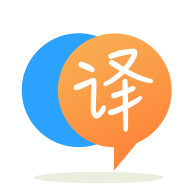
[英]how to hide data table show entries in javascript and jquery?
[英]how to hide the table if there is no data and show the form using jquery or javascript
我以表格格式获取记录并在前端显示数据,但是如果没有数据,我需要隐藏包括表标题在内的总表。这是我的代码。
<table style="width:87%;">
<tr class="spaces">
<th>S.No.</th>
<th style="width:20%;">Rent Earned</th>
<th>House</th>
<th>Address of Property</th>
</tr>
<?php include "rentaldetails.php";
while($row = mysql_fetch_array($result))
{?>
<tr>
<td>
<?php echo $row['house_details_id'];?>
</td>
<td>
<?php echo $row['rental_annual_rent'];?>
</td>
<td>
<?php echo $row['rental_tax_paid'];?>
</td>
<td>
<?php echo $row['rental_town'];?>
</td>
<td style="width:21%;"><a class="button add" onClick="document.location.href='income_tax.php'">Edit Details</a></td>
<td style="width:21%;"><a class="buttons delete" href="deleterental.php?id=<?php echo $row['house_details_id'];?>" onclick="return confirm('Are you sure to delete');" class="table-icon delete">Delete Property</a></td>
<td></td>
</tr>
<?php
}
?>
</table>
<form method="POST" action="details.php">
<th>Address Line</th>
<td><input type="text" name="address" value="" /></td>
<th>Town/City</th>
<td><input type="text" name="city" value="" /></td>
<button type="submit" class = "medium" style="background-color: #a9014b;float:left;">Save</button>
如果数据库中没有数据,我需要隐藏这些总计表,并应显示表格。
您可以使用if else条件显示和隐藏总表,如下所示:
<?php
if(mysqli_num_rows($result) > 0){ ?>
<table style="width:87%;">
<tr class="spaces">
<th>S.No.</th>
<th style="width:20%;">Rent Earned</th>
<th>House</th>
<th>Address of Property</th>
</tr>
<?php include "rentaldetails.php";
while($row = mysql_fetch_array($result))
{?>
<tr>
<td>
<?php echo $row['house_details_id'];?>
</td>
<td>
<?php echo $row['rental_annual_rent'];?>
</td>
<td>
<?php echo $row['rental_tax_paid'];?>
</td>
<td>
<?php echo $row['rental_town'];?>
</td>
<td style="width:21%;"><a class="button add" onClick="document.location.href='income_tax.php'">Edit Details</a></td>
<td style="width:21%;"><a class="buttons delete" href="deleterental.php?id=<?php echo $row['house_details_id'];?>" onclick="return confirm('Are you sure to delete');" class="table-icon delete">Delete Property</a></td>
<td></td>
</tr>
<?php } ?>
</table>
<?php } ?>
您可以使用if else
语句执行此操作。
if(!empty(mysql_fetch_array($result))){
//Here your datatable
}else{
//echo 'No Data';
}
<?php include "rentaldetails.php";
if(mysql_num_rows($result)== 0){
$dispNone = "display:none";
}else{
$dispNone = "display:block";
}
?>
<table style="width:87%;" style="<?=$dispNone?>">
<tr class="spaces">
<th>S.No.</th>
<th style="width:20%;">Rent Earned</th>
<th>House</th>
<th>Address of Property</th>
</tr>
<?php include "rentaldetails.php";
while($row = mysql_fetch_array($result))
{?>
<tr>
<td>
<?php echo $row['house_details_id'];?>
</td>
<td>
<?php echo $row['rental_annual_rent'];?>
</td>
<td>
<?php echo $row['rental_tax_paid'];?>
</td>
<td>
<?php echo $row['rental_town'];?>
</td>
<td style="width:21%;"><a class="button add" onClick="document.location.href='income_tax.php'">Edit Details</a></td>
<td style="width:21%;"><a class="buttons delete" href="deleterental.php?id=<?php echo $row['house_details_id'];?>" onclick="return confirm('Are you sure to delete');" class="table-icon delete">Delete Property</a></td>
<td></td>
</tr>
<?php
}
?>
</table>
编辑:
PHP:
if(mysql_num_rows($result)== 0){
$dispNone = "display:none";
$dispForm = "display:block";
}else{
$dispNone = "display:block";
$dispForm = "display:none";
}
?>
HTML:
<form style="width:87%;" style="<?=$dispForm?>">
</form>
编辑2:
<table style="width:87%;<?=$dispNone?>">
<form style="width:87%;<?=$dispForm?>">
Try this:
<?php
include "rentaldetails.php";
if(!empty(mysql_fetch_array($result))){?>
<table style="width:87%;">
<tr class="spaces" >
<th>S.No.</th>
<th style="width:20%;">Rent Earned</th>
<th>House</th>
<th>Address of Property</th>
</tr>
<?php
while($row = mysql_fetch_array($result))
{?>
<tr>
<td><?php echo $row['house_details_id'];?></td>
<td><?php echo $row['rental_annual_rent'];?></td>
<td><?php echo $row['rental_tax_paid'];?></td>
<td><?php echo $row['rental_town'];?></td>
<td style="width:21%;"><a class="button add" onClick="document.location.href='income_tax.php'">Edit Details</a></td>
<td style="width:21%;"><a class="buttons delete" href="deleterental.php?id=<?php echo $row['house_details_id'];?>" onclick="return confirm('Are you sure to delete');" class="table-icon delete" >Delete Property</a></td>
<td></td>
</tr>
<?php
}
?>
</table>
<?php } ?>
jQuery解决方案:
$(document).ready(function(){ if ($("#mytable td").length == 0){ $("#mytable").hide(); } });
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <table id="mytable" style="width:87%;"> <tr class="spaces"> <th>S.No.</th> <th style="width:20%;">Rent Earned</th> <th>House</th> <th>Address of Property</th> </tr> </table>
您可以使用mysql_num_rows()
隐藏<table>
,它将在获取记录之前检查no的行:
<?php
if(mysql_num_rows($result) > 0){
?>
<table style="width:87%;">
<tr class="spaces">
<th>S.No.</th>
<th style="width:20%;">Rent Earned</th>
<th>House</th>
<th>Address of Property</th>
</tr>
<?php include "rentaldetails.php";
while($row = mysql_fetch_array($result))
{?>
<tr>
<td>
<?php echo $row['house_details_id'];?>
</td>
<td>
<?php echo $row['rental_annual_rent'];?>
</td>
<td>
<?php echo $row['rental_tax_paid'];?>
</td>
<td>
<?php echo $row['rental_town'];?>
</td>
<td style="width:21%;"><a class="button add" onClick="document.location.href='income_tax.php'">Edit Details</a></td>
<td style="width:21%;"><a class="buttons delete" href="deleterental.php?id=<?php echo $row['house_details_id'];?>" onclick="return confirm('Are you sure to delete');" class="table-icon delete">Delete Property</a></td>
<td></td>
</tr>
<?php
}
?>
</table>
<?
}
?>
边注:
请使用mysqli_*
或PDO
扩展名,因为mysql_*
已弃用并在PHP 7中关闭。
如果您需要隐藏整个表,则可以在HTML table标记中使用此PHP代码,如果查询不返回任何结果,则它应该隐藏整个表。
<?php echo (mysql_num_rows($result)>0)?'visible':'hidden'; ?>
因此,基本上,隐藏表的整个代码将如下所示:
<table <?php echo (mysql_num_rows($result)>0)?'visible':'hidden'; ?> style="width:87%;"> <tr class="spaces"> <th>S.No.</th> <th style="width:20%;">Rent Earned</th> <th>House</th> <th>Address of Property</th> </tr> <?php include "rentaldetails.php"; while($row = mysql_fetch_array($result)) {?> <tr> <td> <?php echo $row['house_details_id'];?> </td> <td> <?php echo $row['rental_annual_rent'];?> </td> <td> <?php echo $row['rental_tax_paid'];?> </td> <td> <?php echo $row['rental_town'];?> </td> <td style="width:21%;"><a class="button add" onClick="document.location.href='income_tax.php'">Edit Details</a></td> <td style="width:21%;"><a class="buttons delete" href="deleterental.php?id=<?php echo $row['house_details_id'];?>" onclick="return confirm('Are you sure to delete');" class="table-icon delete">Delete Property</a></td> <td></td> </tr> <?php } ?> </table> <form method="POST" action="details.php"> <th>Address Line</th> <td><input type="text" name="address" value="" /></td> <th>Town/City</th> <td><input type="text" name="city" value="" /></td> <button type="submit" class = "medium" style="background-color: #a9014b;float:left;">Save</button>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.