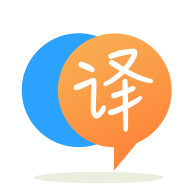
[英]How to move selected rows from one datagridview to another datagridview in c#?
[英]Move selected row from DataGridView to another in Visual C#
我创建了带有两个Datagridviews的表单。 其中一个DataGridViews充满了来自数据库的数据,并且它是源。 使用Button之后,第二个DataGridView应该填充有从源Datagridview中选择的行。
如果填写我的数据表的第一步是这样填充的:
public DataTable loadMatImpTable(String query)
{
myConn.Open();
SQLiteCommand cmd = new SQLiteCommand(query, myConn);
SQLiteDataAdapter sda = new SQLiteDataAdapter();
sda.SelectCommand = cmd;
DataTable dt= new DataTable();
sda.Fill(dt);
return dt;
}
之后,我填充了源DataGridView:
DataTable dt = lt.loadMatImpTable(queryMat);
this.matExpDataGridVW.Rows.Clear();
foreach (DataRow item in dt.Rows)
{
int n = matExpDataGridVW.Rows.Add();
matExpDataGridVW.Rows[n].Cells[0].Value = false;
matExpDataGridVW.Rows[n].Cells[1].Value = item["MaterialID"].ToString();
matExpDataGridVW.Rows[n].Cells[2].Value = item["Name"].ToString();
matExpDataGridVW.Rows[n].Cells[3].Value = item["Preis"];
matExpDataGridVW.Rows[n].Cells[4].Value = item["Anzahl"].ToString();
matExpDataGridVW.Rows[n].Cells[5].Value = item["Datum"].ToString();
}
然后,我按下“ ExportButton”,所有“选择的行”都将复制到第二个DatagRidview。 但是我不会在第二个DataGridview中复制行。 我将移动选定的行。 所以我尝试在foreach循环中删除这些项目:
private void mvImpSelectionBT_Click(object sender, EventArgs e)
{
foreach (DataGridViewRow item in matExpDataGridVW.Rows)
{
if ((bool)item.Cells[0].Value == true)
{
int n = matImpDataGridVW.Rows.Add();
matImpDataGridVW.Rows[n].Cells[0].Value = false;
matImpDataGridVW.Rows[n].Cells[1].Value = item.Cells[1].Value.ToString();
matImpDataGridVW.Rows[n].Cells[2].Value = item.Cells[2].Value.ToString();
matImpDataGridVW.Rows[n].Cells[3].Value = item.Cells[3].Value.ToString();
matImpDataGridVW.Rows[n].Cells[4].Value = item.Cells[4].Value.ToString();
matImpDataGridVW.Rows[n].Cells[5].Value = item.Cells[5].Value.ToString();
}
}
#Delete all Selected rows
foreach (DataGridViewRow item in matExpDataGridVW.SelectedRows)
{
matExpDataGridVW.Rows.Remove(item);
}
}
但是如果我尝试用这种方式删除。 复制所有选定的行,但在源DatGridView中仅删除最后一个选定的行。 删除此选定行的最佳方法是什么?
在复制时创建行列表,然后将该列表用作从源中删除行的基础。
private void mvImpSelectionBT_Click(object sender, EventArgs e)
{
List<DataRow> rowsToDelete = new List<DataRow>();
foreach (DataGridViewRow item in matExpDataGridVW.Rows)
{
if ((bool)item.Cells[0].Value == true)
{
//copy row
int n = matImpDataGridVW.Rows.Add();
matImpDataGridVW.Rows[n].Cells[0].Value = false;
matImpDataGridVW.Rows[n].Cells[1].Value = item.Cells[1].Value.ToString();
matImpDataGridVW.Rows[n].Cells[2].Value = item.Cells[2].Value.ToString();
matImpDataGridVW.Rows[n].Cells[3].Value = item.Cells[3].Value.ToString();
matImpDataGridVW.Rows[n].Cells[4].Value = item.Cells[4].Value.ToString();
matImpDataGridVW.Rows[n].Cells[5].Value = item.Cells[5].Value.ToString();
//add to list
rowsToDelete.Add(item);
}
}
foreach(DataRow row in rowsToDelete)
{
matExpDataGridVW.Rows.Remove(row);
}
}
仅有一个循环的另一种方法是使用带有迭代器的循环,而不是foreach循环。
for (int i = matExpDataGridVW.Rows.Count - 1; i >= 0; i--)
{
if ((bool)matExpDataGridVW.Rows[i].Cells[0].Value == true)
{
//copy row
int n = matImpDataGridVW.Rows.Add();
matImpDataGridVW.Rows[n].Cells[0].Value = false;
matImpDataGridVW.Rows[n].Cells[1].Value = matExpDataGridVW.Rows[i].Cells[1].Value.ToString();
matImpDataGridVW.Rows[n].Cells[2].Value = matExpDataGridVW.Rows[i].Cells[2].Value.ToString();
matImpDataGridVW.Rows[n].Cells[3].Value = matExpDataGridVW.Rows[i].Cells[3].Value.ToString();
matImpDataGridVW.Rows[n].Cells[4].Value = matExpDataGridVW.Rows[i].Cells[4].Value.ToString();
matImpDataGridVW.Rows[n].Cells[5].Value = matExpDataGridVW.Rows[i].Cells[5].Value.ToString();
//delete row
matExpDataGridVW.Rows[i].Delete();
}
matExpDataGridVW.AcceptChanges();
}
如果要“移动”(添加到目标并从源中删除),则将选定的行移至另一个DataGridView
。 尝试通过使用DataSources
移动项目
将数据加载到原始DataGridView
private DataTable _OriginalData;
private DataTable _SelectedData;
private void LoadData()
{
string yourQuery = "SELECT ... FROM ...";
_OriginalData = loadMatImpTable(yourQuery);
//copy structure of original DataTable to the selected table
_SelectedData = _OriginalData.Clone();
//Fill DataGridView
this.matExpDataGridVW.DataSource = _OriginalData;
this.matImpDataGridVW.DataSource = _SelectedData;
//Columns will be generate automatically
//If you want use predefined columns create them through designer or with code
//And set then DataGridView.AutoGenerateColumns = false;
}
然后出口物品会像这样
private void ExportSelectedRows()
{
foreach DataRow row in matExpDataGridVW.SelectedRows
.Cast<DataGridViewRow>()
.Select(r => r.DataBoundItem as DataRowView)
.Where(drv => drv != null)
.Select(drv => drv.Row)
{
_SelectedData.ImportRow(row);
_OriginalData.Rows.Remove(row);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.