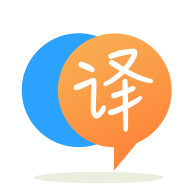
[英]How to get the top 5 elements with the highest occurrence(mode) in a javascript array
[英]Get all elements of array with same (highest) occurrence
我有一个像[1,4,3,1,6,5,1,4,4]
的数组
这里最高元素频率为3,我需要从数组中选择具有3个频率的所有元素,例如上述示例中的[1,4]
。
我已经尝试过了
var count = {},array=[1,4,3,1,6,5,1,4,4],
value;
for (var i = 0; i < array.length; i++) {
value = array[i];
if (value in count) {
count[value]++;
} else {
count[value] = 1;
}
}
console.log(count);
这将输出具有其频率的数组元素,现在我需要所有具有最高频率的元素。
我将按照以下方法解决此问题。
首先,用英语或类似英语(当然是您的母语!)的方式写下您如何解决问题。 写下每个步骤。 从高版本开始,例如:
计算输入中每个元素的频率。
找到最高频率。
等等。 在这一点上,重要的是不要使实施细节陷入困境。 您的解决方案应该适用于几乎所有编程语言。
接下来,通过添加子步骤充实每个步骤。 例如,您可以编写:
找到最高频率。
一种。 假设最高频率为零。
b。 检查每个频率。 如果它高于当前的最高频率,则使其成为当前的最高频率。
通过手动执行算法来测试算法。
接下来,将您编写的内容转换为有时称为伪代码的内容 。 至此,我们的算法开始看起来有点像计算机程序,但是仍然易于理解。 我们现在可以使用变量来表示事物。 例如,我们可以编写“ max_freq←cur_freq”。 我们可以引用数组并编写循环。
最后,将您的伪代码转换为JS。 如果一切顺利,它应该在第一时间工作!
近年来,许多人开始使用JavaScript,甚至没有接触过如何思考算法的知识,甚至是简单的算法。 他们认为,他们需要某种方式能够或者将要神奇地达到他们能够做到的地步,就像在说方言一样,凭空想出JS。 实际上,当遇到问题时,最好的程序员不会立即开始编写array.reduce
。 他们总是经历思考问题的方法的过程(即使只是在头脑中),这是值得我们学习的方法。
如果您不掌握这项技能,那么您每次无法解决问题时都会将剩余的职业生涯花在SO上。
提议包含Array.prototype.reduce()
用于临时对象count
, Object.keys()
用于获取临时对象的密钥, Array.prototype.sort()
方法用于对计数结果进行排序以及Array.prototype.filter()
仅获取计数最多的最高值。
编辑:荣誉@ Xotic750,现在返回原始值。
var array = [1, 4, 3, 1, 6, 5, 1, 4, 4], result = function () { var temp = array.reduce(function (r, a, i) { r[a] = r[a] || { count: 0, value: a }; r[a].count++; return r; }, {}); return Object.keys(temp).sort(function (a, b) { return temp[b].count - temp[a].count; }).filter(function (a, _, aa) { return temp[aa[0]].count === temp[a].count; }).map(function (a) { return temp[a].value; }); }(); document.write('<pre>' + JSON.stringify(result, 0, 4) + '</pre>');
尝试不同的奖励
var array = [1, 4, 3, 1, 6, 5, 1, 4, 4], result = array.reduce(function (r, a) { r.some(function (b, i) { var p = b.indexOf(a); if (~p) { b.splice(p, 1); r[i + 1] = r[i + 1] || []; r[i + 1].push(a); return true; } }) || ( r[1] = r[1] || [], r[1].push(a) ); return r; }, []).pop(); document.write('<pre>' + JSON.stringify(result, 0, 4) + '</pre>');
你可以试试这个
var input = [1,4,3,1,6,5,1,4,4];
var output = {};
for ( var counter = 0; counter < input.length; counter++ )
{
if ( !output[ input[ counter ] ] )
{
output[ input[ counter ] ] = 0;
}
output[ input[ counter ] ]++;
}
var outputArr = [];
for (var key in output)
{
outputArr.push([key, output[key]])
}
outputArr = outputArr.sort(function(a, b) {return b[1] - a[1]})
现在outputArr
初始值是频率最高的
这是小提琴
检查此更新的小提琴 (这将提供您想要的输出)
var input = [1,4,3,1,6,5,1,4,4];
var output = {}; // this object holds the frequency of each value
for ( var counter = 0; counter < input.length; counter++ )
{
if ( !output[ input[ counter ] ] )
{
output[ input[ counter ] ] = 0; //initialized to 0 if value doesn't exists
}
output[ input[ counter ] ]++; //increment the value with each occurence
}
var outputArr = [];
var maxValue = 0;
for (var key in output)
{
if ( output[key] > maxValue )
{
maxValue = output[key]; //find out the max value
}
outputArr.push([key, output[key]])
}
var finalArr = []; //this array holds only those keys whose value is same as the highest value
for ( var counter = 0; counter < outputArr.length; counter++ )
{
if ( outputArr[ counter ][ 1 ] == maxValue )
{
finalArr.push( outputArr[ counter ][ 0 ] )
}
}
console.log( finalArr );
我会做这样的事情。 它未经测试,但已被注释为可帮助您了解我的方法。
// Declare your array
var initial_array = [1,4,3,1,6,5,1,4,4];
// Declare an auxiliar counter
var counter = {};
// Loop over the array
initial_array.forEach(function(item){
// If the elements is already in counter, we increment the repetition counter.
if counter.hasOwnProperty(item){
counter[item] += 1;
// If the element is not in counter, we set the repetitions to one
}else{
counter[item] = 1;
}
});
// counter = {1 : 3, 4 : 3, 3 : 1, 6 : 1, 5 : 1}
// We move the object keys to an array (sorting it's more easy this way)
var sortable = [];
for (var element in counter)
sortable.push([element, counter[element]]);
// sortable = [ [1,3], [4,3], [3,1], [6,1], [5,1] ]
// Sort the list
sortable.sort(function(a, b) {return a[1] - b[1]})
// sortable = [ [1,3], [4,3], [3,1], [6,1], [5,1] ] sorted, in this case both are equals
// The elements in the firsts positions are the elements that you are looking for
// This auxiliar variable will help you to decide the biggest frequency (not the elements with it)
higgest = 0;
// Here you will append the results
results = [];
// You loop over the sorted list starting for the elements with more frequency
sortable.forEach(function(item){
// this condition works because we have sorted the list previously.
if(item[1] >= higgest){
higgest = item[1];
results.push(item[0]);
}
});
我非常喜欢@torazaburo所说的话。
随着ES6越来越多地渗透到我的日常浏览器中,我也成为它的粉丝。 因此,这是使用ES6的解决方案,该解决方案现在可以在我的浏览器中使用。
已加载shims
以修复浏览器浏览器的错误和缺陷,建议在所有环境中使用。
'use strict'; // Your array of values. const array = [1, 4, 3, 1, 6, 5, 1, 4, 4]; // An ES6 Map, for counting the frequencies of your values. // Capable of distinguishing all unique values except `+0` and `-0` // ie SameValueZero (see ES6 specification for explanation) const frequencies = new Map(); // Loop through all the `values` of `array` for (let item of array) { // If item exists in frequencies increment the count or set the count to `1` frequencies.set(item, frequencies.has(item) ? frequencies.get(item) + 1 : 1); } // Array to group the frequencies into list of `values` const groups = []; // Loop through the frequencies for (let item of frequencies) { // The `key` of the `entries` iterator is the value const value = item[0]; // The `value` of the `entries` iterator is the frequency const frequency = item[1]; // If the group exists then append the `value`, // otherwise add a new group containing `value` if (groups[frequency]) { groups[frequency].push(value); } else { groups[frequency] = [value]; } } // The most frequent values are the last item of `groups` const mostFrequent = groups.pop(); document.getElementById('out').textContent = JSON.stringify(mostFrequent); console.log(mostFrequent);
<script src="https://cdnjs.cloudflare.com/ajax/libs/es5-shim/4.4.1/es5-shim.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/json3/3.3.2/json3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/es6-shim/0.34.0/es6-shim.js"></script> <pre id="out"></pre>
您可以像这样找到每个数字的计数发生
var array = [1, 4, 3, 1, 6, 5, 1, 4, 4]; var frequency = array.reduce(function(sum, num) { if (sum[num]) { sum[num] = sum[num] + 1; } else { sum[num] = 1; } return sum; }, {}); console.log(frequency)
<script src="https://getfirebug.com/firebug-lite-debug.js"></script>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.