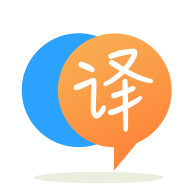
[英]Calculate area of a polygon using longitude and latitude using javascript
[英]How to calculate an area based on the set of Latitude and longitude values using javascript?
如何使用 javascript 根据一组纬度和经度值计算面积?
我有一组从我自己的数据库中提取的纬度和经度值。 它们看起来像这样:
// Define the LatLng coordinates for the polygon's path.
var fizuliCoords = [
{lng:47.1648244,lat:39.6834661},
{lng:47.1653823,lat:39.6906634},
........there are 400-1200 lines...I only list 2
];
var bakuCoords = [
{lng:47.1648244,lat:39.6834661},
{lng:47.1653823,lat:39.6906634},
........there are 400-1200 lines...I only list 2
];
有69个不同的行政区域
我的项目是:
好的,
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no">
<meta charset="utf-8">
<title>ttttt</title>
<style>
html, body {
height: 100%;
margin: 0;
padding: 0;
}
/* #map is the div that places map on the html page*/
#map {
height: 100%;
}
/* end of #map */
</style>
</head>
<body>
<div id="map"></div>
<script>
// This example creates a simple polygon representing the shusha Region.
//the initMapfunction places the map on the page
function initMap() {
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 8,
center: {lat: 40.352310, lng: 47.999462},
mapTypeId: google.maps.MapTypeId.TERRAIN
});
//end oof initMap function
//------------------------------------------------------------------
// Define the LatLng coordinates for the Shusha polygon's path.
var shushaCoords = [
{lng:46.5852069,lat:39.7918524},
{lng:46.5810012,lat:39.789016},
{lng:46.5800569,lat:39.7812995},
{lng:46.5741348,lat:39.7757586},
{lng:46.5720748,lat:39.7722619},
{lng:46.571817,lat:39.763026}
];
// Define the LatLng coordinates for the Khojavend polygon's path.
var khojavendCoords = [
{lng:46.6707802,lat:39.5735368},
{lng:46.6646003,lat:39.5704934},
{lng:46.6628837,lat:39.5675823}
];
// Define the LatLng coordinates for the Zangilan polygon's path.
var xocaliCoords = [
{lng:46.6195822,lat:39.233882},
{lng:46.6161489,lat:39.232918},
{lng:46.6094971,lat:39.2322199},
{lng:46.6076088,lat:39.2318542},
{lng:46.6009998,lat:39.229494}
];
**and so on for 69 more areas then come the polygons**
**// Construct the xocali polygon.**
var xocali = new google.maps.Polygon({
map: map,
paths: xocaliCoords,
strokeColor: '#000000',
strokeOpacity: 0.5,
strokeWeight: 2,
fillColor: '#00CCFF',
fillOpacity: 0.15
});
**//and then 68 more**
// Add a listener for the julfa mouseover/mouseout event.
google.maps.event.addListener(julfa ,'mouseover',function(){
this.setOptions({fillColor: "#00FF00"});
});
**//here I would like to add a popup where the AREA will be calculated**
google.maps.event.addListener(julfa ,'mouseout',function(){
this.setOptions({fillColor: "#00CCFF"});
});
//and then 68 more sets of listeners
}
</script>
<!--end of mapping script-->
<!--the script below is the googleAPI browser script, if i need to display on the server - must change to Server API code.-->
<script async defer src="https://maps.googleapis.com/maps/api/js? key=xxxxxxxxxxxxxxxxx&signed_in=true&callback=initMap"></script>
</body>
</html>
所以我在这里有 3 个问题: 1. 能够构建一个公式,根据每个多边形的 lat lng 值计算面积 2. 在弹出窗口中完成此操作 3. 可以使用外部 .js 文件
Google Maps Javascript API 几何库包含方法computeArea
:
计算区域(路径:数组|MVCArray,半径?:数字)
返回值:数字
返回封闭路径的面积。 计算的面积使用与半径相同的单位。 半径默认为以米为单位的地球半径,在这种情况下,面积以平方米为单位。
我的建议是制作一个子程序,将多边形添加到您的地图中,并带有鼠标悬停/鼠标移出/点击侦听器:
function addPolygon(points, name, bounds, map) {
var path = [];
var polybounds = new google.maps.LatLngBounds();
for (var i = 0; i < points.length; i++) {
path.push(new google.maps.LatLng(points[i].lat, points[i].lng));
bounds.extend(path[path.length - 1]);
polybounds.extend(path[path.length - 1]);
var m = new google.maps.Marker({
position: path[path.length - 1],
// map: map,
title: name + i + " " + path[path.length - 1].toUrlValue(6),
icon: {
url: "https://maps.gstatic.com/intl/en_us/mapfiles/markers2/measle_blue.png",
size: new google.maps.Size(7, 7),
anchor: new google.maps.Point(3.5, 3.5)
}
});
}
var polygon = new google.maps.Polygon({
paths: path,
strokeColor: '#000000',
strokeOpacity: 0.5,
strokeWeight: 2,
fillColor: '#00CCFF',
fillOpacity: 0.15,
map: map,
bounds: polybounds
});
polygons.push(polygon);
google.maps.event.addListener(polygon, 'click', function (evt) {
infowindow.setContent(name + " area: " + google.maps.geometry.spherical.computeArea(polygon.getPath()).toFixed(2) + " square meters");
infowindow.setPosition(evt.latLng);
infowindow.open(map);
});
google.maps.event.addListener(polygon, 'mouseover', function () {
this.setOptions({
fillColor: "#00FF00"
});
});
google.maps.event.addListener(polygon, 'mouseout', function () {
this.setOptions({
fillColor: "#00CCFF"
});
});
}
代码片段:
var infowindow = new google.maps.InfoWindow(); var polygons = []; function initialize() { var map = new google.maps.Map( document.getElementById("map_canvas"), { center: new google.maps.LatLng(37.4419, -122.1419), zoom: 13, mapTypeId: google.maps.MapTypeId.ROADMAP }); var bounds = new google.maps.LatLngBounds(); addPolygon(bakuCoords, "baku", bounds, map); addPolygon(fizuliCoords, "fizuli", bounds, map); map.fitBounds(polygons[0].bounds); } google.maps.event.addDomListener(window, "load", initialize); function addPolygon(points, name, bounds, map) { var path = []; var polybounds = new google.maps.LatLngBounds(); for (var i = 0; i < points.length; i++) { path.push(new google.maps.LatLng(points[i].lat, points[i].lng)); bounds.extend(path[path.length - 1]); polybounds.extend(path[path.length - 1]); } var polygon = new google.maps.Polygon({ paths: path, strokeColor: '#000000', strokeOpacity: 0.5, strokeWeight: 2, fillColor: '#00CCFF', fillOpacity: 0.15, map: map, bounds: polybounds }); polygons.push(polygon); google.maps.event.addListener(polygon, 'click', function(evt) { infowindow.setContent(name + " area: " + google.maps.geometry.spherical.computeArea(polygon.getPath()).toFixed(2) + " square meters"); infowindow.setPosition(evt.latLng); infowindow.open(map); }); google.maps.event.addListener(polygon, 'mouseover', function() { this.setOptions({ fillColor: "#00FF00" }); }); google.maps.event.addListener(polygon, 'mouseout', function() { this.setOptions({ fillColor: "#00CCFF" }); }); } // Define the LatLng coordinates for the polygon's path. var fizuliCoords = [{ lng: 47.1648244, lat: 39.6834661 }, { lng: 47.1653823, lat: 39.6906634 }, { lng: 47.16, lat: 39.68 }, { lng: 47.163, lat: 39.684 }]; var bakuCoords = [{ lng: 47.1648244, lat: 39.6834661 }, { lng: 47.1653823, lat: 39.6906634 }, { lng: 47.165, lat: 39.692 }, { lng: 47.166, lat: 39.692 }];
html, body, #map_canvas { height: 100%; width: 100%; margin: 0px; padding: 0px }
<script src="https://maps.googleapis.com/maps/api/js?libraries=geometry&key=AIzaSyCkUOdZ5y7hMm0yrcCQoCvLwzdM6M8s5qk"></script> <div id="map_canvas"></div>
试试这些...
function GetArea(polygon) { const length = polygon.length; let sum = 0; for (let i = 0; i < length; i += 2) { sum += polygon[i] * polygon[(i + 3) % length] - polygon[i + 1] * polygon[(i + 2) % length]; } console.log(Math.abs(sum) * 0.5); } function latlontocart(latlon) { let latAnchor = latlon[0][0]; let lonAnchor = latlon[0][1]; let x = 0; let y = 0; let R = 6378137; //radius of earth let pos = []; for (let i = 0; i < latlon.length; i++) { let xPos = (latlon[i][1] - lonAnchor) * ConvertToRadian(R) * Math.cos(latAnchor); let yPos = (latlon[i][0] - latAnchor) * ConvertToRadian(R); pos.push(xPos, yPos); } return pos; } function ConvertToRadian(input) { return (input * Math.PI) / 180; } let latlon = [ [9.9847583, 76.578329], [9.984835, 76.578337], [9.984858, 76.578173], [9.984768, 76.578166], ]; GetArea(latlontocart(latlon));
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.