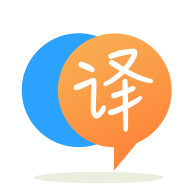
[英]Spring RestController - HTTP Status 400 The request sent by the client was syntactically incorrect
[英]HTTP Status 400 The request sent by the client was syntactically incorrect in Spring
我创建了一个MVC结构来为患者保存新记录,并且不断收到404错误。 这是我的代码,
Patient.java
public class Patient {
private int patient_ID;
private String name;
private String gender;
private int age;
private Date dob;
public Patient(){
}
public Patient(int patient_ID, String name, String gender, int age, Date dob) {
this.patient_ID = patient_ID;
this.name = name;
this.gender = gender;
this.age = age;
this.dob = dob;
}
public int getPatient_ID() {
return patient_ID;
}
public void setPatient_ID(int patient_ID) {
this.patient_ID = patient_ID;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Date getDob() {
return dob;
}
public void setDob(Date dob) {
this.dob = dob;
}
}
控制器类
@Controller
public class PatientController {
@RequestMapping(value="/newPatient",method=RequestMethod.GET)
public ModelAndView newPatient(ModelAndView model) { Patient newpatient = new Patient();
model.addObject("patient", newpatient);
model.setViewName("PatientForm");
System.out.println("sending to patient form");
return model;
}
@RequestMapping(value = "/savePatient", method = RequestMethod.POST)
public ModelAndView savePatient(ModelAndView model,@ModelAttribute Patient patient) {
PatientDaoImplementation patientDaoImpl = new PatientDaoImplementation();
patientDaoImpl.saveOrUpdate(patient);
List<Patient> listPatient = patientDaoImpl.patientList();
model.addObject("listPatient",listPatient);
model.setViewName("home");
return model;
}
}
道实现类
public class PatientDaoImplementation {
private DataSource dataSource;
private static JdbcTemplate jdbcTemplate;
public void setDataSource(DataSource dataSource) {
System.out.println("datasource"+dataSource);
this.dataSource = dataSource;
this.setJdbcTemplate(dataSource);
}
public void setJdbcTemplate(DataSource ds) {
this.jdbcTemplate= new JdbcTemplate(ds);
}
public PatientDaoImplementation(){
}
//get all records in Patient
public List<Patient> patientList() {
String sql ="SELECT * from patient";
List<Patient> listPatient = jdbcTemplate.query(sql, new RowMapper<Patient>(){
public Patient mapRow(ResultSet rs, int arg1) throws SQLException {
Patient patient = new Patient();
patient.setPatient_ID(rs.getInt("Patient_ID"));
patient.setName(rs.getString("Name"));
patient.setAge(rs.getInt("Age"));
patient.setGender(rs.getString("Gender"));
patient.setDob(rs.getDate("DOB"));
return patient;
}
});
return listPatient;
}
public void saveOrUpdate(Patient patient) {
//insert
String sql = "INSERT INTO patient (Patient_ID, Name, Gender, Age,DOB)" + " VALUES (?, ?, ?, ?,?)";
jdbcTemplate.update(sql, patient.getPatient_ID(),patient.getName(),patient.getGender(),patient.getAge(),patient.getDob());
}
}
调度程序servlet
<context:component-scan base-package="com.csc.*" />
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/" />
<property name="suffix" value=".jsp" />
</bean>
<bean id="dataSource"
class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/hospital" />
<property name="username" value="root" />
<property name="password" value="bgowda" />
</bean>
<bean id="patientDaoImpl" class="com.csc.bg.daoimpl.PatientDaoImplementation">
<property name="dataSource" ref="dataSource" />
</bean>
PatientForm.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>New Patient</title>
</head>
<body>
<div align="center">
<h1>New Patient</h1>
<form:form action="save" method="post" modelAttribute="patient" >
<table>
<form:hidden path="patient_ID"/>
<tr>
<td>Name:</td>
<td><form:input path="name" /></td>
</tr>
<tr>
<td>Gender:</td>
<td><form:input path="gender" /></td>
</tr>
<tr>
<td>Age:</td>
<td><form:input path="age" /></td>
</tr>
<tr>
<td>DOB:</td>
<td><form:input path="dob" /></td>
</tr>
<tr>
<td colspan="2" align="center"><input type="submit" value="Save"></td>
</tr>
</table>
</form:form>
</div>
</body>
</html>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>Archetype Created Web Application</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>Dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/Dispatcher-servlet.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
</web-app>
我可以获取列表,但是当我尝试从PatientForm.jsp保存患者详细信息时,表单未将其提交给控制器,并给出400状态错误
当数据发送到控制器时,所有数据都以字符串形式发送。 因此,dob字段也已作为String发送。 当在Model Patient中复制数据时,dob字段是日期类型。
默认情况下,Spring最初没有配置为从字符串类型自动转换为日期类型。 Spring不知道这是一个日期,它将其视为一个字符串。因此,复制到dob字段的数据失败。
您必须在控制器中将String绑定到Date转换器 ,以便在将数据复制到Patient时可以将String dob转换为Date 。
为此,将以下内容添加到您的控制器PatientController中 :
@InitBinder
public void initBinder(WebDataBinder binder) {
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy");
sdf.setLenient(true);
binder.registerCustomEditor(Date.class, new CustomDateEditor(sdf, true));
}
嗯,那个日期字段(dob)是可疑的,您的框架如何知道如何在没有以下内容的情况下进行强制转换:
@DateTimeFormat(pattern = "yyyy-MM-dd")
尝试将此action="save"
为action="savePatient"
。 由于在控制器类中没有名为“ save
操作。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.