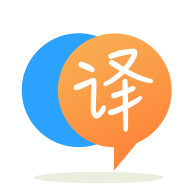
[英]How can I convert a JSON string of objects into an ArrayList using Gson?
[英]how to convert list of objects to json using gson when object has ArrayList attribute
首次启动我的应用程序时,我试图将某些对象列表存储在sharedpreference中,并且在以后使用我的应用程序时,将使用该对象列表。 我的课:
import java.util.ArrayList;
import java.util.List;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicNameValuePair;
public class MyClass {
private int a;
private String b;
private int c;
private ArrayList<NameValuePair> d;
public MyClass(int a, String b, int c) {
super();
this.a = a;
this.b = b;
this.c = c;
}
public MyClass(int a, String b, int c, ArrayList<NameValuePair> d) {
super();
this.a = a;
this.b = b;
this.c = c;
this.d = d;
}
public int getA() {
return a;
}
public void setA(int a) {
this.a = a;
}
public String getB() {
return b;
}
public void setB(String b) {
this.b = b;
}
public int getC() {
return c;
}
public void setC(int c) {
this.c = c;
}
public ArrayList<NameValuePair> getD() {
return d;
}
public void setD(ArrayList<NameValuePair> d) {
this.d = d;
}
public static List<MyClass> getMyObjects() {
// TODO Auto-generated method stub
List<MyClass> myObjects = new ArrayList<MyClass>();
ArrayList<NameValuePair> temp1 = new ArrayList<NameValuePair>();
temp1.add(new BasicNameValuePair("1", "20"));
temp1.add(new BasicNameValuePair("2", "30"));
temp1.add(new BasicNameValuePair("3", "40"));
ArrayList<NameValuePair> temp2 = new ArrayList<NameValuePair>();
temp2.add(new BasicNameValuePair("1", "50"));
temp2.add(new BasicNameValuePair("2", "60"));
temp2.add(new BasicNameValuePair("3", "70"));
ArrayList<NameValuePair> temp3 = new ArrayList<NameValuePair>();
temp3.add(new BasicNameValuePair("1", "50"));
temp3.add(new BasicNameValuePair("2", "60"));
temp3.add(new BasicNameValuePair("3", "70"));
myObjects.add(new MyClass(1, "ABC", 20, temp1));
myObjects.add(new MyClass(2, "DEF", 30, temp2));
myObjects.add(new MyClass(3, "GHI", 40, temp3));
return myObjects;
}
}
我使用以下代码行创建了对象列表并存储在sharedpreference中:
List<MyClass> myObjects = MyClass.getmyObjects();
String myObjectsJSONString = new Gson().toJson(myObjects);
prefsEditor.putString("MYOBJECTS", myObjectsJSONString);
将myObjects写入sharedpreference示例时,JSON字符串按预期形成:
[{"d":[{"name":"1","value":"20"},{"name":"2","value":"30"},{"name":"3","value":"40"}],"b":"ABC","c":20,"a":1},{"d":[{"name":"1","value":"50"},{"name":"2","value":"60"},{"name":"3","value":"70"}],"b":"DEF","c":30,"a":2},{"d":[{"name":"1","value":"50"},{"name":"2","value":"60"},{"name":"3","value":"70"}],"b":"GHI","c":40,"a":3}]
在后续使用中,我尝试使用以下代码行填充列表:
String myobjectsJSONString =appPrefs.getString("MYOBJECTS", null);
Type type = new TypeToken < List <MyClass>> () {}.getType();
List <MyClass> myObjects = new Gson().fromJson(myobjectsJSONString, type);
我可以从没有属性“ d”的sharedpreferences中存储并生成对象列表,该属性为ArrayList<NameValuePair>
类型;
但是,使用“ d”作为属性之一,我无法生成该对象。
显示的错误是:java.lang.RuntimeException:无法为接口org.apache.http.NameValuePair调用no-args构造函数。 使用这种类型的Gson注册InstanceCreator可能会解决此问题
尝试从sharedpreference中存储的json填充对象时引发上述异常
我想我现在明白了:您的MyClass
包含一个字段
private ArrayList<NameValuePair> d;
但是, NameValuePair
实际上是Apache的org.apache.http.NameValuePair
,它是一个接口。 因此,Gson无法创建它的实例-它只能创建一个类的实例。
您可以尝试将其更改为
private ArrayList<BasicNameValuePair> d;
但是该类似乎没有无参数的构造函数,因此它也可能不起作用(尽管此答案表示应该: https : //stackoverflow.com/a/18645370/763935 )。
因此,最安全的选择是编写自己的实现NameValuePair
的类:
public class MyNameValuePair implements NameValuePair {
private final String name;
private final String value;
public MyNameValuePair() {
this.name = null;
this.value = null;
}
@Override
public String getName() {
return this.name;
}
@Override
public String getValue() {
return this.value;
}
}
您可以这样:
{
"Result": [{
"name": "1",
"value": "100"
}, {
"name": "1",
"value": "100"
}, {
"name": "1",
"value": "100"
}]
}
采用 :
Type listType = new TypeToken<List<BeanClass.ResultEntity>>() {
}.getType();
List<BeanClass.ResultEntity> list = new Gson().fromJson(response.toString(), listType);
它肯定会工作...
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.