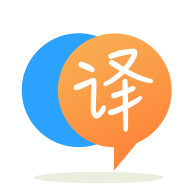
[英]In JQUERY how to find that all ajax calls in a page have been completed?
[英]Without jQuery, how can javascript check that multiple ajax calls have been completed, and once they have, execute a piece of code?
我已经知道如何在jQuery中做到这一点(哦,男孩很容易),但我只是好奇如何在没有jQuery的基本javascript中完成这个。 毕竟,jQuery IS javascript,以及任何可以在一个中完成的东西,都可以在另一个中完成(而不像某些人想象的那样困难!)
请帮助满足我的好奇心! 我想学习潜在的概念〜
一种常见的情况 - 用户执行多个ajax调用,并且只有在完成两个调用时才想执行一段代码。 如何实现这一目标?
这是一个常见场景的简单示例。 这是我到目前为止所尝试过的,(但没有奏效)。 我知道它不起作用的原因是因为在javascript中,一次只能处理一个ajax调用。 那么,什么是这样做没有jQuery的正确方法是什么?
//gets result html code, for a settings div inside a log panel
//this page also inserts some info into my database
//it has to run first and finish first before second ajax call, runs
var xmlhttp;
if (window.XMLHttpRequest) xmlhttp=new XMLHttpRequest();
else xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
xmlhttp.open("POST","/scripts/post_login_panel_actions.php",true);
xmlhttp.setRequestHeader("Content-type","application/x-www-form-urlencoded");
xmlhttp.send("settings_button_activate=1&display_image_url="+encodeURIComponent(settings_display_picture_url));
//gets result html code, for the login panel inside of which, there is a settings div
//this has to run after, so that it can render the new stuff that was added to my database
var xmlhttp2;
if (window.XMLHttpRequest) xmlhttp2=new XMLHttpRequest();
else xmlhttp2=new ActiveXObject("Microsoft.XMLHTTP");
xmlhttp2.open("POST","/scripts/post_login_panel_actions.php",true);
xmlhttp2.setRequestHeader("Content-type","application/x-www-form-urlencoded");
xmlhttp2.send("settings_refresh_login_panel=1");
//after both calls have been completed, the new login panel should be rendered
//and then, the new settings-div should be rendered inside of the new login panel
xmlhttp.onreadystatechange=function() {
if (xmlhttp.readyState==4 && xmlhttp.status==200 && xmlhttp2.readyState==4 && xmlhttp2.status==200) {
document.getElementById("login_panel").innerHTML=xmlhttp2.responseText;
document.getElementById("login_panel_settings").innerHTML=xmlhttp.responseText; } }
编辑后,阅读答案,评论,并尝试编写更多代码:
lleaff的答案是最好的和神圣的狗屎它真的多才多艺,你可以简单地复制粘贴它,它将神奇地工作,对于不同的变量和场景。 我已经接受了它作为正确的答案。
但是这里有一些奖励信息可以帮助那些可能偶然发现这个页面的人......我自己想出了第二种(更粗糙,更愚蠢!)控制两者的方法
这里的问题是,javascript的设计并不是为了让你轻松检查诸如“变量Y变化时的火焰动作X”之类的东西 - 所以有些人可能很难弄清楚如何使用人们告诉你的答案“保持一个全局变量来跟踪已完成的事情”。
您可以启动支票,每次onreadystatechange功能都可以实现...就绪状态已经改变。 我原来实现的问题是我只检查了其中一个ajax调用的就绪状态。
一个更粗略(但易于理解!)的解决方案 ,希望这将为查看此主题的人提供额外的帮助:
//Declare your ajax variables~
var xmlhttp;
if (window.XMLHttpRequest) xmlhttp=new XMLHttpRequest();
else xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
var xmlhttp2;
if (window.XMLHttpRequest) xmlhttp2=new XMLHttpRequest();
else xmlhttp2=new ActiveXObject("Microsoft.XMLHTTP");
//Step 1 (first ajax call) code will begin executing here
xmlhttp.open("POST","/scripts/post_login_panel_actions.php",true);
xmlhttp.setRequestHeader("Content-type","application/x-www-form-urlencoded");
xmlhttp.send("settings_button_activate=1&display_image_url="+encodeURIComponent(document.getElementById("settings_display_picture_url").value));
//Step 2 (second ajax call) this function will be called ... later in the code!
function ajax_call_two() {
xmlhttp2.open("POST","/scripts/post_login_panel_actions.php",true);
xmlhttp2.setRequestHeader("Content-type","application/x-www-form-urlencoded");
xmlhttp2.send("settings_refresh_login_panel=1");
xmlhttp2.onreadystatechange=function() {
if (xmlhttp2.readyState==4 && xmlhttp2.status==200) {
//when Step 2 is finished, Step 3 is called.
stuff_to_do_after_all_ajax_calls_are_finished(); } } }
//Step 3 (stuff_to_do_after_all_ajax_calls_are_finished. literally.) this function will be called ... later in the code!
function stuff_to_do_after_all_ajax_calls_are_finished() {
document.getElementById("login_panel").innerHTML=xmlhttp2.responseText;
document.getElementById("login_panel_settings").innerHTML=xmlhttp.responseText; }
//When Step 1 is finished, Step 2 is called.
xmlhttp.onreadystatechange=function() {
if (xmlhttp.readyState==4 && xmlhttp.status==200) {
ajax_call_two(); } }
只需将传递给xmlhttp.onreadystatechange
的函数命名,并将其分配给每个请求的onreadystatechange
属性。
以下是我将如何概括解决此问题的方法:
function requestsAreComplete(requests) {
return requests.every(function (request) {
return request.readyState == 4;
});
}
function unsuccessfulRequests(requests) {
var unsuccessful = requests.filter(function (request) {
return request.status != 200;
};
return unsuccessful.length ? unsuccessful : null;
}
function onRequestsComplete(requests, callback) {
// Wrap callback into a function that checks for all requests completion
function sharedCallback() {
if (requestsAreComplete(requests)) {
callback(requests, unsuccessfulRequests(requests));
}
}
// Assign the shared callback to each request's `onreadystatechange`
requests.forEach(function (request) {
request.onreadystatechange = sharedCallback;
});
}
而且你会这样使用它:
onRequestsComplete([xmlhttp, xmlhttp2], function(requests, unsuccessful) {
if (unsuccessful) { return; } // Abort if some requests failed
document.getElementById("login_panel").innerHTML=requests[1].responseText;
document.getElementById("login_panel_settings").innerHTML=requests[0].responseText;
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.