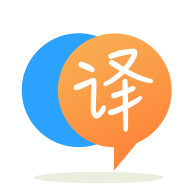
[英]FATAL EXCEPTION: main java.lang.ArrayIndexOutOfBoundsException:
[英]Fatal Exception: java.lang.ArrayIndexOutOfBoundsException
嗨,我正在尝试制作一个用于 gps 检测的应用程序。 它工作正常。但是经过几个时间间隔数组越界发生
这是代码
public class MainActivity extends AppCompatActivity implements GoogleApiClient.ConnectionCallbacks,
GoogleApiClient.OnConnectionFailedListener, LocationListener {
private Button btnShowLocation;
private String TAG = "app";
private GoogleMap mGoogleMap;
private String mLastUpdateTime;
private TextView tvText;
private Marker mapMarker;
private List<Location> loc;
float distance, changeDistance;
private Location mCurrentLocation;
private List<Marker> marker;
double latitude ,longitude;
private static final long INTERVAL = 3000; //3 sec
private static final long FASTEST_INTERVAL = 3000;
private GoogleApiClient mGoogleApiClient;
private LocationRequest mLocationRequest;
private long mTimeStamp;
protected void createLocationRequest() {
mLocationRequest = new LocationRequest();
mLocationRequest.setInterval(INTERVAL);
mLocationRequest.setSmallestDisplacement(10);
mLocationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
mLocationRequest.setFastestInterval(FASTEST_INTERVAL);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Fabric.with(this, new Crashlytics());
setContentView(R.layout.activity_main);
btnShowLocation = (Button) findViewById(R.id.textview1);
marker = new ArrayList<>();
loc = new ArrayList<>();
try {
// Loading map
initilizeMap();
} catch (Exception e) {
e.printStackTrace();
}
tvText = (TextView) findViewById(R.id.text1);
if (!isGooglePlayServicesAvailable()) {
finish();
}
createLocationRequest();
mGoogleApiClient = new GoogleApiClient.Builder(this)
.addApi(LocationServices.API)
.addConnectionCallbacks(this)
.addOnConnectionFailedListener(this)
.build();
updateUI();
btnShowLocation.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
updateUI();
}
});
}
private void initilizeMap() {
mGoogleMap = ((MapFragment) getFragmentManager().findFragmentById(
R.id.map)).getMap();
mGoogleMap.clear();
mGoogleMap.setMapType(GoogleMap.MAP_TYPE_HYBRID);
addMarker(mGoogleMap.getMyLocation());
if (mGoogleMap == null) {
Toast.makeText(getApplicationContext(),
"Sorry! unable to create maps", Toast.LENGTH_SHORT)
.show();
}
}
private void addMarker(Location loc) {
MarkerOptions options = new MarkerOptions();
options.icon(BitmapDescriptorFactory.defaultMarker());
LatLng currentLatLng = new LatLng(loc.getLatitude(), loc.getLongitude());
options.position(currentLatLng);
mapMarker = mGoogleMap.addMarker(options);
mapMarker.setDraggable(true);
mapMarker.showInfoWindow();
marker.add(mapMarker);
Log.d("size marker", marker.size() + " ");
long atTime = mCurrentLocation.getTime();
mLastUpdateTime = DateFormat.getTimeInstance().format(new Date(atTime));
mapMarker.setTitle(mLastUpdateTime + " distance " + distance + "m");
mGoogleMap.moveCamera(CameraUpdateFactory.newLatLngZoom(currentLatLng,
15));
Log.d(TAG, "Zoom done");
}
@Override
public void onStart() {
super.onStart();
Log.d(TAG, "onStart fired ");
mGoogleApiClient.connect();
}
@Override
public void onDestroy() {
super.onDestroy();
Log.d(TAG, "onStop fired ");
mGoogleApiClient.disconnect();
Log.d(TAG, "isConnected : " + mGoogleApiClient.isConnected());
}
private boolean isGooglePlayServicesAvailable() {
int status = GooglePlayServicesUtil.isGooglePlayServicesAvailable(this);
if (ConnectionResult.SUCCESS == status) {
return true;
} else {
GooglePlayServicesUtil.getErrorDialog(status, this, 0).show();
return false;
}
}
@Override
public void onConnected(Bundle bundle) {
Log.d(TAG, "onConnected - isConnected: " + mGoogleApiClient.isConnected());
startLocationUpdates();
}
protected void startLocationUpdates() {
PendingResult<Status> pendingResult = LocationServices.FusedLocationApi.requestLocationUpdates(
mGoogleApiClient, mLocationRequest, this);
Log.d(TAG, "Location update started ");
}
@Override
public void onConnectionSuspended(int i) {
}
@Override
public void onConnectionFailed(ConnectionResult connectionResult) {
Log.d(TAG, "Connection failed: " + connectionResult.toString());
}
@Override
public void onLocationChanged(Location location) {
Log.d(TAG, "Firing onLocationChanged");
mCurrentLocation = location;
if (loc.size() > 0)
if (mCurrentLocation.getTime() <= loc.get(loc.size() - 1).getTime()) return;
if (!mCurrentLocation.hasAccuracy()) {
return;
}
if (mCurrentLocation.getAccuracy() > 50) {
return;
}
loc.add(mCurrentLocation);
addMarker(loc.get(0));
long time = System.currentTimeMillis();
if(time - mTimeStamp < 3 * 1000){
return;
} else {
mTimeStamp = time;
}
Log.d(TAG, loc.size() + " " + marker.size());
// Log.d(TAG, loc.get(loc.size() - 1).getLatitude() + " " + mCurrentLocation.getLatitude());
Log.d("distance", changeDistance + " ");
for (int i = 0; i < loc.size(); i++) {
if (mCurrentLocation != loc.get(i)) {
if (loc.size() == 1 || loc.size() == 0) {
distance = getDistance(loc.get(0).getLatitude(), loc.get(0).getLongitude(), mCurrentLocation.getLatitude(), mCurrentLocation.getLongitude());
} else {
mGoogleMap.addPolyline(new PolylineOptions().geodesic(true)
.add(new LatLng(loc.get(i).getLatitude(), loc.get(i).getLongitude()), new LatLng(loc.get(i + 1).getLatitude(), loc.get(i + 1).getLongitude()))
.width(5)
.color(Color.BLUE));
Log.d("distance1", distance + " i " + i);
distance = getDistance(loc.get(i).getLatitude(), loc.get(i).getLongitude(), loc.get(i + 1).getLatitude(), loc.get(i + 1).getLongitude());
if (marker.size() > 2)
remove(i); // **Error is here**
}
}
}
Log.d("distance new", distance + " i ");
changeDistance = changeDistance + distance;
Log.d("changeDistance ", changeDistance + "");
addMarker(loc.get(loc.size() - 1));
mLastUpdateTime = DateFormat.getTimeInstance().format(new Date());
updateUI();
}
private void remove(int i) {
mapMarker = marker.get(i);
mapMarker.remove();
marker.remove(mapMarker);
}
private void updateUI() {
Log.d(TAG, "UI update initiated");
if (null != mCurrentLocation) {
float distnceInKm = changeDistance / 1000;
Log.d("distance km", distnceInKm + "");
String lat = String.valueOf(mCurrentLocation.getLatitude());
String lng = String.valueOf(mCurrentLocation.getLongitude());
latitude = mCurrentLocation.getLatitude();
longitude = mCurrentLocation.getLongitude();
tvText.setText("At Time: " + mLastUpdateTime + "\n" +
"Latitude: " + lat + "\n" +
"Longitude: " + lng + "\n" +
"Accuracy: " + mCurrentLocation.getAccuracy() + "\n" +
"Provider: " + mCurrentLocation.getProvider() + "\n" +
"Distance " + distnceInKm + " km");
} else {
tvText.setText("location is null ");
Log.d(TAG, "location is null ");
}
}
@Override
public void onResume() {
super.onResume();
if (mGoogleApiClient.isConnected()) {
startLocationUpdates();
Log.d(TAG, "Location update resumed ");
}
}
/* public void showSettingsAlert() {
AlertDialog.Builder alertDialog = new AlertDialog.Builder(this);
// Setting Dialog Title
alertDialog.setTitle("GPS is settings");
// Setting Dialog Message
alertDialog.setMessage("GPS is not enabled. Do you want to go to settings menu?");
// On pressing Settings button
alertDialog.setPositiveButton("Settings", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
Intent intent = new Intent(Settings.ACTION_LOCATION_SOURCE_SETTINGS);
startActivity(intent);
}
});
// on pressing cancel button
alertDialog.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
dialog.cancel();
}
});
// Showing Alert Message
alertDialog.show();
}
*/
public float getDistance(double lat1, double lon1, double lat2, double lon2) {
android.location.Location homeLocation = new android.location.Location("");
homeLocation.setLatitude(lat1);
homeLocation.setLongitude(lon1);
android.location.Location targetLocation = new android.location.Location("");
targetLocation.setLatitude(lat2);
targetLocation.setLongitude(lon2);
return targetLocation.distanceTo(homeLocation);
}
}
移除方法中发生错误,但在长距离跟踪期间出现此问题,例如 6 公里。
谁能解释我为什么我面临这个问题?
错误如此精确,您超出了数组边界,您试图获得列表中不存在的位置。 检查您如何填写、访问、删除和清除您的列表。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.