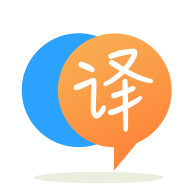
[英]How could I create a static array or list in a class that holds that class' objects c#
[英]How to create a list of the static objects from a class
我通过创建几十个自定义类型的静态对象来设置要测试的数据。 我想要列出这些对象,以便在测试过程中进行动态声明。 这是类和对象:
public class Publication
{
public string Name { get; set; }
public string DropdownText { get; set; }
public string DropdownValue { get; set; }
public string BaseURL { get; set; }
public static Publication MotocrossWeekly = new Publication {
Name = "Motocross Weekly",
DropdownText = "Motocross Weekly",
DropdownValue = "18",
};
public static Publication ExtremeWelding = new Publication {
Name = "Extreme Welding",
DropdownText = "Extreme Welding",
DropdownValue = "6",
};
public static Publication HackersGuide = new Publication {
Name = "Hacker's Guide to Security",
DropdownText = "Hacker's Guide",
DropdownValue = "36",
};
...
public static IList<Publication> Publications = ???;
目标是拥有一个静态的出版物列表,其中将包含Publication类中的所有Publication对象。 这是为了避免每次在系统中添加或删除对象时都必须手动写出列表中的每个对象并编辑列表。
我认为这可以通过反思来完成,但是我找不到我想做的事情的细节。
好吧,要获取公共静态字段的列表,您可以执行以下操作:
typeof(Publication).GetFields(BindingFlags.Static|BindingFlags.Public)
然后循环或使用Linq查找类型为Publication
那些。
但是在类设计中还需要考虑其他一些事项:
Publication
对象替换HackersGuide
。 更好的设计是具有只读属性:
private static Publication _MotocrossWeekly = new Publication {
Name = "Motocross Weekly",
DropdownText = "Motocross Weekly",
DropdownValue = "18",
};
public static MotocrossWeekly {get {return _MotocrossWeekly; } }
// etc.
这样的事情应该起作用:
FieldInfo[] fields = typeof(Publication).GetFields(BindingFlags.Public | BindingFlags.Static)
FieldInfo
应该告诉您您需要了解的有关该字段的所有信息:
https://msdn.microsoft.com/en-us/library/system.reflection.fieldinfo(v=vs.110).aspx
您可以使用反射来做到这一点
public class Publication
{
public string Name { get; set; }
public string DropdownText { get; set; }
public string DropdownValue { get; set; }
public string BaseURL { get; set; }
public static Publication MotocrossWeekly = new Publication
{
Name = "Motocross Weekly",
DropdownText = "Motocross Weekly",
DropdownValue = "18",
};
public static Publication ExtremeWelding = new Publication
{
Name = "Extreme Welding",
DropdownText = "Extreme Welding",
DropdownValue = "6",
};
public static Publication HackersGuide = new Publication
{
Name = "Hacker's Guide to Security",
DropdownText = "Hacker's Guide",
DropdownValue = "36",
};
public static IList<Publication> Publications
{
get
{
return typeof(Publication).GetFields(BindingFlags.Static | BindingFlags.Public)
.Select(f => (Publication)f.GetValue(null))
.ToList();
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.