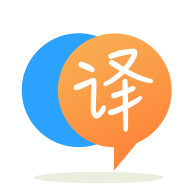
[英]How to make template rvalue reference parameter ONLY bind to rvalue reference?
[英]How to distiguish between an rvalue and rvalue reference in a function parameter
当传递参数时,我想在函数参数中区分这两种情况,如下所示:
int rvalue();
int&& rvalue_ref();
f(rvalue());
f(rvalue_ref());
但是,当我尝试使用这样的转发引用时:
int rvalue()
{
return 1;
}
int&& rvalue_ref(int i)
{
return std::move(i);
}
template<class T>
void f(T&& x)
{
if (std::is_rvalue_reference<T>())
{
std::cout << "Rvalue reference" << std::endl;
}
else if (std::is_lvalue_reference<T>())
{
std::cout << "Lvalue reference" << std::endl;
}
else
{
std::cout << "Not a reference" << std::endl;
}
}
int main()
{
f(rvalue()); // Should print "Not a reference"
f(rvalue_ref(1)); // Should print "Rvalue reference"
}
它打印出两个案例的“不是参考”。 有没有办法在C ++中区分这两种情况?
我不知道如何仅使用函数参数来完成此操作。 xvalue和prvalue之间的区别可能在函数调用中丢失。
但是在调用函数之前,您可以使用在参数上调用decltype
的宏来实现。 下面是一个使用相关信息作为第二个参数调用函数的示例。 我借用了这个帖子的代码。
#include <iostream>
int rvalue()
{
return 1;
}
int&& rvalue_ref(int &&i) // Modified signature to avoid return reference to local variable (ty. user657267)
{
return std::move(i);
}
template<typename T>
struct value_category {
// Or can be an integral or enum value
static constexpr auto value = "prvalue";
};
template<typename T>
struct value_category<T&> {
static constexpr auto value = "lvalue";
};
template<typename T>
struct value_category<T&&> {
static constexpr auto value = "xvalue";
};
// Double parens for ensuring we inspect an expression,
// not an entity
#define VALUE_CATEGORY(expr) value_category<decltype((expr))>::value
#define f(X) f_(X, VALUE_CATEGORY(X))
template<class T>
void f_(T&& x, char const *s)
{
std::cout << s << '\n';
}
int main()
{
f(rvalue()); // Should print "Not a reference"
f(rvalue_ref(1)); // Should print "Rvalue reference"
int j; f(j);
}
输出:
prvalue
xvalue
lvalue
当然,你可以简单地修改字符串以适应,或用枚举等替换它们。
您的代码说“不是引用”的原因是您将T
传递给std::std::is_lvalue_reference<>
和std::std::is_rvalue_reference<>
。 您应该使用decltype()来获取其原始类型。
template<class T>
void f( T&& x )
{
if ( std::is_lvalue_reference< decltype( x ) >() )
{
std::cout << "Lvalue reference" << std::endl;
}
else if ( std::is_rvalue_reference < decltype( x ) >() )
{
std::cout << "Rvalue reference" << std::endl;
}
else
{
std::cout << "Not a reference" << std::endl;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.