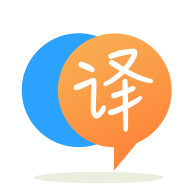
[英]Why assignment operator = doesn't return the value of pointer but the dereference value?
[英]Dereference operator doesn't work (syntax issue?)
因此,我有一个非常单调的清单实现示例。 而且我有一个begin
函数,作为forward_list
的公共成员,该函数返回一个指向列表的第一个(根)元素的指针。
现在,由于它返回指向包含各种成员的_node
对象的指针,据我的理解,必须提供解引用运算符重载,以使_node
知道在取消引用时返回什么。
在解引用运算符定义中,我尝试返回_node
的value
,这似乎很合逻辑,因为begin
返回一个_node
,这意味着解引用begin
会给我_node
后面的value
。 显然不是,正如MSVC编译器告诉我的那样: binary '<<': no operator found which takes a right-hand operand of type '_node<TType>' (or there is no acceptable conversion)
#include <iostream>
#include <cstddef>
//forward declarations
template<class TType> class forward_list;
template<class TType>
class _node
{
private:
TType key;
_node *next;
friend class forward_list<TType>;
public:
TType operator*() { return this->key; } //problem is here
};
template<class TType>
class forward_list
{
private:
_node<TType> *_root;
_node<TType> *_tail;
std::size_t _size;
private:
void _add_node_front(const TType &new_key)
{
_node<TType> *new_node = new _node<TType>{ new_key, this->_root };
if (this->_root == nullptr)
this->_tail = new_node;
this->_root = new_node;
++this->_size;
}
public:
forward_list() : _root(nullptr), _tail(nullptr), _size(0) {}
void push_front(const TType &new_key) { this->_add_node_front(new_key); }
_node<TType> *begin() { return this->_root; }
};
int main()
{
forward_list<int> l;
l.push_front(23);
l.push_front(57);
l.push_front(26); //26 57 23
std::cout << *l.begin(); //expected to print out "26"
}
编辑::感谢乔亚希姆·皮尔博格的建议。 进行以下更改就可以像魅力一样工作:
_node<TType> begin() { return *this->_root; }
您正在返回一个指针:
_node<TType> *begin();
因此您需要两次取消引用:
_node<...>
定义的,而不是为_node<...> *
,它不会被调用而不是简单的取消引用) 也许,更好的办法是更换
TType operator*();
用户定义的转换,将return this->key;
:
operator TType& ();
operator TType const& () const;
并且您不需要第二次取消引用。
问题是您取消了对指针的引用,该指针为您提供了_node<int>
类型的对象,然后您可以再次_node<int>
使用取消引用运算符。
因此,您可以使用两个取消引用运算符来解决您的问题:
**i.begin()
另一个解决方案是您通过值返回节点,而不是返回指向它的指针,这就是迭代器在标准容器中的功能。
您错误的直接原因是begin
返回_node*
,而您的重载operator*
需要一个_node
。
如果要实现符合标准的容器,则begin
操作符应返回一个迭代器 (按值),而不是(指向a的)节点。 这个迭代器应该实现operator*
(以及operator++
和其他一些东西)。 节点类不适合用作迭代器。 您需要一个单独的类。 Google类似“实现我自己的迭代器”。
如果您实现迭代器,请不要向最终用户公开您的节点类。
如果您只需要任何不符合任何条件的列表,最好不要重载任何运算符。 使用getValue
东西。
问题在这条线上:
std::cout << *l.begin(); //expected to print out "26"
您尚未定义operator <<将这些对象之一流式传输到ostream。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.