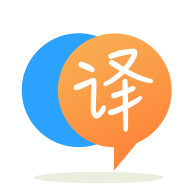
[英]Setting up a RESTful backend mock with Sinon.js for an Angular.js application
[英]Setting up relationships with mongoDB, node and angular.js
我是来自Ruby on Rails的新手,我正在尝试建立这样的数据库: 用户有许多群组和帖子。 每个组都属于一个用户。 每个帖子都属于一个用户和一个或多个组。
我了解这应该如何工作的总体概念,但是,我很难将各个部分放在一起。 我很好奇我应该如何设置数据库,编写api,然后设置控制器。 我列出了到目前为止的内容。 任何指向我正确方向的事情都会很有帮助。
谢谢
用户数据模型
// app/models/user.js
// load the things we need
var mongoose = require('mongoose');
var bcrypt = require('bcrypt-nodejs');
var Schema = mongoose.Schema;
var Post = require('./post');
var Group = require('./groups');
// define the schema for our user model
var userSchema = mongoose.Schema({
local : {
email : String,
password : String,
username : String,
group : {
name: String,
created : {type:Date, default: Date.now},
post: { url: String,
highlighted: String,
comment: String,
image: String,
group: String,
timeStamp: String,
description: String,
title: String,
created: {type:Date, default: Date.now}
}
},
created: {type:Date, default: Date.now}
}
});
userSchema.methods.generateHash = function(password) {
return bcrypt.hashSync(password, bcrypt.genSaltSync(8), null);
};
userSchema.methods.validPassword = function(password) {
return bcrypt.compareSync(password, this.local.password);
};
module.exports = mongoose.model('User', userSchema);
API
...
router.route('/users')
.post(function(req, res) {
var user = new User();
user.local.name = req.body.name;
user.local.email = req.body.email;
user.local.password = req.body.password;
user.local.posts = req.body.post;
user.local.groups = req.body.group;
user.save(function(err) {
if (err)
res.send(err);
res.json({ message: 'user created!' });
});
})
.get(function(req, res) {
User.find(function(err, users) {
if (err)
res.send(err);
res.json(users);
});
});
router.route('/users/:user_id')
// get the post with that id (accessed at GET http://localhost:8080/api/users/:user_id)
.get(function(req, res) {
User.findById(req.params.user_id, function(err, user) {
if (err)
res.send(err);
res.json(user);
});
})
// update the user with this id (accessed at PUT http://localhost:8080/api/users/:user_id)
.put(function(req, res) {
// use our user model to find the user we want
User.findById(req.params.user_id, function(err, user) {
if (err)
res.send(err);
user.name = req.body.name;
user.local.email = req.body.email;
user.local.password = req.body.password;
user.local.posts = req.body.post;
user.local.groups = req.body.group;
// save the user
user.save(function(err) {
if (err)
res.send(err);
res.json({ message: 'user updated!' });
});
});
})
// delete the user with this id (accessed at DELETE http://localhost:8080/api/users/:user_id)
.delete(function(req, res) {
User.remove({
_id: req.params.user_id
}, function(err, user) {
if (err)
res.send(err);
res.json({ message: 'Successfully deleted' });
});
});
...
控制者
$scope.addUser = function() {
//$scope.user.url = parenturl;
console.log($scope.user._id);
$http.user('/api/users', $scope.user).success(function(response){
refreshuser();
//$scope.user = {url: parenturl}
});
};
$scope.remove = function(id) {
console.log(id);
$http.delete('/api/users/' + id).success(function(response) {
//refreshuser();
});
return false;
};
$scope.edit = function(id){
console.log(id);
$http.get('/api/users/' + id).success(function(response) {
$scope.user = response;
});
};
$scope.update = function() {
console.log($scope.user.id);
$http.put('/api/users/' + $scope.user._id, $scope.post).success(function(response) {
refreshUser();
});
};
我认为你做得很好。 当然,这比我刚开始的时候要好得多。 我不确定这里的问题是什么,但是如果您要在MEAN路径上寻找其他资源,首先我建议使用一个框架,这样可以减少开发时间。 还有许多其他选择。
我也读过这本书 ,发现它简明扼要,直达主题。 本书从零开始,最后是在heroku上实时部署应用程序。 该书的作者还管理着一个名为scotch.io的站点,您将在其中找到有关MEAN堆栈的一些不错的教程。
如果您想一般地学习更多的javascript,也可以找到egghead.io 。 他们在那里有免费和付费教程。 有时甚至免费的也不错。
如果您想了解更多有关mongo的信息,请绝对从mongo University上课。它们免费,而且很棒。 到目前为止,我已经参加了4堂课,如果您通过了该课程,您将获得结业证书。 拥有证书肯定会激励我,也可能会激励您。
如果您想了解有关角度本身的更多信息,我强烈推荐Dan Wahlin的 udemy课程。 我从他那儿拿走了两个,他们从字面上照亮了我,使棱角分明变得更有意义。 如果您不想支付全价,我建议您等待几周,直到在美国感恩节。 在那段时间发生了许多在线交易。 我以每笔$ 10的价格参加了这些课程,或者在交易中获得了类似的价格。
我从事PHP开发已有6年以上。 在这一点上,感谢nodejs,我可以肯定JavaScript是未来。 那是我的个人观点,我只提到它是希望它能给您勇气,而且绝对不会引发讨论战争。 希望我能帮上忙。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.