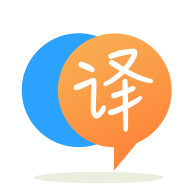
[英]ASP.NET Core MediatR error: Register your handlers with the container
[英]MediatR with ASP.NET Core DI
我正在使用新的 ASP.NET 内核,目前正在创建一个 API,我想从 JavaScript 前端调用它。
我想使用调解器模式来减少耦合,我从 Jimmy Bogard 找到了 Library MediatR 。
我的问题在于使用 DI 中的构建将其连接起来,我尝试查看示例,但看不到它如何绑定到启动 class 中的 ConfigureServices 方法中。
有人有任何见解吗?
更新:我得到了它的工作,从我的 ConfigureService 方法:
services.AddScoped<SingleInstanceFactory>(p => t => p.GetRequiredService(t));
services.Scan(scan => scan
.FromAssembliesOf(typeof(IMediator), typeof(MyHandler.Handler))
.AddClasses()
.AsImplementedInterfaces());
截至 2016 年 7 月,MediatR 的作者 Jimmy Bogard 发布了一个包,用于注册 MediatR 和 Handlers,以及 ASP.Net Core DI 服务(实际上是接口IServiceCollection
,在Microsoft.Extensions.DependencyInjection
实现,而不是仅限在 ASP.Net Core 中使用)。
MediatR.Extensions.Microsoft.DependencyInjection
可以在此处找到介绍该软件包及其功能的博客文章
直接从(非常短的)博客文章中复制的注册示例:
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddMediatR(typeof(Startup));
}
此包执行多项功能以启用 MediatR,包括所需的 Handler 程序集扫描:
您可以传入处理程序所在的程序集,也可以从这些处理程序所在的程序集中传入 Type 对象。 该扩展将 IMediator 接口添加到您的服务、所有处理程序和正确的委托工厂以加载处理程序。 然后在您的控制器中,您可以只使用 IMediator 依赖项:
public class HomeController : Controller
{
private readonly IMediator _mediator;
public HomeController(IMediator mediator)
{
_mediator = mediator;
}
public IActionResult Index()
{
var pong = _mediator.Send(new Ping {Value = "Ping"});
return View(pong);
}
}
我让它工作了,我的代码:
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped<SingleInstanceFactory>(p => t => p.GetRequiredService(t));
services.AddScoped<MultiInstanceFactory>(p => t => p.GetRequiredServices(t));
services.Scan(scan => scan
.FromAssembliesOf(typeof(IMediator), typeof(MyHandlerOne.Handler))
.FromAssembliesOf(typeof(IMediator), typeof(MyHandlerTwo.Handler))
.AddClasses()
.AsImplementedInterfaces());
}
我有一个实现 MultiInstanceFactory 需要的 GetRequiredService 的类:
public static class GetServices
{
public static IEnumerable<object> GetRequiredServices(this IServiceProvider provider, Type serviceType)
{
return (IEnumerable<object>)provider.GetRequiredService(typeof(IEnumerable<>).MakeGenericType(serviceType));
}
}
根据 MediatR 文档注册 MediatR 服务和所有处理程序,您应该以这种方式使用 AddMediatR 方法:
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddMediatR(typeof(Startup));
}
这很容易,很舒服,但是如果您想更换其中一个处理程序怎么办? 然后你应该找到 oldhandler.cs 并从中删除接口,这样一个以上的实现就不会有 ID 冲突。
为避免这种情况,我的建议是手动注册每个处理程序
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddMediatR(typeof(Mediator)); //registering MediatR and all required dependencies
//registering handlers
services.AddScoped<IRequestHandler<CreateProductCommand, int>,CreateProductCommandHandler>();
services.AddScoped<IRequestHandler<DeleteProductCommand, int>,DeleteProductCommandHandler>();
}
此解决方案允许您为同一命令执行多个处理程序实现,并控制使用女巫实现
https://dotnetcoretutorials.com/有一个很好的教程。 这是正确安装和配置 MediatR 的示例代码。
安装 MediatR
我们需要做的第一件事是安装 MediatR nuget 包。 所以从你的包管理器控制台运行:
安装包 MediatR
我们还需要安装一个包,允许我们使用 .NET Core 中的内置 IOC 容器来发挥我们的优势(我们很快就会看到更多内容)。 所以还要安装以下包:
安装包 MediatR.Extensions.Microsoft.DependencyInjection
最后我们打开我们的 startup.cs 文件。 在我们的 ConfigureServices 方法中,我们需要添加一个调用来注册 MediatR 的所有依赖项。
public void ConfigureServices(IServiceCollection services)
{
services.AddMediatR(Assembly.GetExecutingAssembly());
//Other injected services.
}
这是链接: https : //dotnetcoretutorials.com/2019/04/30/the-mediator-pattern-part-3-mediatr-library/
我希望这有帮助。
我为 ASP.NET Core RC2创建了一个DI 助手,您可以将其添加到您的启动中。 它为您提供基于基本约定的映射,因此如果您有一个类,例如:
MyClass : IMyClass
它将在 IOC 容器中映射IMyClass
,使其可用于注入。
我还添加了 MediatR 的映射。
要使用它只需将该类添加到您的项目中,然后在您的 startup.cs 类中将您需要的行添加到ConfigureServices()
方法中:
public void ConfigureServices(IServiceCollection services)
{
//Other Code here......
var ioc = new PearIoc(services);
//ioc.AddTransient<IEmailSender, AuthMessageSender>();
//ioc.AddTransient<ISmsSender, AuthMessageSender>();
ioc.WithStandardConvention();
ioc.WithMediatR();
ioc.RunConfigurations();
}
我添加了AddTransient()
方法只是为了方便(您也可以只使用services.AddTransient()
),但它也公开了IServiceCollection
以防您需要用它做更多事情。
您也可以像我使用.WithMediatR()
扩展一样扩展它并编写自己的自定义映射。
注册所有命令的另一种方法
public void ConfigureServices(IServiceCollection services)
{
services.AddMediatR(Assembly.GetAssembly(typeof(YouClass)));
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.