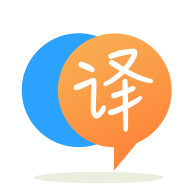
[英]jQuery - OnClick, change background color for table cells always when clicked
[英]Change background color of cells of table using jQuery
我有一张有6行和x列的表。 我需要将单元格的背景色更改为数组中包含的颜色。 颜色索引与应绘制的行数匹配。 我有下一个颜色数组:
var arr = ["lightgreen", "orange", "red"];
还有我的jQuery脚本:
$.each(arr, function(index, val) {
$("tr:eq(" + (index) + ")").css("background-color", val);
});
这段代码仅以不同的颜色绘制了前三行。 我需要这样的输出表:
lightgreen lightgreen lightgreen lightgreen
orange orange orange orange
orange orange orange orange
red red red red
red red red red
red red red red
我的jsfiddle: http : //jsfiddle.net/kvdokq13/
您想每次处理N行 ,而使用$("tr:eq(" + (index) + ")")
仅选择唯一的第N行!
您可以这样实现:
var arr = ["lightgreen", "orange", "red"];
var $rows = $('tr');
var nextRow = 0;
$.each(arr, function(index, val) {
for (var i = 0; i < index + 1; i++) {
$rows.eq(nextRow++).css("background-color", val);
}
});
http://jsfiddle.net/kvdokq13/6/
对于更一般的用途,请注意,应该对其进行增强以适当地照顾到数组长度和行数之间的差异:当前,它假定完全有(1 + 2 + ... + N)
行!
修改了Azim的解决方案...
var arr = ["lightgreen", "orange", "red"];
var tr = $('table tr');
for (var i=0; i<tr.length; i++){
$(tr[i]).css("background-color", arr[i]);
if (i>2){
$(tr[i]).css("background-color", arr[2]);
}
}
http://jsfiddle.net/kvdokq13/5/
仅使用CSS可以提高效率,但是我猜必须使用JQuery吗?
您应该保持编号需要跳过多少行:
var arr = ["lightgreen", "orange", "red"];
var skip = 0;
$.each(arr, function(index, val) {
var _repeat = index+1; // js arrays are zero indexed
for (var i = 0; i < _repeat; i++)
$("tr:eq(" + (i+skip) + ")").css("background-color", val);
skip = skip + _repeat;
});
尽管您已经接受了该问题的答案,但我认为我会使用普通JavaScript而不是库来替代,以防万一可能有用。
请注意,尽管我将发布替代方法(在答案的“主要”部分下方)以显示一个ECMAScript 6功能,“胖箭头”语法和Array.from()
(请参阅下面的参考资料),但我已经使用了它们。如果您还不愿意使用ES6,请选择其他实现)。
也就是说,我自己的解决方法是:
// the colors Array:
var colors = ["lightgreen", "orange", "red"],
// a reference to the <table> element that is
// to be coloured:
table = document.querySelector('table');
// declaring the relevant function to handle the
// colouring:
function colorNRows(arr, table) {
// caching the rows of the table:
var rows = table.rows,
// using Array.from() to convert the HTMLCollection
// into an Array:
clone = Array.from(rows),
// iterating over the array of colours, using
// Array.prototype.map(),
// row: the array-element of the array over which
// we're iterating,
// index: the index of the current array-element
// in that array,
// array: (unused here), the array over which we're
// iterating.
segments = arr.map(function(row, index, array) {
// removing 'index+1' elements of the array from
// the clone Array, starting at index 0 and
// returning the removed row(s) to form a new
// Array:
return clone.splice(0, index + 1);
// iterating over the newly-formed Array,
// using Array.prototype.forEach();
// the automagically-passed array-elements
// are, as above, the array-element (of the new
// array) and the index of the current
// array-element (in that array):
}).forEach(function(rowSegments, index) {
// here we iterate over the array(s) held
// in each array-element, again using the
// automagic variables are the same as above
// relative to the current array.
// we're using the 'fat arrow' syntax to pass
// the array-element (toColor) into the
// the process on the right hand side, thereby
// styling the backgroundColor of each array-element
// (a <tr>) according to the color held in the 'arr'
// array, at the index held in the outer forEach():
rowSegments.forEach(toColor => toColor.style.backgroundColor = arr[index]);
});
}
// calling the function:
colorNRows(colors, table);
var colors = ["lightgreen", "orange", "red"], table = document.querySelector('table'); function colorNRows(arr, table) { var rows = table.rows, clone = Array.from(rows), segments = arr.map(function(row, index, array) { return clone.splice(0, index + 1); }).forEach(function(rowSegments, index) { rowSegments.forEach(toColor => toColor.style.backgroundColor = arr[index]); }); } colorNRows(colors, table);
<table border="4" cellpadding="4" cellspacing="4"> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> <td>5</td> <td>6</td> <td>7</td> <td>8</td> <td>9</td> <td>10</td> </tr> <tr> <td>11</td> <td>12</td> <td>13</td> <td>14</td> <td>15</td> <td>16</td> <td>17</td> <td>18</td> <td>19</td> <td>20</td> </tr> <tr> <td>21</td> <td>22</td> <td>23</td> <td>24</td> <td>25</td> <td>26</td> <td>27</td> <td>28</td> <td>29</td> <td>30</td> </tr> <tr> <td>31</td> <td>32</td> <td>33</td> <td>34</td> <td>35</td> <td>36</td> <td>37</td> <td>38</td> <td>39</td> <td>40</td> </tr> <tr> <td>41</td> <td>42</td> <td>43</td> <td>44</td> <td>45</td> <td>46</td> <td>47</td> <td>48</td> <td>49</td> <td>50</td> </tr> <tr> <td>51</td> <td>52</td> <td>53</td> <td>54</td> <td>55</td> <td>56</td> <td>57</td> <td>58</td> <td>59</td> <td>60</td> </tr> </table>
JS小提琴演示 。
删除ES6功能以与大多数现代浏览器兼容,这给我们留下了以下内容(所有内容都与注释的代码完全相同):
function colorNRows(arr, table) {
var rows = table.rows,
// cloning the rows HTMLCollection, using
// Array.prototype.slice() in conjunction with
// Function.prototype.call(), to allow us to use
// slice() on the Array-like collection:
clone = Array.prototype.slice.call(rows, 0),
segments = arr.map(function(row, index, array) {
return clone.splice(0, index + 1);
}).forEach(function(rowSegments, index) {
// here, rather than a fat arrow function,
// we explicitly use an inner forEach() loop
// performing the same action, but slightly
// more verbose:
rowSegments.forEach(function(toColor) {
toColor.style.backgroundColor = arr[index];
})
});
}
var colors = ["lightgreen", "orange", "red"], table = document.querySelector('table'); function colorNRows(arr, table) { var rows = table.rows, clone = Array.prototype.slice.call(rows, 0), segments = arr.map(function(row, index, array) { return clone.splice(0, index + 1); }).forEach(function(rowSegments, index) { rowSegments.forEach(function(toColor) { toColor.style.backgroundColor = arr[index]; }) }); } colorNRows(colors, table);
<table border="4" cellpadding="4" cellspacing="4"> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> <td>5</td> <td>6</td> <td>7</td> <td>8</td> <td>9</td> <td>10</td> </tr> <tr> <td>11</td> <td>12</td> <td>13</td> <td>14</td> <td>15</td> <td>16</td> <td>17</td> <td>18</td> <td>19</td> <td>20</td> </tr> <tr> <td>21</td> <td>22</td> <td>23</td> <td>24</td> <td>25</td> <td>26</td> <td>27</td> <td>28</td> <td>29</td> <td>30</td> </tr> <tr> <td>31</td> <td>32</td> <td>33</td> <td>34</td> <td>35</td> <td>36</td> <td>37</td> <td>38</td> <td>39</td> <td>40</td> </tr> <tr> <td>41</td> <td>42</td> <td>43</td> <td>44</td> <td>45</td> <td>46</td> <td>47</td> <td>48</td> <td>49</td> <td>50</td> </tr> <tr> <td>51</td> <td>52</td> <td>53</td> <td>54</td> <td>55</td> <td>56</td> <td>57</td> <td>58</td> <td>59</td> <td>60</td> </tr> </table>
JS小提琴演示 。
参考文献:
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.