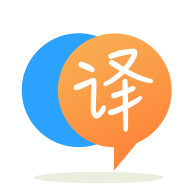
[英]Elegant way to sort three integers in javascript from highest to lowest, then compare relative distance between them
[英]Use Javascript to alert highest and lowest integers from user input
JS的新手,试图完成一项任务,要求用户输入年龄并使用Javascript执行以下任务:
我已经获得了第一名,但是我不知道从第二个开始。 我已经将输入中的数字记录到了控制台,因为我将从那里进行图像比较。 我已经开始尝试勾勒出alertAge()函数,但不知道从哪里获取。
最好的方法是什么?
document.getElementById("myButton").addEventListener("click", checkAge); function checkAge() { var ages = document.getElementById("age").value; if (isNaN(ages)) { alert("Value must be a number. Try again!"); } else { console.log(ages); } } function alertAge() { var highest; var lowest; if (highest === undefined) { // This is the first age we're seeing highest = age; lowest = age; } else { if (age > highest) highest = age; // This is the new highest age if (age < lowest) lowest = age; // This is the new lowest age } }
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width"> <title>JS Bin</title> </head> <body> <label for="age">Enter your age:</label> <input id="age" /> <button id="myButton" type="submit">Go</button> </body> </html>
JS Bin:
对于#1,您可以使用<input type="number">
代替签入JS(如果它是数字)。
对于#2,您需要将值存储在函数之外。 阅读有关Javascript上下文的更多信息 。 要在函数外部使用变量,请执行以下操作:
var highest,lowest;
function alertAge() {...};
更精确的解释:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JS Bin</title>
</head>
<body>
<label for="age">Enter your age:</label>
<input type="number" id="age" />
<button onClick="checkAge()">Go</button>
<script>
var highest = null;
var lowest = null;
function alertAge(age) {
if (!highest) {
highest = lowest = age;
} else {
if (age > highest) {
highest = age; // This is the new highest age
alert('new highest', age);
}
if (age < lowest) {
lowest = age; // This is the new lowest age
alert("new lowest", age);
}
}
}
function checkAge() {
var ages = document.getElementById("age").value;
if (ages) alertAge(ages);
}
</script>
</body>
</html>
<input type="number">
仅限于数字。 只要单击该元素, onClick="checkAge()"
调用checkAge()(不必是按钮)。
highest = lowest = age;
将最低设置为“年龄”,将最高设置为最低(即“年龄”)。 少写,您将需要精力:)
alert('my text')
是典型的javascript弹出窗口“ alert”。
if (ages)
条件,以确保我们不会将怪异的东西发送给alertAge(); highest
变量和lowest
变量使它们具有“某种”性能,即使在函数完成时,也可以使用它们。 如果在函数内声明变量,则在函数执行后该变量将不复存在。 由于您要保存函数的最高年龄或最低年龄,因此需要将其关闭。
尝试这个。
var alertAge = function() {
var highest;
var lowest;
return function(age){
if (highest === undefined) { // This is the first age we're seeing
highest = age;
lowest = age;
} else {
if (age > highest)
highest = age; // This is the new highest age
// alert here
if (age < lowest)
lowest = age;
// alert here
}
};
}();
那么你也能
<script>
var highest = null;
var lowest = null;
function checkAge() {
var ages = document.getElementById("age").value;
if (isNaN(ages)) {
alert("Value must be a number. Try again!");
}
else {
alertAge(ages);
alert("highest="+highest+"\nlowest="+lowest);
console.log(ages);
}
}
function alertAge(age) {
if (highest === null) { // This is the first age we're seeing
highest = age;
lowest = age;
} else {
if (age > highest)
highest = age; // This is the new highest age
if (age < lowest)
lowest = age; // This is the new lowest age
}
}
</script>
<button id="myButton" onclick="checkAge();">Go</button>
我希望这能解决您的问题。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.