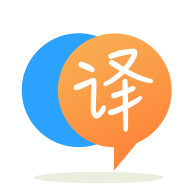
[英]JsonDeserialize set inherited properties also when objectmapper readvalue
[英]ObjectMapper properties set in a generic way?
我正在编写一个类JsonUtils
,它将包含用于序列化和反序列化数据的不同函数。
public class JsonUtils {
private static final ObjectMapper JSON_MAPPER = new ObjectMapper();
public static String toJsonString(Object obj) {
String json = null;
JSON_MAPPER.setPropertyNamingStrategy(new CustomNamingStrategy());
JSON_MAPPER.setSerializationInclusion(Inclusion.NON_NULL);
try {
System.out.print("OBJECT MAPPER:---> JSON STRING:\n" + JSON_MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(obj));
json = JSON_MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(obj);
} catch (JsonGenerationException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return json;
}
public static <T> T toPOJO(String json, Class<T> type){
JSON_MAPPER.setPropertyNamingStrategy(new CustomNameNamingStrategy());
System.out.println("TO POJO: Json string " + json);
try {
return JSON_MAPPER.readValue(json, type);
} catch (JsonParseException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
现在,我希望这些功能可以通用使用。 例如:有人想调用toJsonString
方法,但想使用其他命名策略来转换为json。 或者可能想要向ObjectMapper
添加一些其他属性,例如注册模块。
当前,在函数内部设置了ObjectMapper
属性,因此无法使用新的命名策略或ObjectMapper
的其他属性。
有没有一种方法可以让JsonUtils
每个用户最初为ObjectMapper
设置其自己的属性? 还是编写Utility类的有效且通用的方法?
您可以使用如下形式:
ObjectMapperProperties.java
package example;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
public class ObjectMapperProperties {
private PropertyNamingStrategy propertyNamingStrategy;
private ObjectMapperProperties(final PropertyNamingStrategy propertyNamingStrategy) {
this.propertyNamingStrategy = propertyNamingStrategy;
}
public PropertyNamingStrategy getPropertyNamingStrategy() {
return propertyNamingStrategy;
}
public static class ObjectMapperPropertiesBuilder {
private PropertyNamingStrategy builderPropertyNamingStrategy;
public ObjectMapperPropertiesBuilder() {
}
public ObjectMapperPropertiesBuilder setPropertyNamingStrategy(final PropertyNamingStrategy builderPropertyNamingStrategy) {
this.builderPropertyNamingStrategy = builderPropertyNamingStrategy;
return this;
}
public ObjectMapperProperties build() {
return new ObjectMapperProperties(builderPropertyNamingStrategy);
}
}
}
ObjectMapperFactory.java
package example;
import com.fasterxml.jackson.databind.ObjectMapper;
public class ObjectMapperFactory {
public static ObjectMapper getObjectMapper(final ObjectMapperProperties objectMapperProperties) {
final ObjectMapper result = new ObjectMapper();
result.setPropertyNamingStrategy(objectMapperProperties.getPropertyNamingStrategy());
return result;
}
}
客户类
package example;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
import example.ObjectMapperProperties.ObjectMapperPropertiesBuilder;
public class Client {
public static void main(String[] args) {
ObjectMapperPropertiesBuilder objectMapperPropertiesBuilder = new ObjectMapperPropertiesBuilder();
objectMapperPropertiesBuilder.setPropertyNamingStrategy(PropertyNamingStrategy.CAMEL_CASE_TO_LOWER_CASE_WITH_UNDERSCORES);
ObjectMapperFactory factory = new ObjectMapperFactory();
ObjectMapper objectMapper = ObjectMapperFactory.getObjectMapper(objectMapperPropertiesBuilder.build());
}
}
比您可以根据需要使用设置创建ObjectMapper。 这毫无意义,而且在已创建的实例上两次设置属性很容易出错。
private static final ObjectMapper JSON_MAPPER = new ObjectMapper();
JSON_MAPPER.setSerializationInclusion(Inclusion.NON_NULL);
因此,例如,下次您需要重置此属性时,但是通过某个工厂创建新的ObjectMapper()是无价的,并且不易出错
答案:
不,您将通过ObjectMapperFactory为每个调用创建ObjectMapper的新实例,并仅传递ObjectMapperProperties。
public static String toJsonString(Object obj,final ObjectMapperProperties objectMapperProperties) {
ObjectMapper objectMapper = ObjectMapperFactory.getObjectMapper(objectMapperProperties);
}
如果您不想创建新的ObjectMapper实例并且属性是最终的(意味着您将始终使用相同的属性创建ObjectMapper)而不是方法。
public static String toJsonString(Object obj, ObjectMapper objMapper) {}
第二个问题,请参阅构建器模式
为了更好地使用Factory作为接口进行测试,变化会有所帮助:
ObjectMapperFactory.class
public interface ObjectMapperFactory {
public ObjectMapper getObjectMapper(final ObjectMapperProperties objectMapperProperties) {
}
ObjectMapperFactory的实现
ObjectMapperFactoryImpl.class
package example;
import com.fasterxml.jackson.databind.ObjectMapper;
public class ObjectMapperFactoryImpl implements ObjectMapperFactory {
public ObjectMapper getObjectMapper(final ObjectMapperProperties objectMapperProperties) {
final ObjectMapper result = new ObjectMapper();
result.setPropertyNamingStrategy(objectMapperProperties.getPropertyNamingStrategy());
return result;
}
}
在你班上
public class JsonUtils {
private final ObjectMapperFactory objectMapperFactory;
public JsonUtils(final ObjectMapperFactory objectMapperFactory) {
this.objectMapperFactory = objectMapperFactory;
}
}
但这只是一个变种。 为了您的目的,上面发布的答案就足够了。
您可以使用hashmap并在调用之前从调用者函数中首先放置一些设置值,如下所示
Map <String, String>settings = new HashMap<String, String>();
settings.put("CUSTOM_NAMING_PROPERTY", "CAMEL_CASE");
然后在函数toJsonString
检查该值。
public static String toJsonString(Object obj,Map settings ) {
String json = null;
if(settings.get("CUSTOM_NAMING_PROPERTY")!=null){
//put your settings here.......
}
/////....... contd.....
JSON_MAPPER.setSerializationInclusion(Inclusion.NON_NULL);
try {
System.out.print("OBJECT MAPPER:---> JSON STRING:\n" + JSON_MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(obj));
json = JSON_MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(obj);
} catch (JsonGenerationException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return json;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.