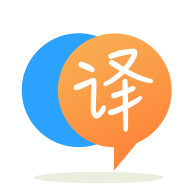
[英]Java guess game. How do I use data validation to check if a number is within a certain range?
[英]How to use Do While loop in “Guess my number ” game?
我正在完成一个名为“猜我的号码”的简单任务,我正在尝试实现我的代码,因此当用户猜测正确的号码时,应用程序将显示“祝贺”,并允许用户选择是否再次播放。 我认为do while循环可以做到,但是我仍然不确定如何实现。 有任何想法吗?。 谢谢高级!
这是我的代码,可以正常运行。
Random random = new Random();
while (true)
{
int randomNumber = random.Next(1, 5);
int counter = 1;
while (true)
{
Console.Write("Guess a number between 1 and 5");
int input = Convert.ToInt32(Console.ReadLine());
if (input < randomNumber)
{
Console.WriteLine("Too low, try again.");
++counter;
continue;
}
else if (input > randomNumber)
{
Console.WriteLine("Too high, try again.");
++counter;
continue;
}
else
{
Console.WriteLine("Congratulations. You guessed the number!");
break;
}
}
}
您可以在最外层循环中添加一个布尔值标志,以在用户说“否”时中断:
Random random = new Random();
while (true)
{
int randomNumber = random.Next(1, 5);
int counter = 1;
bool retry = true;
while (true)
{
Console.Write("Guess a number between 1 and 5");
int input = Convert.ToInt32(Console.ReadLine());
if (input < randomNumber)
{
Console.WriteLine("Too low, try again.");
++counter;
continue;
}
else if (input > randomNumber)
{
Console.WriteLine("Too high, try again.");
++counter;
continue;
}
else
{
Console.WriteLine("Congratulations. You guessed the number!");
Console.WriteLine("Would you like to retry? y/n");
string answer = Console.ReadLine();
if (answer != "y")
{
retry = false;
}
break;
}
}
if (!retry) break;
}
对于do-while版本:
bool retry = true;
Random random = new Random();
do
{
int randomNumber = random.Next(1, 5);
int counter = 1;
while (true)
{
Console.Write("Guess a number between 1 and 5");
int input = Convert.ToInt32(Console.ReadLine());
if (input < randomNumber)
{
Console.WriteLine("Too low, try again.");
++counter;
continue;
}
else if (input > randomNumber)
{
Console.WriteLine("Too high, try again.");
++counter;
continue;
}
else
{
Console.WriteLine("Congratulations. You guessed the number!");
Console.WriteLine("Would you like to retry? y/n");
string answer = Console.ReadLine();
if (answer != "y")
{
retry = false;
}
break;
}
}
} while (retry);
在代码中实现do .. while循环很容易,没有太大区别
Random random = new Random();
do
{
int randomNumber = random.Next(1, 5);
int counter = 1;
do
{
Console.Write("Guess a number between 1 and 5");
int input = Convert.ToInt32(Console.ReadLine());
if (input < randomNumber)
{
Console.WriteLine("Too low, try again.");
++counter;
continue;
}
else if (input > randomNumber)
{
Console.WriteLine("Too high, try again.");
++counter;
continue;
}
else
{
Console.WriteLine("Congratulations. You guessed the number!");
break;
}
}while (true);
}while (true);
希望有帮助...
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.