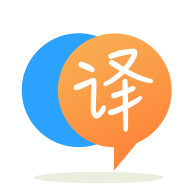
[英]How do I convert string to number and ensure a float type in JavaScript?
[英]How do I convert a float number to a whole number in JavaScript?
我想在 JavaScript 中将浮点数转换为整数。 实际上,我想知道如何进行两种标准转换:截断和舍入。 并且有效,而不是通过转换为字符串和解析。
var intvalue = Math.floor( floatvalue );
var intvalue = Math.ceil( floatvalue );
var intvalue = Math.round( floatvalue );
// `Math.trunc` was added in ECMAScript 6
var intvalue = Math.trunc( floatvalue );
// value=x // x=5 5<x<5.5 5.5<=x<6 Math.floor(value) // 5 5 5 Math.ceil(value) // 5 6 6 Math.round(value) // 5 5 6 Math.trunc(value) // 5 5 5 parseInt(value) // 5 5 5 ~~value // 5 5 5 value | 0 // 5 5 5 value >> 0 // 5 5 5 value >>> 0 // 5 5 5 value - value % 1 // 5 5 5
消极的
// x = Number.MAX_SAFE_INTEGER/10 // =900719925474099.1
// value=x x=900719925474099 x=900719925474099.4 x=900719925474099.5
Math.floor(value) // 900719925474099 900719925474099 900719925474099
Math.ceil(value) // 900719925474099 900719925474100 900719925474100
Math.round(value) // 900719925474099 900719925474099 900719925474100
Math.trunc(value) // 900719925474099 900719925474099 900719925474099
parseInt(value) // 900719925474099 900719925474099 900719925474099
value | 0 // 858993459 858993459 858993459
~~value // 858993459 858993459 858993459
value >> 0 // 858993459 858993459 858993459
value >>> 0 // 858993459 858993459 858993459
value - value % 1 // 900719925474099 900719925474099 900719925474099
正数 - 较大的数字
// x = Number.MAX_SAFE_INTEGER/10 // =900719925474099.1 // value=xx=900719925474099 x=900719925474099.4 x=900719925474099.5 Math.floor(value) // 900719925474099 900719925474099 900719925474099 Math.ceil(value) // 900719925474099 900719925474100 900719925474100 Math.round(value) // 900719925474099 900719925474099 900719925474100 Math.trunc(value) // 900719925474099 900719925474099 900719925474099 parseInt(value) // 900719925474099 900719925474099 900719925474099 value | 0 // 858993459 858993459 858993459 ~~value // 858993459 858993459 858993459 value >> 0 // 858993459 858993459 858993459 value >>> 0 // 858993459 858993459 858993459 value - value % 1 // 900719925474099 900719925474099 900719925474099
负数 - 更大的数字
// x = Number.MAX_SAFE_INTEGER/10 * -1 // -900719925474099.1 // value = x // x=-900719925474099 x=-900719925474099.5 x=-900719925474099.6 Math.floor(value) // -900719925474099 -900719925474100 -900719925474100 Math.ceil(value) // -900719925474099 -900719925474099 -900719925474099 Math.round(value) // -900719925474099 -900719925474099 -900719925474100 Math.trunc(value) // -900719925474099 -900719925474099 -900719925474099 parseInt(value) // -900719925474099 -900719925474099 -900719925474099 value | 0 // -858993459 -858993459 -858993459 ~~value // -858993459 -858993459 -858993459 value >> 0 // -858993459 -858993459 -858993459 value >>> 0 // 3435973837 3435973837 3435973837 value - value % 1 // -900719925474099 -900719925474099 -900719925474099
按位或运算符可用于截断浮点数,它适用于正数和负数:
function float2int (value) {
return value | 0;
}
结果
float2int(3.1) == 3
float2int(-3.1) == -3
float2int(3.9) == 3
float2int(-3.9) == -3
我创建了一个比较性能的JSPerf 测试:
Math.floor(val)
val | 0
val | 0
按位或~~val
按位非parseInt(val)
这只适用于正数。 在这种情况下,您可以安全地使用按位运算以及Math.floor
函数。
但是,如果您需要您的代码同时使用 positives 和 negatives ,那么按位运算是最快的(OR 是首选)。 这个其他 JSPerf 测试比较相同,很明显,由于额外的符号检查, Math 现在是四个中最慢的。
如评论中所述,BITWISE 运算符对有符号的 32 位整数进行操作,因此将转换大数,例如:
1234567890 | 0 => 1234567890
12345678901 | 0 => -539222987
注意:您不能使用Math.floor()
作为 truncate 的替代品,因为Math.floor(-3.1) = -4
而不是-3
!!
截断的正确替换是:
function truncate(value)
{
if (value < 0) {
return Math.ceil(value);
}
return Math.floor(value);
}
双位非运算符可用于截断浮点数。 您提到的其他操作可通过Math.floor
、 Math.ceil
和Math.round
获得。
> ~~2.5
2
> ~~(-1.4)
-1
对于截断:
var intvalue = Math.floor(value);
对于圆形:
var intvalue = Math.round(value);
您可以使用parseInt方法不进行舍入。 由于 0x(十六进制)和 0(八进制)前缀选项,请注意用户输入。
var intValue = parseInt(floatValue, 10);
编辑:作为警告(来自评论部分),请注意某些数值将被转换为它们的指数形式,例如1e21
这会导致"1"
的十进制表示不正确
位移 0 相当于除以 1
// >> or >>>
2.0 >> 0; // 2
2.0 >>> 0; // 2
在您的情况下,当您最后需要一个字符串(以便插入逗号)时,您也可以只使用Number.toFixed()
函数,但是,这将执行舍入。
另一种可能的方法 - 使用 XOR 操作:
console.log(12.3 ^ 0); // 12 console.log("12.3" ^ 0); // 12 console.log(1.2 + 1.3 ^ 0); // 2 console.log(1.2 + 1.3 * 2 ^ 0); // 3 console.log(-1.2 ^ 0); // -1 console.log(-1.2 + 1 ^ 0); // 0 console.log(-1.2 - 1.3 ^ 0); // -2
按位运算的优先级低于数学运算的优先级,它很有用。 试试https://jsfiddle.net/au51uj3r/
截断:
// Math.trunc() is part of the ES6 spec console.log(Math.trunc( 1.5 )); // returns 1 console.log(Math.trunc( -1.5 )); // returns -1 // Math.floor( -1.5 ) would return -2, which is probably not what you wanted
舍入:
console.log(Math.round( 1.5 )); // 2 console.log(Math.round( 1.49 )); // 1 console.log(Math.round( -1.6 )); // -2 console.log(Math.round( -1.3 )); // -1
这里有很多建议。 到目前为止,按位 OR 似乎是最简单的。 这是另一个简短的解决方案,它也适用于负数以及使用模运算符。 它可能比按位 OR 更容易理解:
intval = floatval - floatval%1;
此方法也适用于 '|0' 、 '~~' 和 '>>0' 都不能正常工作的高值数字:
> n=4294967295;
> n|0
-1
> ~~n
-1
> n>>0
-1
> n-n%1
4294967295
//Convert a float to integer Math.floor(5.95) //5 Math.ceil(5.95) //6 Math.round(5.4) //5 Math.round(5.5) //6 Math.trunc(5.5) //5 //Quick Ways console.log(5.95| 0) console.log(~~5.95) console.log(5.95 >> 0) //5
Math.floor()函数返回小于或等于给定数字的最大整数。
console.log('Math.floor : ', Math.floor(3.5)); console.log('Math.floor : ', Math.floor(-3.5));
Math.ceil()函数总是将数字向上舍入到下一个最大整数。
console.log('Math.ceil : ', Math.ceil(3.5)); console.log('Math.ceil : ', Math.ceil(-3.5));
Math.round()函数返回四舍五入到最接近整数的数值。
console.log('Math.round : ', Math.round(3.5)); console.log('Math.round : ', Math.round(-3.5));
Math.trunc()函数通过删除任何小数位返回数字的整数部分。
console.log('Math.trunc : ', Math.trunc(3.5)); console.log('Math.trunc : ', Math.trunc(-3.5));
如果查看 JavaScript 中的原生Math
对象,你会得到一大堆函数来处理数字和值等......
基本上你想要做的是非常简单和原生的 JavaScript ......
假设您有以下数字:
const myValue = 56.4534931;
现在,如果您想将其四舍五入到最接近的数字,只需执行以下操作:
const rounded = Math.floor(myValue);
你得到:
56
如果要将其四舍五入到最接近的数字,只需执行以下操作:
const roundedUp = Math.ceil(myValue);
你得到:
57
此外Math.round
只是将其四舍五入到更高或更低的数字取决于哪个更接近浮点数。
您也可以在浮点数后面使用~~
,这会将浮点数转换为整数。
你可以像~~myValue
...
今天 2020 年 11 月 28 日,我在 Chrome v85、Safari v13.1.2 和 Firefox v80 上对 MacOs HighSierra 10.13.6 进行测试,以选择解决方案。
我执行你可以在这里运行的测试用例
下面的片段显示了解决方案A B C D E F G H I J K L之间的差异
function A(float) { return Math.trunc( float ); } function B(float) { return parseInt(float); } function C(float) { return float | 0; } function D(float) { return ~~float; } function E(float) { return float >> 0; } function F(float) { return float - float%1; } function G(float) { return float ^ 0; } function H(float) { return Math.floor( float ); } function I(float) { return Math.ceil( float ); } function J(float) { return Math.round( float ); } function K(float) { return float.toFixed(0); } function L(float) { return float >>> 0; } // --------- // TEST // --------- [A,B,C,D,E,F,G,H,I,J,K,L] .forEach(f=> console.log(`${f.name} ${f(1.5)} ${f(-1.5)} ${f(2.499)} ${f(-2.499)}`))
This snippet only presents functions used in performance tests - it not perform tests itself!
这是 chrome 的示例结果
如果你想要一个向下的四舍五入答案:
var intvalue = Math.floor( floatvalue );
var integer = Math.floor(4.56);
Answer = 4
如果要向上舍入:
var intvalue = Math.ceil( floatvalue );
Answeer would be = 5
我只想指出,从金钱上讲,您要四舍五入,而不是截断。 减少一分钱的可能性要小得多,因为四舍五入的 4.999452 * 100 会给你 5,这是一个更具代表性的答案。
最重要的是,不要忘记银行家的四舍五入,这是一种抵消直接四舍五入带来的轻微正偏差的方法——您的金融应用程序可能需要它。
如果您使用的是 angularjs,那么简单的解决方案如下在 HTML 模板绑定中
{{val | number:0}}
它会将 val 转换为整数
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.