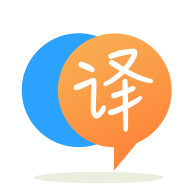
[英]How to call angular2 component method from outside and have reference to this?
[英]Angular2 - how to call component function from outside the app
我正在使用具有回调的 javascript 对象。 一旦回调被触发,我想在 Angular2 组件中调用一个函数。
示例 HTML 文件。
var run = new Hello('callbackfunction');
function callbackfunction(){
// how to call the function **runThisFunctionFromOutside**
}
<script>
System.config({
transpiler: 'typescript',
typescriptOptions: { emitDecoratorMetadata: true },
packages: {'js/app': {defaultExtension: 'ts'}}
});
System.import('js/app/main')
.then(null, console.error.bind(console));
</script>
我的App.component.ts
import {Component NgZone} from 'angular2/core';
import {GameButtonsComponent} from './buttons/game-buttons.component';
@Component({
selector: 'my-app',
template: ' blblb'
})
export class AppComponent {
constructor(private _ngZone: NgZone){}
ngOnInit(){
calledFromOutside() {
this._ngZone.run(() => {
this.runThisFunctionFromOutside();
});
}
}
runThisFunctionFromOutside(){
console.log("run");
}
我如何调用App.component.ts内的函数 runThisFunctionFromOutside
我基本上遵循了这个答案,但我不希望我的“外部”代码了解有关 NgZone 的任何信息。 这是 app.component.ts:
import {Component, NgZone, OnInit, OnDestroy} from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: 'app.component.html'
})
export class AppComponent implements OnInit, OnDestroy {
constructor(private ngZone: NgZone) {}
ngOnInit() {
window.my = window.my || {};
window.my.namespace = window.my.namespace || {};
window.my.namespace.publicFunc = this.publicFunc.bind(this);
}
ngOnDestroy() {
window.my.namespace.publicFunc = null;
}
publicFunc() {
this.ngZone.run(() => this.privateFunc());
}
privateFunc() {
// do private stuff
}
}
我还必须为 TypeScript 添加一个定义来扩展 window 对象。 我把它放在typings.d.ts中:
interface Window { my: any; }
从控制台调用该函数现在非常简单:
my.namespace.publicFunc()
当组件被构造时,让它将自己分配给一个全局变量。 然后你可以从那里引用它并调用方法。 不要忘记使用zone.run(() => { ... })
以便 Angular 收到有关所需更改检测运行的通知。
function callbackfunction(){
// window['angularComponentRef'] might not yet be set here though
window['angularComponent'].zone.run(() => {
runThisFunctionFromOutside();
});
}
constructor(private _ngZone: NgZone){
window['angularComponentRef'] = {component: this, zone: _ngZone};
}
ngOnDestroy() {
window.angularComponent = null;
}
在浏览器控制台中,您必须从<topframe>
切换到plunkerPreviewTarget....
因为 Plunker 在iFrame
执行代码。 然后运行
window['angularComponentRef'].zone.run(() => {window['angularComponentRef'].component.callFromOutside('1');})
或者
window.angularComponentRef.zone.run(() => {window.angularComponentRef.componentFn('2');})
另一种方法
将是在 Angular 之外调度事件并在 Angular 中收听它们,就像在Angular 2 中解释的那样- typescript 函数与外部 js 库的通信
Plunker 示例 2 (来自评论)
下面是一个解决方案。
function callbackfunction(){
window.angularComponent.runThisFunctionFromOutside();
}
<script>
System.config({
transpiler: 'typescript',
typescriptOptions: { emitDecoratorMetadata: true },
packages: {'js/app': {defaultExtension: 'ts'}}
});
System.import('js/app/main')
.then(null, console.error.bind(console));
</script>
我的 App.component.ts
import {Component NgZone} from 'angular2/core';
import {GameButtonsComponent} from './buttons/game-buttons.component';
@Component({
selector: 'my-app',
template: ' blblb'
})
export class AppComponent {
constructor(private _ngZone: NgZone){
window.angularComponent = {runThisFunctionFromOutside: this.runThisFunctionFromOutside, zone: _ngZone};
}
runThisFunctionFromOutside(){
console.log("run");
}
}
另一种不使用全局变量的方法是使用传递控制对象并将其属性绑定到要公开的变量和方法。
export class MyComponentToControlFromOutside implements OnChanges {
@Input() // object to bind to internal methods
control: {
openDialog,
closeDialog
};
ngOnChanges() {
if (this.control) {
// bind control methods to internal methods
this.control.openDialog = this.internalOpenDialog.bind(this);
this.control.closeDialog = this.internalCloseDialog;
}
}
internalOpenDialog(): Observable<boolean> {
// ...
}
internalCloseDialog(result: boolean) {
// ...
}
}
export class MyHostComponent {
controlObject= {};
}
<my-component-to-control [control]="controlObject"></my-component-to-control>
<a (click)="controlObject.open()">Call open method</a>
我在使用fullCalendar库的回调 'eventClick' 时遇到了类似的情况,它的回调从 angular zone 外部返回,导致我的应用程序具有部分和不可靠的效果。 我能够将区域方法和对组件的闭包引用结合起来,如下所示,以引发输出事件。 一旦我开始在 zone.run() 方法内执行事件,该事件及其影响将再次可预测并被角度变化检测检测到。 希望这可以帮助某人。
constructor(public zone: NgZone) { // code removed for clarity
}
ngOnInit() {
this.configureCalendar();
}
private configureCalendar() {
// FullCalendar settings
this.uiConfig = {
calendar: { // code removed for clarity
}
};
this.uiConfig.calendar.eventClick = this.onEventClick();
}
private onEventClick() {
const vm = this;
return function (event, element, view) {
vm.zone.run(() => {
vm.onSequenceSelected.emit(event.sequenceSource);
});
return false;
};
}
只是添加到@Dave Kennedy :
从控制台调用该函数现在非常简单:
my.namespace.publicFunc()
1)如果我们尝试从不同的域访问我们组件的公共方法,您将陷入 CORS 问题(跨域问题,如果服务器和客户端代码驻留在同一台机器上,则可以解决)。
2)如果您要使用 javascript 从服务器调用此方法,则必须使用window.opener.my.namespace.publicFunc()
而不是window.my.namespace.publicFunc():
window.opener.my.namespace.publicFunc();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.