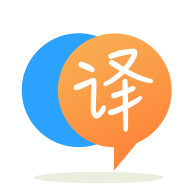
[英]Error parsing data org.json.JSONException: Value <!— of type java.lang.String cannot be converted to JSONObject
[英]How to debug “json parsing error: Value true at error of type java.lang.Boolean cannot be converted to JSONObject”
我正在制作具有gcm集成的android应用,用于群组聊天和消息广播。 但是当我执行它时,应用程序显示错误:
json解析错误:错误类型为java.lang.Boolean时,值true不能转换为JSONObject
LoginActivity.java
:
public class LoginActivity extends AppCompatActivity {
private String TAG = LoginActivity.class.getSimpleName();
private EditText inputName, inputEmail;
private TextInputLayout inputLayoutName, inputLayoutEmail;
private Button btnEnter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/**
* Check for login session. It user is already logged in
* redirect him to main activity
* */
if (MyApplication.getInstance().getPrefManager().getUser() != null) {
startActivity(new Intent(this, MainActivity.class));
finish();
}
setContentView(R.layout.activity_login);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
inputLayoutName = (TextInputLayout) findViewById(R.id.input_layout_name);
inputLayoutEmail = (TextInputLayout) findViewById(R.id.input_layout_email);
inputName = (EditText) findViewById(R.id.input_name);
inputEmail = (EditText) findViewById(R.id.input_email);
btnEnter = (Button) findViewById(R.id.btn_enter);
inputName.addTextChangedListener(new MyTextWatcher(inputName));
inputEmail.addTextChangedListener(new MyTextWatcher(inputEmail));
btnEnter.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
login();
}
});
}
/**
* logging in user. Will make http post request with name, email
* as parameters
*/
private void login() {
if (!validateName()) {
return;
}
if (!validateEmail()) {
return;
}
final String name = inputName.getText().toString();
final String email = inputEmail.getText().toString();
StringRequest strReq = new StringRequest(Request.Method.POST,
EndPoints.LOGIN, new Response.Listener<String>() {
@Override
public void onResponse(String response) {
Log.e(TAG, "response: " + response);
try {
JSONObject obj = new JSONObject(response);
// check for error flag
if (obj.getBoolean("error") == false) {
// user successfully logged in
JSONObject userObj = obj.getJSONObject("user");
User user = new User(userObj.getString("user_id"),
userObj.getString("name"),
userObj.getString("email"));
// storing user in shared preferences
MyApplication.getInstance().getPrefManager().storeUser(user);
// start main activity
startActivity(new Intent(getApplicationContext(), MainActivity.class));
finish();
} else {
// login error - simply toast the message
Toast.makeText(getApplicationContext(), "" + obj.getJSONObject("error").getString("message"), Toast.LENGTH_LONG).show();
}
} catch (JSONException e) {
Log.e(TAG, "json parsing error: " + e.getMessage());
Toast.makeText(getApplicationContext(), "Json parse error: " + e.getMessage(), Toast.LENGTH_SHORT).show();
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
NetworkResponse networkResponse = error.networkResponse;
Log.e(TAG, "Volley error: " + error.getMessage() + ", code: " + networkResponse);
Toast.makeText(getApplicationContext(), "Volley error: " + error.getMessage(), Toast.LENGTH_SHORT).show();
}
}) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<>();
params.put("name", name);
params.put("email", email);
Log.e(TAG, "params: " + params.toString());
return params;
}
};
//Adding request to request queue
MyApplication.getInstance().addToRequestQueue(strReq);
}
private void requestFocus(View view) {
if (view.requestFocus()) {
getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_STATE_ALWAYS_VISIBLE);
}
}
// Validating name
private boolean validateName() {
if (inputName.getText().toString().trim().isEmpty()) {
inputLayoutName.setError(getString(R.string.err_msg_name));
requestFocus(inputName);
return false;
} else {
inputLayoutName.setErrorEnabled(false);
}
return true;
}
// Validating email
private boolean validateEmail() {
String email = inputEmail.getText().toString().trim();
if (email.isEmpty() || !isValidEmail(email)) {
inputLayoutEmail.setError(getString(R.string.err_msg_email));
requestFocus(inputEmail);
return false;
} else {
inputLayoutEmail.setErrorEnabled(false);
}
return true;
}
private static boolean isValidEmail(String email) {
return !TextUtils.isEmpty(email) && android.util.Patterns.EMAIL_ADDRESS.matcher(email).matches();
}
private class MyTextWatcher implements TextWatcher {
private View view;
private MyTextWatcher(View view) {
this.view = view;
}
public void beforeTextChanged(CharSequence charSequence, int i, int i1, int i2) {
}
public void onTextChanged(CharSequence charSequence, int i, int i1, int i2) {
}
public void afterTextChanged(Editable editable) {
switch (view.getId()) {
case R.id.input_name:
validateName();
break;
case R.id.input_email:
validateEmail();
break;
}
}
}
}
因为您得到的response
没有正确格式化以进行Json转换。
JSONObject obj = new JSONObject(response);
您尝试将其转换为JSONObject
返回boolean
。
编辑1:在获取boolean
也可能出现问题。
尝试像这样获取它:
jsonObject.optBoolean("error");
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.