该选择一些关于该主题的好书。 这对您和 SO 社区都将更有成效。 所以有一个很棒的帖子:
void textParser()
{
char str[51] = "Test String";
char test[50] = "";
char word1[20] = "";
//char * word2;
char word3[20] = "";
char word4[20] = "";
system("cls");
system("cls");
scanf("%50[0-9a-zA-Z ]", &test);
flushall();
strcpy(word3, strtok(test, " ,.-"));
int cray;
for (bool correctI = false; correctI == false;)
{
if (word3 != NULL)
{
strcpy(word1, strtok(NULL, " ,.-"));
if (word1 != NULL)
{
strcpy(word4, word1);
}
}
printf("%s", word3);
printf("%s", word4);
cray = stricmp(word1, "Items");
if (cray = 0)
{
printf("Success!!");
}
else
{
printf("Fail");
}
}
_getch();
}
仍然在 strcpy(word1, strtok(NULL, " ,.-")); 处抛出 accces 违规错误;
对您(前)TL;DR kod 列表的一些评论
scanf("%50[0-9a-zA-Z ]", &test);
flushall();
strcpy(word3, strtok(test, " ,.-"));
备注:不要使用flushall() 参见http://faq.cprogramming.com/cgi-bin/smartfaq.cgi?answer=1044873249&id=1043284392而是使用读取缓冲区的循环,例如使用fgetc() 或使用替代方法- 见下一条评论。
备注:要更好地控制输入,请使用 fgets 然后使用 sscanf,将其放在单独的函数中
for (bool correctI = false; correctI == false;)
备注:这是不寻常的, do { ... } while (!correctI)
会更清楚。
strcpy(word1, strtok(NULL, " ,.-"));
cray = strcmp(word1, NULL);
if (cray != 0)
{
strcpy(word4,word1);
}
备注:检查 word1 是否为 NULL 改为执行
char* tok = strtok(NULL, " ,.-");
if (tok != NULL)
{
strcpy(word4,tok);
}
word verbs[30];//This is an array of all the verbs
strcpy(verbs[0].text, "Items");
strcpy(verbs[1].text, "Stats");
...
备注:可以写成
word verbs[] = { {"Items", 0}, {"Stats", 1}, ... };
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.