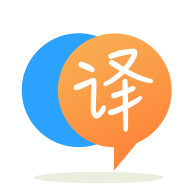
[英]How can I set the DateTimePicker dropdown to select Years or Months only?
[英]How can I set the datetimepicker dropdown to show Months only
为什么你需要这样做? 如果您只想显示月份,那么更简单的方法是在 Combox 中列出月份列表。
但是我在 msdn 上为您找到了一些东西。 看看这里https://social.msdn.microsoft.com/Forums/en-US/7bdca56f-719e-44bf-be6d-a9600dfa8f78/wpf-datepicker-for-months-only?forum=wpf
使用windows消息方法,您可以检测月历控件显示并强制月视图,您可以检测视图更改并关闭月历控件对月度视图更改(在选择月份后)。
实现它的最简单方法是覆盖 DateTimePicker。
public class MonthPicker : DateTimePicker
{
// initialize Format/CustomFormat to display only month and year.
public MonthPicker()
{
Format = DateTimePickerFormat.Custom;
CustomFormat = "MMMM yyyy";
}
// override Format to redefine default value (used by designer)
[DefaultValue(DateTimePickerFormat.Custom)]
public new DateTimePickerFormat Format
{
get => base.Format;
set => base.Format = value;
}
// override CustomFormat to redefine default value (used by designer)
[DefaultValue("MMM yyyy")]
public new string CustomFormat
{
get => base.CustomFormat;
set => base.CustomFormat = value;
}
protected override void WndProc(ref Message m)
{
if (m.Msg == WM_NOFITY)
{
var nmhdr = (NMHDR)Marshal.PtrToStructure(m.LParam, typeof(NMHDR));
switch (nmhdr.code)
{
// detect pop-up display and switch view to month selection
case -950:
{
var cal = SendMessage(Handle, DTM_GETMONTHCAL, IntPtr.Zero, IntPtr.Zero);
SendMessage(cal, MCM_SETCURRENTVIEW, IntPtr.Zero, (IntPtr)1);
break;
}
// detect month selection and close the pop-up
case MCN_VIEWCHANGE:
{
var nmviewchange = (NMVIEWCHANGE)Marshal.PtrToStructure(m.LParam, typeof(NMVIEWCHANGE));
if (nmviewchange.dwOldView == 1 && nmviewchange.dwNewView == 0)
{
SendMessage(Handle, DTM_CLOSEMONTHCAL, IntPtr.Zero, IntPtr.Zero);
}
break;
}
}
}
base.WndProc(ref m);
}
private const int WM_NOFITY = 0x004e;
private const int DTM_CLOSEMONTHCAL = 0x1000 + 13;
private const int DTM_GETMONTHCAL = 0x1000 + 8;
private const int MCM_SETCURRENTVIEW = 0x1000 + 32;
private const int MCN_VIEWCHANGE = -750;
[DllImport("user32.dll")]
public static extern IntPtr SendMessage(IntPtr hWnd, int wMsg, IntPtr wParam, IntPtr lParam);
[StructLayout(LayoutKind.Sequential)]
private struct NMHDR
{
public IntPtr hwndFrom;
public IntPtr idFrom;
public int code;
}
[StructLayout(LayoutKind.Sequential)]
struct NMVIEWCHANGE
{
public NMHDR nmhdr;
public uint dwOldView;
public uint dwNewView;
}
}
试试下面的代码:
DateTime newDateValue = new DateTime(dateTimePicker_month.Value.Year, 1, 1);
dateTimePicker_month.Value = newDateValue;
dateTimePicker_month.Format = DateTimePickerFormat.Custom;
dateTimePicker_month.CustomFormat = "MMM-yyyy";
dateTimePicker_month.ShowUpDown = true;
您必须为二月添加 (1,1) ,其中有 28/29 天以确定所有月份的值。如果您希望查询选择月份。以下是一个示例:
string month = dateTimePicker_month.Value.Month.ToString();
string year = dateTimePicker_month.Value.Year.ToString();
使用以下查询选择月份:
select CAST(date AS DATE) from table where DATEPART(month, date) = '" + month + "' and DATEPART(year,date) = '" + year + "'
尝试使用格式属性:
dateTimePicker.Format = DateTimePickerFormat.Custom;
dateTimePicker.CustomFormat = "MM";
由于在我的情况下不起作用,我修改了 Orance 的答案,将其放入从 DateTimePicker 继承的类中:
protected override void WndProc(ref Message m)
{
if (_MonthSelectStyle)
{
if (m.Msg == 0X204E) // = Win32Messages.WM_REFLECT_NOTIFY
{
var nmhdrI = (NMHDR)(Marshal.PtrToStructure(m.LParam, typeof(NMHDR)));
switch (nmhdrI.code)
{
case -754: // Win32Messages.DTN_DROPDOWN
var cal = SendMessage(m.HWnd, WinApi.Win32Messages.DTM_GETMONTHCAL, IntPtr.Zero, IntPtr.Zero);
SendMessage(cal, WinApi.Win32Messages.MCM_SETCURRENTVIEW, IntPtr.Zero, (IntPtr)1);
break;
case -759: // Win32Messages.DTN_DATETIMECHANGE
WinApi.SendMessage(Handle, WinApi.Win32Messages.DTM_CLOSEMONTHCAL, IntPtr.Zero, IntPtr.Zero);
break;
}
}
}
base.WndProc(ref m);
}
和 VB 等价物:
Protected Overrides Sub WndProc(ByRef m As Message)
If _MonthSelectStyle Then
If m.Msg = &H204E Then ' WM_REFLECT_NOTIFY '&H204E
Dim nmhdrI = CType(Marshal.PtrToStructure(m.LParam, GetType(NMHDR)), NMHDR)
Select Case nmhdrI.code
Case -754 ' Win32Messages.DTN_DROPDOWN '-754
Dim cal = SendMessage(m.HWnd, WinApi.Win32Messages.DTM_GETMONTHCAL, IntPtr.Zero, IntPtr.Zero)
SendMessage(cal, WinApi.Win32Messages.MCM_SETCURRENTVIEW, IntPtr.Zero, CType(1, IntPtr))
Case -759 ' Win32Messages.DTN_DATETIMECHANGE '-759
WinApi.SendMessage(Handle, WinApi.Win32Messages.DTM_CLOSEMONTHCAL, IntPtr.Zero, IntPtr.Zero)
End Select
End If
End If
MyBase.WndProc(m)
End Sub
class "public class MonthPicker: DateTimePicker" 继承自 DateTimePicker,它不是自定义用户控件。
这就是为什么我在我的控制框中看不到 MonthPicker 的原因。 我错过了什么吗? 如何将 MonthPicker.cs 添加到我的代码中,并将其用作自定义控件?
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.