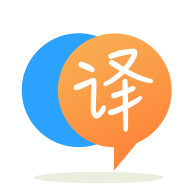
[英]Retrieve the returned value from sql server stored procedure using java
[英]Cannot retrieve the value I desired to Select using Stored Procedure
我试图找到一个记录。 这让我可以选择使用存储过程在我的数据库中查找现有记录。 当我尝试搜索现有数据时,它没有给我想要的值。 当我点击搜索按钮时,它不会将值打印到文本字段。
代码
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
String searchSection = Section_SearchSection_Textfield.getText();
String searchSection_Name = Section_SectionName_TextField.getText();
int sectionID = 0;
if (searchSection.isEmpty())
{
JOptionPane.showMessageDialog(null, "Please fill up this fields");
}
else
try (Connection myConn = DBUtil.connect())
{
try (CallableStatement myFirstCs = myConn.prepareCall("{call getSECTION_NAME(?,?)}"))
{
myFirstCs.setInt(1, sectionID);// I set the ID for Primary Key
myFirstCs.registerOutParameter(2, Types.VARCHAR);
myFirstCs.setString(2, searchSection_Name);
boolean hasresults = myFirstCs.execute();
if (hasresults)
{
try (ResultSet myRs = myFirstCs.getResultSet())
{
int resultsCounter = 0;
while (myRs.next())
{
sectionID = myRs.getInt("SECTION_ID");
String sectionName = myRs.getString(2);
Section_SectionName_TextField.setText(sectionName);//Set the value of text
Section_SectionName_TextField.setEnabled(true);//Set to enable
resultsCounter++;
}//end of while
}//end of if
}//end of resultset
}//end of callablestatement
}//end of connection
catch (SQLException e)
{
DBUtil.processException(e);
}
}
存储过程
CREATE PROCEDURE getSECTION_NAME(IN ID INT, OUT NAME VARCHAR(50))
SELECT * FROM allsections_list WHERE SECTION_ID = ID AND SECTION_NAME = NAME
桌子
CREATE TABLE
(
SECTION_ID INT PRIMARY KEY AUTO_INCREMENT,
SECTION_NAME VARCHAR(50) NOT NULL
)
任何帮助,将不胜感激! 谢谢!
更新! 根据我读到的存储过程可以返回一个结果集。 我想检索 OUT 参数的值。
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
String searchSection = Section_SearchSection_Textfield.getText();
String searchSection_Name = Section_SectionName_TextField.getText();
if (searchSection.isEmpty())
{
JOptionPane.showMessageDialog(null, "Please fill up this fields");
}
else
try (Connection myConn = DBUtil.connect();
CallableStatement myFirstCs = myConn.prepareCall("{call getSECTION_NAME(?,?)}"))
{
myFirstCs.setInt(1, sectionID);// I set the ID for Primary Key
myFirstCs.registerOutParameter(2, Types.VARCHAR);
boolean hasresults = myFirstCs.execute();
if (hasresults)
{
try (ResultSet myRs = myFirstCs.getResultSet())
{
while (myRs.next())
{
sectionID = myRs.getInt("SECTION_ID");
System.out.print(sectionID);
}//end of while
}//end of resultset
}//end of if
String sectionName = myFirstCs.getString(2);
Section_SectionName_TextField.setText(sectionName);//Set the value of text
Section_SectionName_TextField.setEnabled(true);//Set to enable
System.out.print(sectionName);
}//end of connection
catch (SQLException e)
{
DBUtil.processException(e);
}
}
我删除了String sectionName = myRs.getString(2); Section_SectionName_TextField.setText(sectionName); Section_SectionName_TextField.setEnabled(true);
String sectionName = myRs.getString(2); Section_SectionName_TextField.setText(sectionName); Section_SectionName_TextField.setEnabled(true);
从结果集块中取出并将其放入可调用语句块中。 当我运行程序时。 唯一的变化是文本字段被启用并向我打印一个“空”值。
第二次更新! 我想返回 OUT 参数的值我不应该使用结果集来检索它。 所以我根据@Gord Thompson 使用了 Callable Statement 参数和存储过程的 OUT 参数。
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
String searchSection = Section_SearchSection_Textfield.getText();
String searchSection_Name = Section_SectionName_TextField.getText();
if (searchSection.isEmpty())
{
JOptionPane.showMessageDialog(null, "Please fill up this fields");
}
else
try (Connection myConn = DBUtil.connect();
CallableStatement myFirstCs = myConn.prepareCall("{call getSECTION_NAME(?,?)}"))
{
myFirstCs.setInt(1, 2);// I set the ID for Primary Key
myFirstCs.registerOutParameter(2, Types.VARCHAR);
myFirstCs.execute();
String sectionName = myFirstCs.getString(2); // retrieve value from OUT parameter
Section_SectionName_TextField.setText(sectionName);//Set the value of text
Section_SectionName_TextField.setEnabled(true);//Set to enable
System.out.println(sectionName);
}//end of connection
catch (SQLException e)
{
DBUtil.processException(e);
}
}
它仍然给我一个空值,我不知道为什么我得到这个值。
对我的 GUI 的唯一更改是启用了文本字段,并且它没有在以下文本字段中打印我想要的值。 :(
感谢您的回复。 随意发表评论。
如果您想要通过存储过程的 OUT 参数返回的值,则不使用 ResultSet,而是使用与存储过程的 OUT 参数关联的 CallableStatement 参数。 例如,对于测试表
CREATE TABLE `allsections_list` (
`SECTION_ID` int(11) NOT NULL,
`SECTION_NAME` varchar(50) DEFAULT NULL,
PRIMARY KEY (`SECTION_ID`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8
包含样本数据
SECTION_ID SECTION_NAME
---------- ---------------
1 one_section
2 another_section
和存储过程
CREATE PROCEDURE `getSECTION_NAME`(IN myID INT, OUT myName VARCHAR(50))
BEGIN
SELECT SECTION_NAME INTO myName FROM allsections_list WHERE SECTION_ID = myID;
END
那么下面的Java代码
try (CallableStatement myFirstCs = conn.prepareCall("{call getSECTION_NAME(?,?)}")) {
myFirstCs.setInt(1, 2); // set IN parameter "myID" to value 2
myFirstCs.registerOutParameter(2, Types.VARCHAR);
myFirstCs.execute();
String sectionName = myFirstCs.getString(2); // get value from OUT parameter "myName"
System.out.println(sectionName);
}
印刷
another_section
您应该使用delimiter
将分隔delimiter
更改为不同于“;”的内容。 创建过程时:
delimiter //
CREATE PROCEDURE getSECTION_NAME(IN ID INT, OUT NAME VARCHAR(50))
BEGIN
SELECT SECTION_NAME INTO NAME FROM allsections_list WHERE SECTION_ID = ID;
END
//
delimiter ;
第一个delimiter
语句将分隔delimiter
设置为“//”。 这样,“;” 在您的存储过程中,代码不再被解释为分隔符。 然后您的CREATE PROCEDURE
语句正确地以“//”结尾。 之后,第二个delimiter
语句将定界符改回“;”。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.