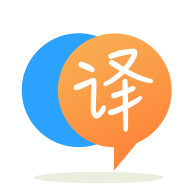
[英]Java: How to find all “entry pairs with maximum value” from a Hashtable
[英]How to check if java hashtable contains key-value pairs from another hashtable
是否有任何内置的java方法来检查一个哈希表是否包含另一个哈希表中存在的所有键值对?
例如:
h1={{0,1},{1,4},{2,5},{3,6}}
h2={{0,1},{2,5}}
在这种情况下, h1
确实包含来自h2
的键值对。
Hashtable
上没有允许您直接检查此方法的方法,但您可以在每个Hashtable
上使用entrySet()方法来获取Set
其所有键值对。
然后你可以使用containsAll()来查看其中一个是否是另一个的子集,因为它
如果此set包含指定collection的所有元素,则返回true。 如果指定的集合也是集合,则此方法如果是此集合的子集,则返回true。
例如
// h1={{0,1},{1,4},{2,5},{3,6}}
Hashtable<Integer, Integer> h1 = new Hashtable<>();
h1.put(0, 1);
h1.put(1, 4);
h1.put(2, 5);
h1.put(3, 6);
// h2={{0,1},{2,5}}
Hashtable<Integer, Integer> h2 = new Hashtable<>();
h2.put(0, 1);
h2.put(2, 5);
Set<Entry<Integer, Integer>> e1 = h1.entrySet();
Set<Entry<Integer, Integer>> e2 = h2.entrySet();
System.out.println(e2.containsAll(e1)); // false
System.out.println(e1.containsAll(e2)); // true
这是一个小示例程序,用于演示如何匹配2个不同映射的键或键和值:
public class HashMapMatch {
public boolean hasMatchingKey(String key, Map m1, Map m2){
return m1.containsKey(key) && m2.containsKey(key);
}
public boolean hasMatchingKeyAndValue(String key, Map m1, Map m2){
if(hasMatchingKey(key, m1, m2)){
return m1.get(key).equals(m2.get(key));
}
return false;
}
public static void main(String[] args) {
HashMapMatch matcher = new HashMapMatch();
Map<String, Integer> map1 = new HashMap<>();
map1.put("One",1);
map1.put("Two",22);
map1.put("Three",3);
Map<String, Integer> map2 = new HashMap<>();
map2.put("One",1);
map2.put("Two",2);
map2.put("Four",4);
System.out.println("Has matching key? :" + matcher.hasMatchingKey("One", map1, map2));
System.out.println("Has matching key? :" + matcher.hasMatchingKey("Two", map1, map2));
System.out.println("Has matching key? :" + matcher.hasMatchingKey("Three", map1, map2));
System.out.println("Has matching key value: :" + matcher.hasMatchingKeyAndValue("One", map1,map2));
System.out.println("Has matching key value: :" + matcher.hasMatchingKeyAndValue("Two", map1,map2));
}
}
产生输出:
Has matching key? :true
Has matching key? :true
Has matching key? :false
Has matching key value: :true
Has matching key value: :false
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.